I need help with this Java problem as it's explained in the image below: Image attached can not edit only the code below: public class ShoppingList{ public void add(Item item){ // *** Student task #1 *** /* Requirements: 1. if null item is not allowed: do nothing if null 2. if the list is full, double the array size-dynamic array allocation 3. No duplicated items-all items' names must be unique. If an item with the same name already exists in the list, simply add quantity to the existing item in the list 4. insert the item to the array to maintain sorted-items are sorted based on item names. *** Enter your code below *** */ } public void remove(Item item){//remove all items with the same name. // *** Student task #2 *** /* Requirements: 1. If item not found(item name), display the item does not exists in the list otherwise, remove the item from the list. 2. Hint: You do need to shift all items after removed one. *** Enter your code below *** */ } public void remove(String name){ // remove item by its name // *** Student task #3 *** /* Requirements: 1. If item not found(item name), display the item does not exists in the list otherwise, remove the item from the list. 2. Hint: You do need to shift all items after removed one. *** Enter your code below *** */ } //*****************DONOT modify codes below this line**************************** private Item[] list; private int numberOfItems; public ShoppingList(int initialSize){ list=new Item[initialSize]; } private void doubling(){ //Double the list size but keep all items in the list. Item[] tmp=new Item[list.length*2]; for(int i=0; i
I need help with this Java problem as it's explained in the image below: Image attached can not edit only the code below: public class ShoppingList{ public void add(Item item){ // *** Student task #1 *** /* Requirements: 1. if null item is not allowed: do nothing if null 2. if the list is full, double the array size-dynamic array allocation 3. No duplicated items-all items' names must be unique. If an item with the same name already exists in the list, simply add quantity to the existing item in the list 4. insert the item to the array to maintain sorted-items are sorted based on item names. *** Enter your code below *** */ } public void remove(Item item){//remove all items with the same name. // *** Student task #2 *** /* Requirements: 1. If item not found(item name), display the item does not exists in the list otherwise, remove the item from the list. 2. Hint: You do need to shift all items after removed one. *** Enter your code below *** */ } public void remove(String name){ // remove item by its name // *** Student task #3 *** /* Requirements: 1. If item not found(item name), display the item does not exists in the list otherwise, remove the item from the list. 2. Hint: You do need to shift all items after removed one. *** Enter your code below *** */ } //*****************DONOT modify codes below this line**************************** private Item[] list; private int numberOfItems; public ShoppingList(int initialSize){ list=new Item[initialSize]; } private void doubling(){ //Double the list size but keep all items in the list. Item[] tmp=new Item[list.length*2]; for(int i=0; i
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help with this Java problem as it's explained in the image below:
Image attached can not edit only the code below:
public class ShoppingList{
public void add(Item item){
// *** Student task #1 ***
/*
Requirements:
1. if null item is not allowed: do nothing if null
2. if the list is full, double the array size-dynamic array allocation
3. No duplicated items-all items' names must be unique. If an item with the same name
already exists in the list, simply add quantity to the existing item in the list
4. insert the item to the array to maintain sorted-items are sorted based on item names.
*** Enter your code below ***
*/
}
public void remove(Item item){//remove all items with the same name.
// *** Student task #2 ***
/*
Requirements:
1. If item not found(item name), display the item does not exists in the list
otherwise, remove the item from the list.
2. Hint: You do need to shift all items after removed one.
*** Enter your code below ***
*/
}
public void remove(String name){ // remove item by its name
// *** Student task #3 ***
/*
Requirements:
1. If item not found(item name), display the item does not exists in the list
otherwise, remove the item from the list.
2. Hint: You do need to shift all items after removed one.
*** Enter your code below ***
*/
}
//*****************DONOT modify codes below this line****************************
private Item[] list;
private int numberOfItems;
public ShoppingList(int initialSize){
list=new Item[initialSize];
}
private void doubling(){
//Double the list size but keep all items in the list.
