I need the functions to be defined using Haskell language. med3 a b c returns the median value of the set {a,b,c}. med3 :: Integer -> Integer -> Integer -> Integer med3 a b c = undefined -- ghci> med3 1 8 17 -- 8 -- ghci> med3 25 (-1) 6 -- 6 -- ghci> med3 2 11 2 -- 2 blur produces a list containing the means of each pair of numbers in a list. That is, the average of the first and second, the average of the second and third, the average of the third and fourth, and so forth. Note: It returns an empty list if the input has fewer than two elements. blur :: [Double] -> [Double] blur l = undefined -- ghci> blur [1, 3, 5, 7] -- [2.0,4.0,6.0] -- ghci> blur [7, 8, 2.5, 16, 45, 45, 0] -- [7.5,5.25,9.25,30.5,45.0,22.5] -- ghci> blur [] -- [] -- ghci> blur [1] -- [] Note: As this function uses Double, use / rather than div for division.
I need the functions to be defined using Haskell language.
med3 a b c returns the median value of the set {a,b,c}.
med3 :: Integer -> Integer -> Integer -> Integer
med3 a b c = undefined
-- ghci> med3 1 8 17
-- 8
-- ghci> med3 25 (-1) 6
-- 6
-- ghci> med3 2 11 2
-- 2
blur :: [Double] -> [Double]
blur l = undefined
-- [2.0,4.0,6.0]
-- ghci> blur [7, 8, 2.5, 16, 45, 45, 0]
-- [7.5,5.25,9.25,30.5,45.0,22.5]
-- ghci> blur []
-- []
-- ghci> blur [1]
-- []

`med3` Function Algorithm:
1. Define a function called `med3` that takes three Integer arguments: `a`, `b`, and `c`.
2. In the function, use pattern matching and guards to handle different cases based on the values of `a`, `b`, and `c`.
3. Check which of the three values, `a`, `b`, or `c`, is the median.
- If `b` is the median, return `b`.
- If `a` is the median, return `a`.
- If `c` is the median, return `c`.
`blur` Function Algorithm:
1. Define a function called `blur` that takes a list of Doubles, denoted as `l`.
2. Use pattern matching to define different cases for the input list:
- If the input list `l` is empty (`[]`), return an empty list (`[]`) since there are no elements to blur.
- If the input list `l` has only one element (i.e., `[x]`), return an empty list (`[]`) because there are not enough elements to form pairs for blurring.
- For the general case, where `l` has at least two elements:
- Deconstruct the list into the first element `x` and the second element `y`, and the rest of the list (`rest`).
- Calculate the average of `x` and `y` by adding them together and dividing by 2: `(x + y) / 2`.
- Prepend the calculated average to the result of recursively calling the `blur` function on the rest of the list `rest`.
3. Continue this process recursively until the list is reduced to one or zero elements, appending averages of adjacent pairs along the way.
4. The function returns a list of averages.
Step by step
Solved in 4 steps with 1 images

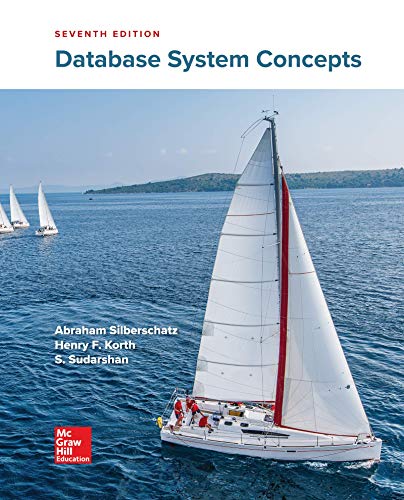
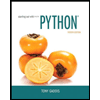
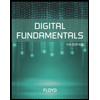
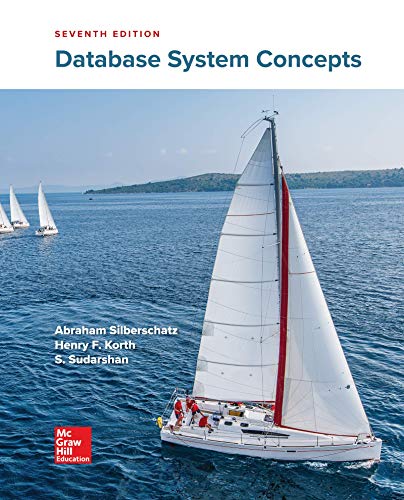
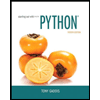
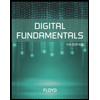
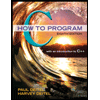
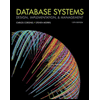
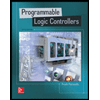