I need help with this lab. It’s one. 13. One lab introduction to cryptography ( classes/ constructors)  This is the current code that must stay within the program and cannot be changed, only amended: class CipherTest: # TODO: Define a constructor with parameters to #initialize instance attributes (shift = 0, direction = 'r', text = "Testing" ) #set the default values of the parameters and also set the #these values as pf the user passed def __init__(self, shift=0, direction='r', text="Testing"): self.shift = shift self.direction = direction self.text = text #Shift to right function def shift_to_right(self): encrypted_Text = "" for i in range(len(self.text)): c = self.text[i] #Encrypt upper case if(c == ' '): encrypted_Text += ' ' elif (c.isupper()): encrypted_Text += chr((ord(c) + self.shift-65) % 26 + 65) #Encrypt lower case else: encrypted_Text += chr((ord(c) + self.shift-97) % 26 + 97) return encrypted_Text #Shift to left function def shift_to_left(self): encrypted_Text = "" for i in range(len(self.text)): c = self.text[i] #Encrypt upper case if(c == ' '): encrypted_Text += ' ' elif (c.isupper()): encrypted_Text += chr((ord(c) - self.shift-65) % 26 + 65) #Encrypt lower case else: encrypted_Text += chr((ord(c) - self.shift-97) % 26 + 97) return encrypted_Text if __name__ == "__main__": # TODO: create input for text, shift value, and direction (use lower( ) to keep l and r lower case #read the input for each variable and store it into the vairables text = input("Enter the text: ") shift = int(input("Enter the shift value: ")) #note, the input was turned to lower case direction = input("Enter the direction (r or l): ").lower() # TODO: create a cipher item and use the constructor with the above input values #create the object of the class and pass all the inputs to its constructor CipherTestObj = CipherTest(shift, direction, text) # TODO: use control structures to call shift_to_right() if direction is right and call shift_to_left if direction # is left. Make sure you print out the return encrypted message here. #variable to store the encrypted text encryptedText = "" #based on the direction entered by the user, call the appropriate functions if CipherTestObj.direction == 'r': encryptedText = CipherTestObj.shift_to_right() elif CipherTestObj.direction == 'l': encryptedText = CipherTestObj.shift_to_left() #if the direction is not valid, print the error message else: print("Invalid direction was found!!") #print the final returned Encrypted Text print("Encrypted text:", encryptedText) This is the copy of the current lab, and the error message on line 51 that I am having a hard time with. Thank you for your help.
I need help with this lab. It’s one. 13. One lab introduction to cryptography ( classes/ constructors)  This is the current code that must stay within the program and cannot be changed, only amended: class CipherTest: # TODO: Define a constructor with parameters to #initialize instance attributes (shift = 0, direction = 'r', text = "Testing" ) #set the default values of the parameters and also set the #these values as pf the user passed def __init__(self, shift=0, direction='r', text="Testing"): self.shift = shift self.direction = direction self.text = text #Shift to right function def shift_to_right(self): encrypted_Text = "" for i in range(len(self.text)): c = self.text[i] #Encrypt upper case if(c == ' '): encrypted_Text += ' ' elif (c.isupper()): encrypted_Text += chr((ord(c) + self.shift-65) % 26 + 65) #Encrypt lower case else: encrypted_Text += chr((ord(c) + self.shift-97) % 26 + 97) return encrypted_Text #Shift to left function def shift_to_left(self): encrypted_Text = "" for i in range(len(self.text)): c = self.text[i] #Encrypt upper case if(c == ' '): encrypted_Text += ' ' elif (c.isupper()): encrypted_Text += chr((ord(c) - self.shift-65) % 26 + 65) #Encrypt lower case else: encrypted_Text += chr((ord(c) - self.shift-97) % 26 + 97) return encrypted_Text if __name__ == "__main__": # TODO: create input for text, shift value, and