I need help with these, C Programming
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
I need help with these, C

Transcribed Image Text:### 3.12.2: Bool in branching statements
**Challenge Activity**
Write an if-else statement to describe an integer. Print "Positive even number" if `isEven` and `isPositive` are both true. Print "Positive number" if `isEven` is false and `isPositive` is true. Print "Not a positive number" otherwise. End with newline.
#### Learn how our autograder works
Here's the code snippet provided for the activity:
```c
#include <stdio.h>
#include <stdbool.h>
int main(void) {
int userNum;
bool isPositive;
bool isEven;
scanf("%d", &userNum);
isPositive = (userNum > 0);
isEven = ((userNum % 2) == 0);
/* Your solution goes here */
return 0;
}
```
### Explanation:
1. **Include Libraries:**
- `#include <stdio.h>`: Includes the standard input-output library for using `scanf` and `printf`.
- `#include <stdbool.h>`: Includes the boolean data type (bool) and constants `true` and `false`.
2. **Main Function:**
- `int main(void)`: Defines the main function of the program.
3. **Variable Declarations:**
- `int userNum;`: An integer variable to store the user's input number.
- `bool isPositive;`: A boolean variable to indicate if the number is positive.
- `bool isEven;`: A boolean variable to indicate if the number is even.
4. **Input Reading:**
- `scanf("%d", &userNum);`: Reads an integer from the user and stores it in `userNum`.
5. **Boolean Evaluations:**
- `isPositive = (userNum > 0);`: Evaluates if `userNum` is greater than 0.
- `isEven = ((userNum % 2) == 0);`: Evaluates if `userNum` is evenly divisible by 2 (i.e., is an even number).
6. **Your Solution Goes Here:**
Insert an if-else statement to print the correct message based on the values of `isPositive` and `isEven`.
Example Solution:
```c
if (isEven && isPositive) {
printf("Positive even number\n");

Transcribed Image Text:### Section 3.12: Boolean Data Type
#### 3.12.1: Using Bool
**Challenge Activity**
**Problem Statement:**
Assign `isTeenager` with `true` if `kidAge` is 13 to 19 inclusive. Otherwise, assign `isTeenager` with `false`.
**Detailed Explanation:**
```c
#include <stdio.h>
#include <stdbool.h>
int main(void) {
bool isTeenager;
int kidAge;
scanf("%d", &kidAge);
if (kidAge>=13 && kidAge<=19) {
isTeenager=true;
}
else {
isTeenager=false;
}
if (isTeenager) {
printf("Teen");
} else {
printf("Not teen");
}
return 0;
}
```
**Issues in the Code:**
There are compilation errors in the code:
1. **Error at line 13: `else` without a previous `if`**
- The first 'else if' is not needed because it's supposed to pair with a preceding 'if.'
- Correct it by just using `else`.
2. **Error at line 13 & 14: expected `(` before `isTeenager=false;`**
- There is an error in syntax. Correct the structure of `else` to avoid this error.
**Corrected Code:**
```c
#include <stdio.h>
#include <stdbool.h>
int main(void) {
bool isTeenager;
int kidAge;
scanf("%d", &kidAge);
if (kidAge>=13 && kidAge<=19) {
isTeenager=true;
} else {
isTeenager=false;
}
if (isTeenager) {
printf("Teen");
} else {
printf("Not teen");
}
return 0;
}
```
**Explanation of the Logic:**
1. **Initialization:**
- A boolean variable `isTeenager` and an integer variable `kidAge` are declared.
2. **Reading Input:**
- `scanf` is used to read an integer value for `kidAge`.
3. **Conditional Check:**
- If `kidAge` is between 13 and 19 (inclusive), `isTeenager` is set to `true`.
- Otherwise, `isTeenager`
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
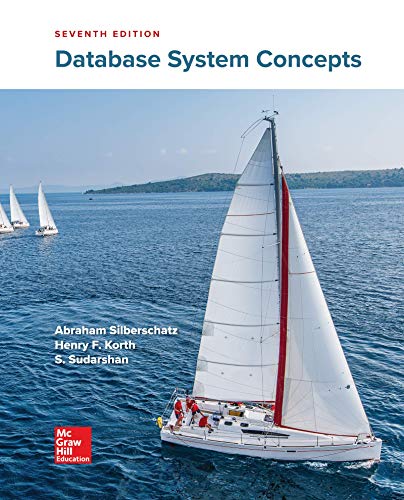
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
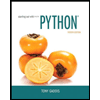
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
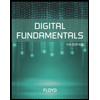
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
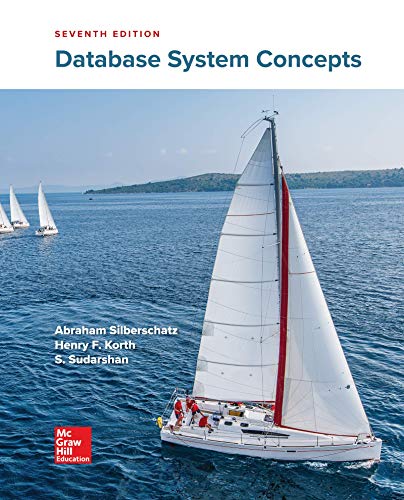
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
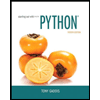
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
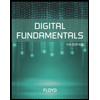
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
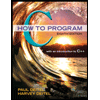
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
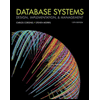
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
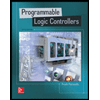
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education