I would like for you to review this code and help me figure out why it isn't displaying the calculated temperatures when I test it. It seems to be an issue with the .js code. index.html Convert Temperatures Convert temperatures Fahrenheit to Celsius Celsius to Fahrenheit Enter F degrees: Degrees Celsius: convert_temp.css body { font-family: Arial, Helvetica, sans-serif; background-color: white; margin: 0 auto; width: 500px; border: 3px solid blue; padding: 0 2em 1em; } h1 { color: blue; } div { margin-bottom: 1em; } label { display: inline-block; width: 11em; text-align: right; } input { margin-left: 1em; margin-right: 0.5em; } span { color: red; } #convert { width: 10em; } /*div that's the 3rd child of its parent element (main) */ div:nth-child(3) { margin-bottom: 1.5em; } convert_temp.js "use strict"; const $ = selector => document.querySelector(selector); /********************* * helper functions * **********************/ const calculateCelsius = temp => (temp-32) * 5/9; const calculateFahrenheit = temp => temp * 9/5 + 32; const toggleDisplay = (label1Text, label2Text) => { document.getElementById("degree_label_1").innerHTML = label1Text; document.getElementById("degree_label_2").innerHTML = label2Text; document.getElementById("degrees_computed").value = ""; } /**************************** * event handler functions * *****************************/ const convertTemp = () => { let degree = document.getElementById("degrees_entered").value; degree = parseFloat(degree); if (isNaN(degree)) { document.getElementById("message").innerHTML = "Please enter a valid degree!."; } else { let result; document.getElementById("message").innerHTML = ""; let check = document.getElementById("to_celsius"); if (check.checked) { result = calculateCelsius(degree); } else { result = calculateFahrenheit(degree); } document.getElementById("degrees_computed").value = Math.round(result); } }; const toCelsius = () => toggleDisplay("Enter F degrees:", "Degrees Celsius:"); const toFahrenheit = () => toggleDisplay("Enter C degrees:", "Degrees Fahrenheit:"); document.addEventListener("DOMContentLoaded", () => { // add event handlers $("#convert").addEventListener("click", convertTemp); $("#to_celsius").addEventListener("click", toCelsius); $("#to_fahrenheit").addEventListener("click", toFahrenheit); // move focus $("#degrees_entered").focus(); });
I would like for you to review this code and help me figure out why it isn't displaying the calculated temperatures when I test it. It seems to be an issue with the .js code. index.html Convert Temperatures Convert temperatures Fahrenheit to Celsius Celsius to Fahrenheit Enter F degrees: Degrees Celsius: convert_temp.css body { font-family: Arial, Helvetica, sans-serif; background-color: white; margin: 0 auto; width: 500px; border: 3px solid blue; padding: 0 2em 1em; } h1 { color: blue; } div { margin-bottom: 1em; } label { display: inline-block; width: 11em; text-align: right; } input { margin-left: 1em; margin-right: 0.5em; } span { color: red; } #convert { width: 10em; } /*div that's the 3rd child of its parent element (main) */ div:nth-child(3) { margin-bottom: 1.5em; } convert_temp.js "use strict"; const $ = selector => document.querySelector(selector); /********************* * helper functions * **********************/ const calculateCelsius = temp => (temp-32) * 5/9; const calculateFahrenheit = temp => temp * 9/5 + 32; const toggleDisplay = (label1Text, label2Text) => { document.getElementById("degree_label_1").innerHTML = label1Text; document.getElementById("degree_label_2").innerHTML = label2Text; document.getElementById("degrees_computed").value = ""; } /**************************** * event handler functions * *****************************/ const convertTemp = () => { let degree = document.getElementById("degrees_entered").value; degree = parseFloat(degree); if (isNaN(degree)) { document.getElementById("message").innerHTML = "Please enter a valid degree!."; } else { let result; document.getElementById("message").innerHTML = ""; let check = document.getElementById("to_celsius"); if (check.checked) { result = calculateCelsius(degree); } else { result = calculateFahrenheit(degree); } document.getElementById("degrees_computed").value = Math.round(result); } }; const toCelsius = () => toggleDisplay("Enter F degrees:", "Degrees Celsius:"); const toFahrenheit = () => toggleDisplay("Enter C degrees:", "Degrees Fahrenheit:"); document.addEventListener("DOMContentLoaded", () => { // add event handlers $("#convert").addEventListener("click", convertTemp); $("#to_celsius").addEventListener("click", toCelsius); $("#to_fahrenheit").addEventListener("click", toFahrenheit); // move focus $("#degrees_entered").focus(); });
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I would like for you to review this code and help me figure out why it isn't displaying the calculated temperatures when I test it. It seems to be an issue with the .js code.
