I need help with this code for my class assigment. I have paste the code and the instructions. "Write a function named alternate(s1,s2) that takes as input two sequences of integers, s1 and s2, and returns a sequence consisting of the first integer in s1, then the first integer in s2, then the second integer in s1, then the second integer in s2, and so forth. If the two sequences are not of equal length, the remaining integers in the longer sequence are listed at the end. Use the alternate(s1,s2) function in a program alternating any two (finite) sequences of integers." #include #include // Function to alternate elements from two sequences std::vector alternate(const std::vector& s1, const std::vector& s2) { std::vector result; int i = 0, j = 0; // Alternate elements from both vectors while (i < s1.size() && j < s2.size()) { result.push_back(s1[i++]); // Add element from s1 and increment i result.push_back(s2[j++]); // Add element from s2 and increment j } // Append remaining elements from s1, if any while (i < s1.size()) { result.push_back(s1[i++]); // Add remaining elements from s1 } // Append remaining elements from s2, if any while (j < s2.size()) { result.push_back(s2[j++]); // Add remaining elements from s2 } return result; } int main() { // Example sequences std::vector s1 = {1, 3, 5, 7}; std::vector s2 = {2, 4, 6, 8, 10, 12}; // Get the alternated sequence std::vector result = alternate(s1, s2); // Print the alternated sequence std::cout << "Alternated sequence: "; for (int num : result) { std::cout << num << " "; } std::cout << std::endl; return 0; }
I need help with this code for my class assigment. I have paste the code and the instructions.
"Write a function named alternate(s1,s2) that takes as input two sequences of integers, s1 and s2, and returns a sequence consisting of the first integer in s1, then the first integer in s2, then the second integer in s1, then the second integer in s2, and so forth. If the two sequences are not of equal length, the remaining integers in the longer sequence are listed at the end. Use the alternate(s1,s2) function in a program alternating any two (finite) sequences of integers."
#include <iostream>
#include <vector>
// Function to alternate elements from two sequences
std::vector<int> alternate(const std::vector<int>& s1, const std::vector<int>& s2) {
std::vector<int> result;
int i = 0, j = 0;
// Alternate elements from both
while (i < s1.size() && j < s2.size()) {
result.push_back(s1[i++]); // Add element from s1 and increment i
result.push_back(s2[j++]); // Add element from s2 and increment j
}
// Append remaining elements from s1, if any
while (i < s1.size()) {
result.push_back(s1[i++]); // Add remaining elements from s1
}
// Append remaining elements from s2, if any
while (j < s2.size()) {
result.push_back(s2[j++]); // Add remaining elements from s2
}
return result;
}
int main() {
// Example sequences
std::vector<int> s1 = {1, 3, 5, 7};
std::vector<int> s2 = {2, 4, 6, 8, 10, 12};
// Get the alternated sequence
std::vector<int> result = alternate(s1, s2);
// Print the alternated sequence
std::cout << "Alternated sequence: ";
for (int num : result) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}

Step by step
Solved in 4 steps with 2 images

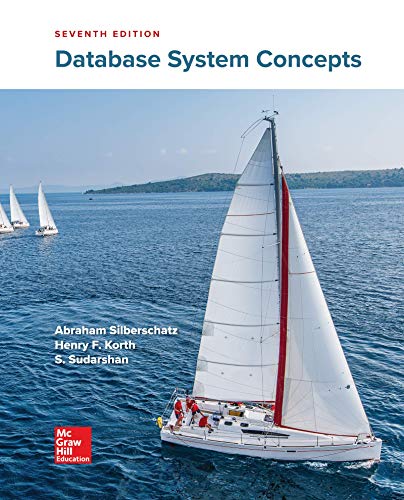
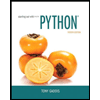
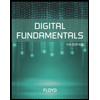
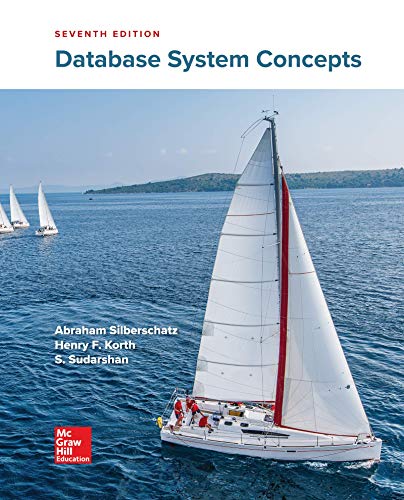
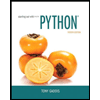
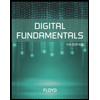
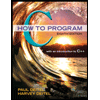
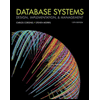
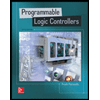