direction (use lower( ) to keep l and r lower case #read the input for each variable and store it into the vairables text = input("Enter the text: ") shift = int(input("Enter the shift value: ")) #note, the input was turned to lower case direction = input("Enter the direction (r or l): ").lower() # TODO: create a cipher item and use the constructor with the above input values #create the object of the class and pass all the inputs to its constructor CipherTestObj = CipherTest(shift, direction, text) # TODO: use control structures to call shift_to_right() if direction is right and call shift_to_left if direction # is left. Make sure you print out the return encrypted message here. #variable to store the encrypted text encryptedText = "" #based on the direction entered by the user, call the appropriate functions if CipherTestObj.direction == 'r': encryptedText = CipherTestObj.shift_to_right() elif CipherTestObj.direction == 'l': encryptedText = CipherTestObj.shift_to_left() #if the direction is not valid, print the error message else: print("Invalid direction was found!!") #print the final returned Encrypted Text print("Encrypted text:", encryptedText) This is the copy of the current lab, and the error message on line 51 that I am having a hard time with. Thank you for your help.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I need help with this lab. It’s one. 13. One lab introduction to cryptography ( classes/ constructors)
 This is the current code that must stay within the program and cannot be changed, only amended:
class CipherTest:
# TODO: Define a constructor with parameters to
#initialize instance attributes (shift = 0, direction = 'r', text = "Testing" )
#set the default values of the parameters and also set the
#these values as pf the user passed
def __init__(self, shift=0, direction='r', text="Testing"):
self.shift = shift
self.direction = direction
self.text = text
#Shift to right function
def shift_to_right(self):
encrypted_Text = ""
for i in range(len(self.text)):
c = self.text[i]
#Encrypt upper case
if(c == ' '):
encrypted_Text += ' '
elif (c.isupper()):
encrypted_Text += chr((ord(c) + self.shift-65) % 26 + 65)
#Encrypt lower case
else:
encrypted_Text += chr((ord(c) + self.shift-97) % 26 + 97)
return encrypted_Text
#Shift to left function
def shift_to_left(self):
encrypted_Text = ""
for i in range(len(self.text)):
c = self.text[i]
#Encrypt upper case
if(c == ' '):
encrypted_Text += ' '
elif (c.isupper()):
encrypted_Text += chr((ord(c) - self.shift-65) % 26 + 65)
#Encrypt lower case
else:
encrypted_Text += chr((ord(c) - self.shift-97) % 26 + 97)
return encrypted_Text
if __name__ == "__main__":
# TODO: create input for text, shift value, and direction (use lower( ) to keep l and r lower case
#read the input for each variable and store it into the vairables
text = input("Enter the text: ")
shift = int(input("Enter the shift value: "))
#note, the input was turned to lower case
direction = input("Enter the direction (r or l): ").lower()
# TODO: create a cipher item and use the constructor with the above input values
#create the object of the class and pass all the inputs to its constructor
CipherTestObj = CipherTest(shift, direction, text)
# TODO: use control structures to call shift_to_right() if direction is right and call shift_to_left if direction
# is left. Make sure you print out the return encrypted message here.
#variable to store the encrypted text
encryptedText = ""
#based on the direction entered by the user, call the appropriate functions
if CipherTestObj.direction == 'r':
encryptedText = CipherTestObj.shift_to_right()
elif CipherTestObj.direction == 'l':
encryptedText = CipherTestObj.shift_to_left()
#if the direction is not valid, print the error message
else:
print("Invalid direction was found!!")
#print the final returned Encrypted Text
print("Encrypted text:", encryptedText)
This is the copy of the current lab, and the error message on line 51 that I am having a hard time with.
Thank you for your help.

