I updated the code for it to start the program but when I click add meal plan it pops up for a second then disspears. How do I fix that? Also all the collection boxes for the listbox appear twice when I run the program. Solutions? Public Class FrmMain Private Sub FrmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load 'Display the Dormitory price in the list box lstDormitories.Items.Add("Allen Hall - 2,500.00 ") lstDormitories.Items.Add("Pike Hall - 2,200.00") lstDormitories.Items.Add("Farthing Hall - 2,100.00") lstDormitories.Items.Add("UniversitySuites - 2,800.00") End Sub Private Sub BtnAddDormitory_Click(sender As Object, e As EventArgs) Handles btnAddDormitory.Click ' if user don't choose any price in list box Select Case lstDormitories.SelectedIndex Case -1 MessageBox.Show("Select A Dormitory Please!") End Select 'Determine the value in Module and display the value of price to lblDormitory Select Case lstDormitories.SelectedIndex Case 0 lblDormitory.Text = mdlSample.Allen_Hall.ToString("c2") Case 1 lblDormitory.Text = mdlSample.Pike_Hall.ToString("c2") Case 2 lblDormitory.Text = mdlSample.Farthing_Hall.ToString("c2") Case 3 lblDormitory.Text = mdlSample.UniversitySuites.ToString("c2") End Select End Sub Private Sub BtnViewMP_Click(sender As Object, e As EventArgs) Handles btnViewMeal.Click 'Display the second form (Meal Plan) Me.Hide() frmMealPlan.Show() End Sub Private Sub BtnClear_Click(sender As Object, e As EventArgs) Handles btnClear.Click 'Clear all the label except the list box lblDormitory.Text = String.Empty lblMealPlan.Text = String.Empty lblTotalCost.Text = String.Empty End Sub Private Sub BtnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click ' Exit Program Application.Exit() End Sub End Class Module mdlSample 'Public constants for Dormitory prices and weedkly meal plan prices Public Const Allen_Hall As Decimal = 2500D Public Const Pike_Hall As Decimal = 2200D Public Const Farthing_Hall As Decimal = 2100D Public Const UniversitySuites As Decimal = 2800D Public Const Meal_Plan_7 As Decimal = 1560D Public Const Meal_Plan_14 As Decimal = 2095D Public Const Meal_Plan_Unilimited As Decimal = 2500D End Module Public Class frmMealPlan Private Sub BtnAdd_MP_Click(sender As Object, e As EventArgs) Handles btnAddMealPlan.Click 'Declare the variable Dim MealPlans As Integer ' if user doesn't choose any Meal Plan then display message box Select Case lstMealPlans.SelectedIndex Case -1 MessageBox.Show("Select A Meal Plan Please!") End Select ' Determine the value in Module and display the value of price to lblMeal_Plan in form Main Select Case lstMealPlans.SelectedIndex Case 0 FrmMain.lblMealPlan.Text = mdlSample.Meal_Plan_7.ToString("c2") MealPlans = mdlSample.Meal_Plan_7.ToString("c2") Case 1 FrmMain.lblMealPlan.Text = mdlSample.Meal_Plan_14.ToString("c2") MealPlans = mdlSample.Meal_Plan_14.ToString("c2") Case 2 FrmMain.lblMealPlan.Text = mdlSample.Meal_Plan_Unilimited.ToString("c2") MealPlans = mdlSample.Meal_Plan_Unilimited.ToString("c2") End Select 'Calculate the total of Dormitory and Meal Plan and display it to form Main FrmMain.lblTotalCost.Text = (FrmMain.lblDormitory.Text + MealPlans).ToString("c2") 'Display to the form Main (frmMain) Me.Hide() FrmMain.Show() End Sub Private Sub FrmMealPlans_Load(sender As Object, e As EventArgs) Handles MyBase.Load 'Display the Weedkly Meal Plan price in the list box lstMealPlans.Items.Add("7 Meals - 1,560.00 ") lstMealPlans.Items.Add("14 Meals - 2,095.00") lstMealPlans.Items.Add("Unlimited Meals - 2,500.00") End Sub Private Sub BtncClose_Click(sender As Object, e As EventArgs) Handles btnClose.Click ' Close program Me.Close() End Sub End Class
I updated the code for it to start the program but when I click add meal plan it pops up for a second then disspears. How do I fix that? Also all the collection boxes for the listbox appear twice when I run the program. Solutions?
Public Class FrmMain
Private Sub FrmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
'Display the Dormitory price in the list box
lstDormitories.Items.Add("Allen Hall - 2,500.00 ")
lstDormitories.Items.Add("Pike Hall - 2,200.00")
lstDormitories.Items.Add("Farthing Hall - 2,100.00")
lstDormitories.Items.Add("UniversitySuites - 2,800.00")
End Sub
Private Sub BtnAddDormitory_Click(sender As Object, e As EventArgs) Handles btnAddDormitory.Click
' if user don't choose any price in list box
Select Case lstDormitories.SelectedIndex
Case -1
MessageBox.Show("Select A Dormitory Please!")