Item[] tmp=new Item[list.length*2];
for(int i=0; i<list.length; i++){
tmp[i]=list[i];
}
list=tmp;
}
public int indexOf(Item item){
// return the index of the item that has the same item name in the list array, return -1 if not found
for(int i=0; i<numberOfItems; i++){
if(list[i].compareTo(item)==0)
return i;
}
return -1; // not found
}
public int indexOf(String name){
// return the index of the item that has the same item name in the list array, return -1 if not found
name=name.toLowerCase();
for(int i=0; i<numberOfItems; i++){
if(list[i].getName().toLowerCase().compareTo(name)==0)
return i;
}
return -1; // not found
}
public boolean isFull(){
return numberOfItems==list.length;
}
public boolean isEmpty(){
return numberOfItems==0;
}
public int size(){
return numberOfItems;
}
public void printNames(){
System.out.print("[");
for(int i=0; i<numberOfItems; i++){
System.out.print(list[i].getName());
if(i<numberOfItems-1)
System.out.print(", ");
}
System.out.println("]");
}
public void print(){
for(int i=0; i<numberOfItems; i++){
System.out.println((i+1)+". "+list[i].toString());
}
}
public void printItems(){
for(int i=0; i<numberOfItems; i++){
System.out.printf("%3s%-16s%3d %.2f\n",""+(i+1)+". ",list[i].getName(),list[i].getQuantity(), list[i].getUnitPrice());
}
}
public Item[] getList(){
return list;
}
}
![***
*Item.java: Item class represents grocery items to be added to the shopping list
*1
public class Item implements Comparable<Item>{
private String name; //product name
private int quantity; //quantity
private double unitPrice; //unit price
public Item(String name, int quantity, double unitPrice){
}
setName(name);
setQuantity(quantity);
setUnitPrice(unitPrice);
public String getName(){
return name;
}
public int getQuantity(){
}
return quantity;
public double getUnitPrice(){
}
return unitPrice;
public void setName(String name){
// The length of product name is limited to [1,50], if more than 50 characters, use the first 50 chars
// must neigher be a null value nor an empty String (throw runtime exception)
if(name == null || name.length()==0){
}
throw new RuntimeException("null or empty String value is not allowed");
if(name.length()>50){
name=name.substring(0, 50);
}
this.name = name;
}
public void setQuantity(int quantity){
//quantity is limited to [1, 100], assign 1 for all invalid numbers
if(quantity<1 || quantity>100){
}
}
quantity=1;
this.quantity = quantity;
public void setUnitPrice(double unitPrice){
//unitPrice is limited to (0, 1000], assign 0.99 for all invalid numbers
if(unitPrice<=0 || unitPrice>1000){
unitPrice=0.99;
}
}
this.unitPrice = unitPrice;
public int compareTo(Item item){
}
}
//compare two items based on their names, ignore cases
return name.toLowerCase().compareTo(item.getName().toLowerCase());
public double subtotal(){
return quantity*unitPrice;
public String toString(){
}}
return String.format("%-50%-5d$%.2f", name,quantity, quantity*unitPrice);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e135d5c-f867-4afd-9f0d-b505f4f19664%2F2f892c26-8911-4507-a12e-a82d5057b36c%2Fb7r4tug_processed.png&w=3840&q=75)
Transcribed Image Text:***
*Item.java: Item class represents grocery items to be added to the shopping list
*1
public class Item implements Comparable<Item>{
private String name; //product name
private int quantity; //quantity
private double unitPrice; //unit price
public Item(String name, int quantity, double unitPrice){
}
setName(name);
setQuantity(quantity);
setUnitPrice(unitPrice);
public String getName(){
return name;
}
public int getQuantity(){
}
return quantity;
public double getUnitPrice(){
}
return unitPrice;
public void setName(String name){
// The length of product name is limited to [1,50], if more than 50 characters, use the first 50 chars
// must neigher be a null value nor an empty String (throw runtime exception)
if(name == null || name.length()==0){
}
throw new RuntimeException("null or empty String value is not allowed");
if(name.length()>50){
name=name.substring(0, 50);
}
this.name = name;
}
public void setQuantity(int quantity){
//quantity is limited to [1, 100], assign 1 for all invalid numbers
if(quantity<1 || quantity>100){
}
}
quantity=1;
this.quantity = quantity;
public void setUnitPrice(double unitPrice){
//unitPrice is limited to (0, 1000], assign 0.99 for all invalid numbers
if(unitPrice<=0 || unitPrice>1000){
unitPrice=0.99;
}
}
this.unitPrice = unitPrice;
public int compareTo(Item item){
}
}
//compare two items based on their names, ignore cases
return name.toLowerCase().compareTo(item.getName().toLowerCase());
public double subtotal(){
return quantity*unitPrice;
public String toString(){
}}
return String.format("%-50%-5d$%.2f", name,quantity, quantity*unitPrice);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
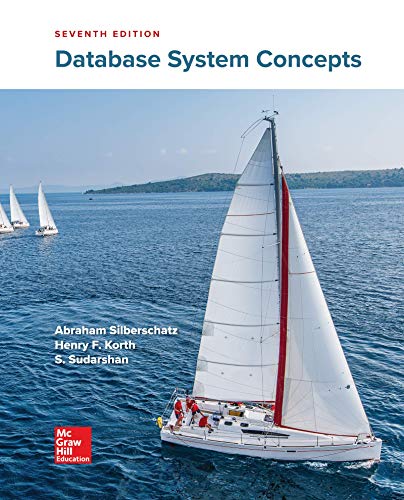
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
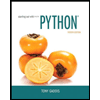
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
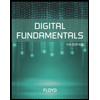
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
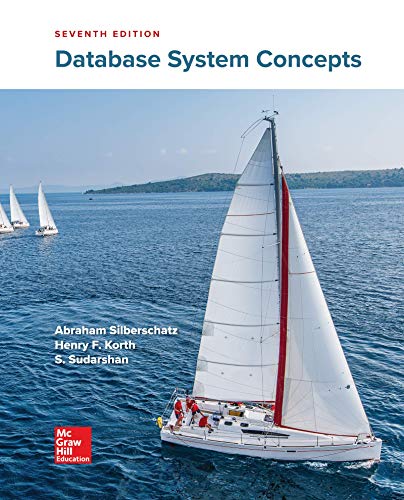
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
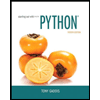
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
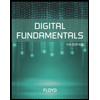
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
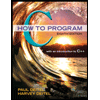
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
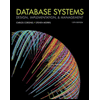
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
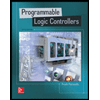
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education