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Convert Temperatures</title>
<link rel="stylesheet" href="convert_temp.css">
</head>
<body>
<main>
<h1>Convert temperatures</h1>
<div>
<input type="radio" name="conversion_type" id="to_celsius" checked>Fahrenheit to Celsius
</div>
<div>
<input type="radio" name="conversion_type" id="to_fahrenheit">Celsius to Fahrenheit
</div>
<div>
<label id="degree_label_1">Enter F degrees:</label>
<input type="text" id="degrees_entered">
</div>
<div>
<label id="degree_label_2">Degrees Celsius:</label>
<input type="text" id="degrees_computed" disabled>
</div>
<div>
<label></label>
<input type="button" id="convert" value="Convert" />
</div>
</main>
<script src="convert_temp.js"></script>
</body>
</html>
convert_temp.css
body {
font-family: Arial, Helvetica, sans-serif;
background-color: white;
margin: 0 auto;
width: 500px;
border: 3px solid blue;
padding: 0 2em 1em;
}
h1 {
color: blue;
}
div {
margin-bottom: 1em;
}
label {
display: inline-block;
width: 11em;
text-align: right;
}
input {
margin-left: 1em;
margin-right: 0.5em;
}
span {
color: red;
}
#convert {
width: 10em;
}
/*div that's the 3rd child of its parent element (main) */
div:nth-child(3) {
margin-bottom: 1.5em;
}
convert_temp.js
"use strict";
const $ = selector => document.querySelector(selector);
/*********************
* helper functions *
**********************/
const calculateCelsius = temp => (temp-32) * 5/9;
const calculateFahrenheit = temp => temp * 9/5 + 32;
const toggleDisplay = (label1Text, label2Text) => {
document.getElementById("degree_label_1").innerHTML = label1Text;
document.getElementById("degree_label_2").innerHTML = label2Text;
document.getElementById("degrees_computed").value = "";
}
/****************************
* event handler functions *
*****************************/
const convertTemp = () => {
let degree = document.getElementById("degrees_entered").value;
degree = parseFloat(degree);
if (isNaN(degree)) {
document.getElementById("message").innerHTML =
"Please enter a valid degree!.";
}
else {
let result;
document.getElementById("message").innerHTML = "";
let check = document.getElementById("to_celsius");
if (check.checked) {
result = calculateCelsius(degree);
} else {
result = calculateFahrenheit(degree);
}
document.getElementById("degrees_computed").value = Math.round(result);
}
};
const toCelsius = () => toggleDisplay("Enter F degrees:", "Degrees Celsius:");
const toFahrenheit = () => toggleDisplay("Enter C degrees:", "Degrees Fahrenheit:");
document.addEventListener("DOMContentLoaded", () => {
// add event handlers
$("#convert").addEventListener("click", convertTemp);
$("#to_celsius").addEventListener("click", toCelsius);
$("#to_fahrenheit").addEventListener("click", toFahrenheit);
// move focus
$("#degrees_entered").focus();
});
Expert Solution

Step 1
Please refer to the following step for the complete solution to the problem above.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
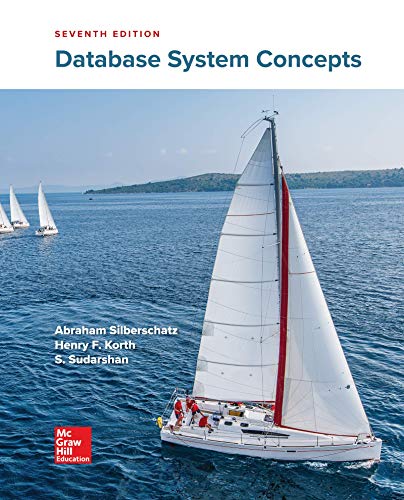
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
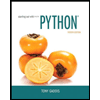
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
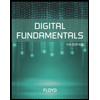
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
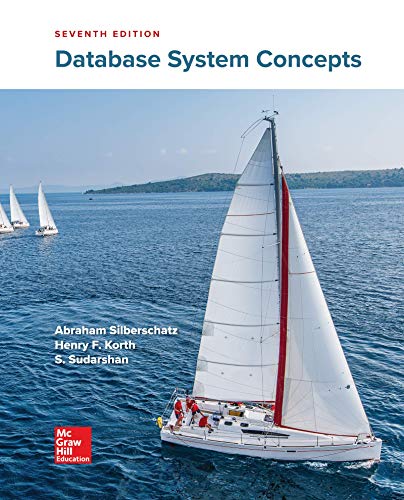
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
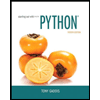
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
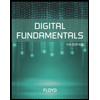
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
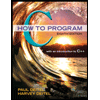
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
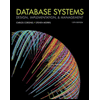
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
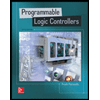
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education