In C++ code: Design and write a C++ class that reads text, binary and csv files. The class functions: Size: Returns the file size Name: Returns the file name Raw: Returns the unparsed raw data Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse. Call-back.cpp #include #include #include #include using namespace std; string ToLower(string s) { string temp; for (char c : s) temp.push_back(tolower(c)); temp.push_back(NULL); return temp; } string ToUpper(string s) { string temp; for (char c : s) temp.push_back(toupper(c)); temp.push_back(NULL); return temp; } struct Append { string operator()(string a, string b) // function object { return a + b; } }; class CallBack { function callback; public: CallBack(function cb) { callback = cb; } string useCallBack(string s) { return callback(s); } }; void embedd(string s) { CallBack cback(ToUpper); cout << cback.useCallBack(s) << endl; } void functional() { string cat = "cat"; string dog = "DOG"; function fn1 = ToUpper; // ToLower; // function function fn2 = &ToUpper; // function pointer function fn3 = Append(); // function object function fn4 = [](string s) { return s.length(); }; // lambda expression function fn5 = plus(); // standard function object cout << fn1(dog) << endl; cout << fn2(cat) << endl; cout << fn3(cat, dog) << endl; cout << fn4(dog) << endl; cout << fn5(123, 456) << endl; } void main() { functional(); embedd("dog"); }
In C++ code:
Design and write a C++ class that reads text, binary and csv files. The class functions:
Size: Returns the file size
Name: Returns the file name
Raw: Returns the unparsed raw data
Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse.
Call-back.cpp
#include <string>
#include <functional>
#include <iostream>
#include <vector>
using namespace std;
string ToLower(string s)
{
string temp;
for (char c : s)
temp.push_back(tolower(c));
temp.push_back(NULL);
return temp;
}
string ToUpper(string s)
{
string temp;
for (char c : s)
temp.push_back(toupper(c));
temp.push_back(NULL);
return temp;
}
struct Append
{
string operator()(string a, string b) // function object
{
return a + b;
}
};
class CallBack
{
function<string(string)> callback;
public:
CallBack(function<string(string)> cb) { callback = cb; }
string useCallBack(string s) { return callback(s); }
};
void embedd(string s)
{
CallBack cback(ToUpper);
cout << cback.useCallBack(s) << endl;
}
void functional()
{
string cat = "cat";
string dog = "DOG";
function<string(string)> fn1 = ToUpper; // ToLower; // function
function<string(string)> fn2 = &ToUpper; // function pointer
function<string(string, string)> fn3 = Append(); // function object
function<int(string)> fn4 = [](string s) { return s.length(); }; // lambda expression
function<int(int, int)> fn5 = plus<int>(); // standard function object
cout << fn1(dog) << endl;
cout << fn2(cat) << endl;
cout << fn3(cat, dog) << endl;
cout << fn4(dog) << endl;
cout << fn5(123, 456) << endl;
}
void main()
{
functional();
embedd("dog");
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

If we need to put
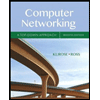
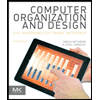
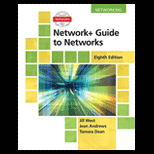
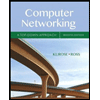
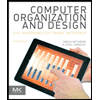
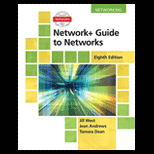
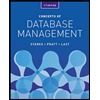
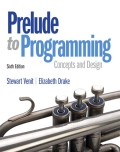
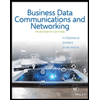