End Select
'Determine the value in Module and display the value of price to lblDormitory
Select Case lstDormitories.SelectedIndex
Case 0
lblDormitory.Text = mdlSample.Allen_Hall.ToString("c2")
Case 1
lblDormitory.Text = mdlSample.Pike_Hall.ToString("c2")
Case 2
lblDormitory.Text = mdlSample.Farthing_Hall.ToString("c2")
Case 3
lblDormitory.Text = mdlSample.UniversitySuites.ToString("c2")
End Select
End Sub
Private Sub BtnViewMP_Click(sender As Object, e As EventArgs) Handles btnViewMeal.Click
'Display the second form (Meal Plan)
Me.Hide()
frmMealPlan.Show()
End Sub
Private Sub BtnClear_Click(sender As Object, e As EventArgs) Handles btnClear.Click
'Clear all the label except the list box
lblDormitory.Text = String.Empty
lblMealPlan.Text = String.Empty
lblTotalCost.Text = String.Empty
End Sub
Private Sub BtnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
' Exit Program
Application.Exit()
End Sub
End Class
Module mdlSample
'Public constants for Dormitory prices and weedkly meal plan prices
Public Const Allen_Hall As Decimal = 2500D
Public Const Pike_Hall As Decimal = 2200D
Public Const Farthing_Hall As Decimal = 2100D
Public Const UniversitySuites As Decimal = 2800D
Public Const Meal_Plan_7 As Decimal = 1560D
Public Const Meal_Plan_14 As Decimal = 2095D
Public Const Meal_Plan_Unilimited As Decimal = 2500D
End Module
Public Class frmMealPlan
Private Sub BtnAdd_MP_Click(sender As Object, e As EventArgs) Handles btnAddMealPlan.Click
'Declare the variable
Dim MealPlans As Integer
' if user doesn't choose any Meal Plan then display message box
Select Case lstMealPlans.SelectedIndex
Case -1
MessageBox.Show("Select A Meal Plan Please!")
End Select
' Determine the value in Module and display the value of price to lblMeal_Plan in form Main
Select Case lstMealPlans.SelectedIndex
Case 0
FrmMain.lblMealPlan.Text = mdlSample.Meal_Plan_7.ToString("c2")
MealPlans = mdlSample.Meal_Plan_7.ToString("c2")
Case 1
FrmMain.lblMealPlan.Text = mdlSample.Meal_Plan_14.ToString("c2")
MealPlans = mdlSample.Meal_Plan_14.ToString("c2")
Case 2
FrmMain.lblMealPlan.Text = mdlSample.Meal_Plan_Unilimited.ToString("c2")
MealPlans = mdlSample.Meal_Plan_Unilimited.ToString("c2")
End Select
'Calculate the total of Dormitory and Meal Plan and display it to form Main
FrmMain.lblTotalCost.Text = (FrmMain.lblDormitory.Text + MealPlans).ToString("c2")
'Display to the form Main (frmMain)
Me.Hide()
FrmMain.Show()
End Sub
Private Sub FrmMealPlans_Load(sender As Object, e As EventArgs) Handles MyBase.Load
'Display the Weedkly Meal Plan price in the list box
lstMealPlans.Items.Add("7 Meals - 1,560.00 ")
lstMealPlans.Items.Add("14 Meals - 2,095.00")
lstMealPlans.Items.Add("Unlimited Meals - 2,500.00")
End Sub
Private Sub BtncClose_Click(sender As Object, e As EventArgs) Handles btnClose.Click
' Close program
Me.Close()
End Sub
End Class

It looks like the issue may be related to the order in which the forms are being shown and hidden. When you click the "Add Meal Plan" button, the current form (frmMealPlan) is being hidden and the main form (FrmMain) is being shown. However, if you immediately close the main form, the entire application will close, which may be why the "Add Meal Plan" form disappears quickly.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

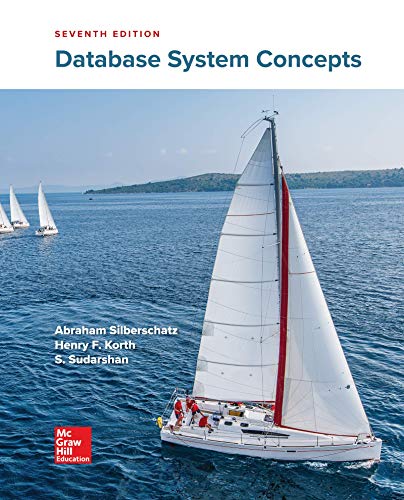
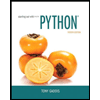
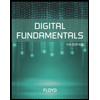
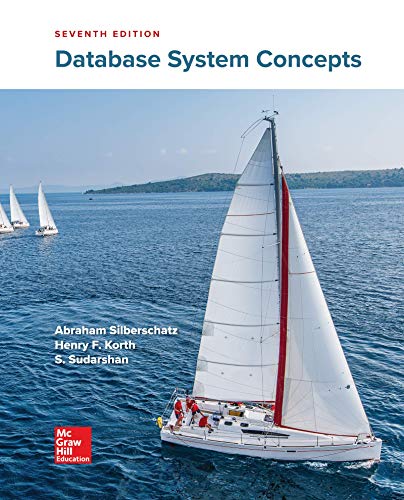
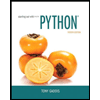
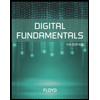
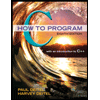
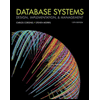
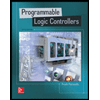