Transcribed Image Text:ry > CYB 135: Object-Oriented Scripting Language II home > 1.13: LAB: Introduction to Cryptography (classes/constructors)
46 if name
==
main":
47
# TODO: create input for text, shift value, and direction (use Lower() to keep L and r Lower case
to search
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
53
#read the input for each variable and store it into the vairables
text input ("Enter the text: ")
=
shift = int(input ("Enter the shift value: "))
#note, the input was turned to Lower case
direction = input ("Enter the direction (r or 1): ").lower()
# TODO: create a cipher item and use the constructor with the above input values
#create the object of the class and pass all the inputs to its constructor
Cipher TestObj = CipherTest (shift, direction, text)
# TODO: use control structures to call shift_to_right() if direction is right and call shift_to_left if directio
# is left. Make sure you print out the return encrypted message here.
Develop mode
Submit mode
Enter program input (optional)
Cryptography is fun!
Run program
Run your program as often as you'd like, before submitting for grading. Below, type any needed
input values in the first box, then click Run program and observe the program's output in the
second box.
Input (from above) →
Program errors displayed here
Traceback (most recent call last):
File "main.py", line 51, in <module>
shift int (input ("Enter the shift value: "))
EOFError: EOF when reading a line
Program output displayed here
Enter the text: Enter the shift value:
W
main.py
(Your program)
→→→→Output (shown below)
EzyBooks ca

Transcribed Image Text:1.13 LAB: Introduction to Cryptography (classes/constructors)
Cryptography is the practice of encryption. Information Security uses cryptography techniques to encrypt and decrypt data. A simple
encryption method might take plaintext and mix up the letters using some predetermined pattern and then use that pattern to decrypt the
data for reading.
Ciphers are the algorithms used to put the data into its secret pattern and then systematically decrypt it for reading. This script is going to
use a famous simple cipher called the Caesar Cipher. It is a substitution cipher where each letter in the text is 'shifted' in a certain number
of places. It uses the alphabet as the primary pattern and then based on the shift number, it would shift all the letters and replace the
alphabet with our pattern.
For example, if our shift number was 3, then A would be replaced with D, if we performed a right shift. As an example:
Text = "THE CAT IS VISIBLE AT MIDNIGHT" Ciphertext = "WKH FDW LV YLVLEOH DW PLGQLIJKW"
The keys to decrypt this message are the shift number and the direction. The shift value can be any integer from 0-25. The above example
uses shift = 3 and the shift direction is right or direction = 'r'.
Complete the CipherTest class by adding a constructor to initialize a cipher item. The constructor should initialize the shift to 0, and the
direction to 'r' for right shift. If the constructor is call with a shift lue, and direction, the constructor should assign each instance
attribute with the appropriate parameter value.
Complete the following TODO's: (1) create input for text, shift value, and direction (use lower()) to keep I and r lower case (2) create a cipher
item and use the constructor with the above input values (3) use control structures to call shifttoright() if direction is right and call shifttoleft
if direction is left. Make sure you print out the return encrypted message inside the control structures.
We can create the encrypted text by using the ord () function. This function will return an integer that represents the Unicode code point of
the character. Character are represented by different values for upp/er and lower case so an 'a' returns the integer 97. By using the unicode
value we can add and subtract our shift value represented by an integer.
The given program accepts as input a text string as our message to be encrypted, a shift value, and a direction of 'I' for left and 'r' for right.
The program creates a cipher item using the input values. The program outputs the encrypted message based on the shift value and the
direction provided.
Ex: If the input is text = "Cryptography is fun!", shift = 4, and direction = 1.
The output is:
Ynulpkcnwldu eo bajk
442626 1727602.qxaray7
LAB
1.13.1: LAB: Introduction to Cryptography (classes/constructors)
0/10
8
AC
GO
Rai
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
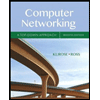
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
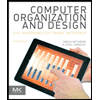
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
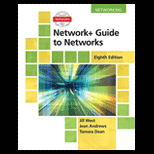
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
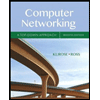
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
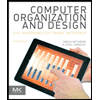
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
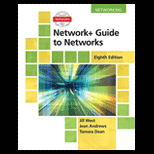
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
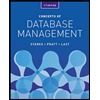
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
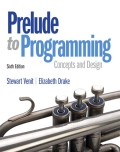
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
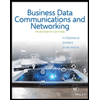
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY