I want this code in C++
I want this code in C++
#include<malloc.h>
//function to fill
int Equalcols(int *A,int rows,int cols)
{
//creating a dynamic array of length cols to store sum of each column
int *b=(int*)(malloc(sizeof(int)*cols));
int i,j;
//initializing the values zero in b array
for(i=0;i<cols;i++)
{
*(b+i)=0;
}
//loop to add elements of each column to respective index in b array
for(i=0;i<rows;i++)
{
for(j=0;j<cols;j++)
{
//adding value of element in respective column to index in b array
*(b+j)+=*(A+j+cols*i);
//printf("%d %d\n",j+cols*i,*(A+j+cols*i));
}
}
//loop to check whether sum is equal or not
for(i=1;i<cols;i++)
{
//printf("%d %d\n",*(b+i-1),*(b+i));
//checking for consecutive value are not equal
if(*(b+i-1)!=*(b+i))
{
//returning 0
return 0;
}
}
//returning 1
return 1;
}
int main()
{
//variables to store no. of rows and columns value
int rows,cols,i,j;
//telling user about the Maximum dimensions
printf("Maximum allowed dimensions of array is 10 X 10\n");
//asking user for no. of rows
printf("Enter no. of rows: ");
//entering user input
scanf("%d",&rows);
//asking user for no. of columns
printf("Enter no. of columns: ");
//entering no. of columns
scanf("%d",&cols);
//2D array creation
int *A=(int*)(malloc(sizeof(int)*rows*cols));
//loop to ask user input for elements in array
for(i=0;i<rows;i++)
{
printf("Enter the elements of %d row:\n",i);
for(j=0;j<cols;j++)
{
//storing value in particular index
scanf("%d",(A+cols*i+j));
}
}
//calling function
int res=Equalcols(A,rows,cols);
if (res)
{
printf("\nSum of elements in columns are equal.");
}
else
{
printf("\nSum of elements in columns are not equal.");
}
return 0;
}

The code has been converted into C++ using the respective libraries that are required to perform the functionality of this code.
Step by step
Solved in 4 steps with 2 images

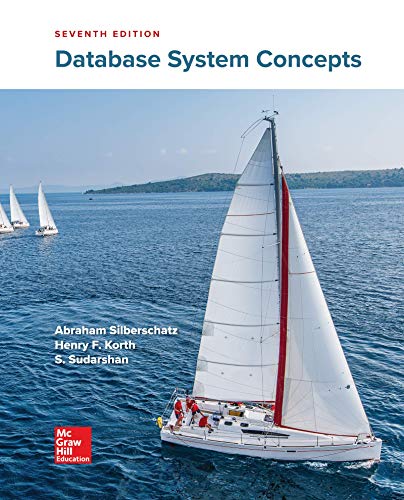
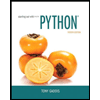
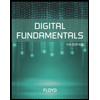
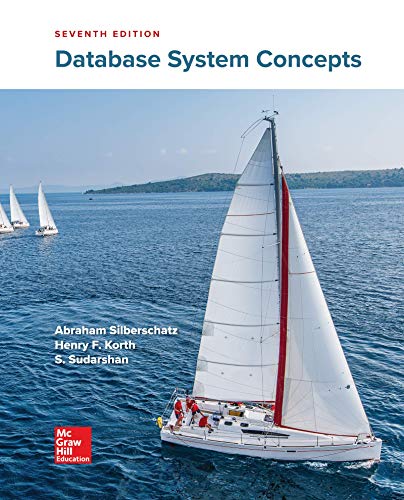
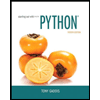
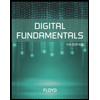
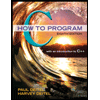
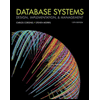
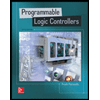