I put in my while loop, and now it has caused my col, num, and tol variables to come out as errors and I don't know why. public static void main(String[] args) { int min = 19; int num1=0, num2=0, num3=0; int tol1=0, tol2=0, tol3=0; int max = 50; int i = 0; while (i < 49) { int col[]=new int[49]; i++; } int ran = (int)(Math.random() * (max - min + 1) + min); if( ran <= 20 && ran < 30){ num1 += 1; tol1 += ran; } if(ran <= 30 && ran < 40){ num2 += 1; tol2 += ran; } if(ran <= 40 && ran < 50){ num3 += 1; tol3 += ran; } int col(i)= ran; } { for(int i = 0; i < 49; i++ ){ if(i == 7 || i == 14 || i == 21 || i == 28 || i == 35 ||i == 42){ System.out.println("\n"); } System.out.printf(("%7d"),+ col[i]); } System.out.printf("\n"); System.out.printf("Found "+ num1 +" number(s) in the 20s "+ "totaling "+ tol1); System.out.printf("\nFound "+ num2 +" number(s) in the 30s "+ "totaling "+ tol2); System.out.printf("\nFound "+ num3 +" number(s) in the 40s "+ "totaling "+ tol3); } }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
I put in my while loop, and now it has caused my col, num, and tol variables to come out as errors and I don't know why.
public static void main(String[] args) {
int min = 19;
int num1=0, num2=0, num3=0;
int tol1=0, tol2=0, tol3=0;
int max = 50;
int i = 0;
while (i < 49) {
int col[]=new int[49];
i++;
}
int ran = (int)(Math.random() * (max - min + 1) + min);
if( ran <= 20 && ran < 30){
num1 += 1;
tol1 += ran;
}
if(ran <= 30 && ran < 40){
num2 += 1; tol2 += ran;
}
if(ran <= 40 && ran < 50){
num3 += 1; tol3 += ran;
}
int col(i)= ran;
}
{
for(int i = 0; i < 49; i++ ){
if(i == 7 || i == 14 || i == 21 || i == 28 || i == 35 ||i == 42){
System.out.println("\n");
}
System.out.printf(("%7d"),+ col[i]);
}
System.out.printf("\n");
System.out.printf("Found "+ num1 +" number(s) in the 20s "+ "totaling "+ tol1);
System.out.printf("\nFound "+ num2 +" number(s) in the 30s "+ "totaling "+ tol2);
System.out.printf("\nFound "+ num3 +" number(s) in the 40s "+ "totaling "+ tol3);
}
}

Step by step
Solved in 2 steps with 1 images

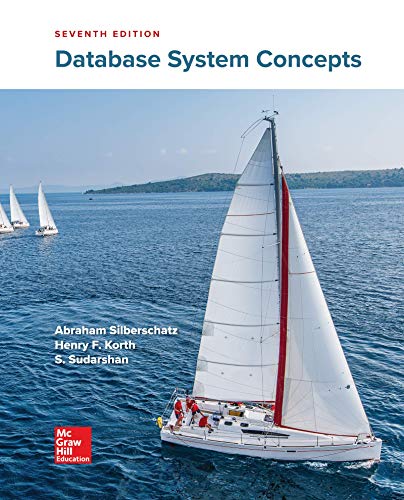
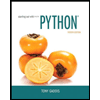
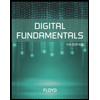
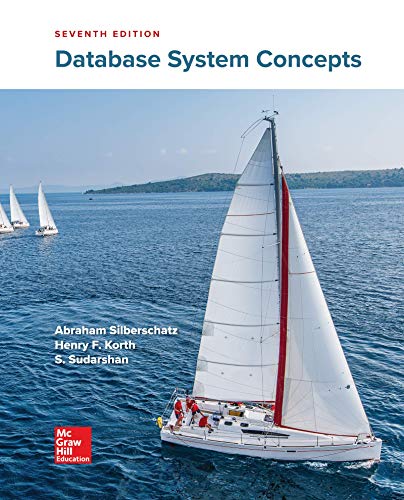
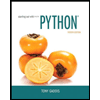
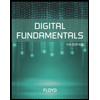
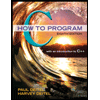
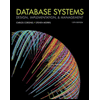
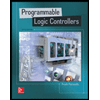