I need help with the following Python 3 program: Given a distance (in kilometers) and a finish time (in hours & minutes), you want tofigure out three things:1. Miles ran, converted from kilometers.2. What was the average pace per mile?3. What was the average miles per hour? Write a python program that will figure this out for you and your running buddies. Your programshould prompt the user for three inputs: 1. Number of kilometers they ran, a floating-point number2. Number of hours, a whole number3. Number of minutes, a whole number You can use any wording you like to gather information for the user, it doesn’t have to match ourexample below. However, the order in which you prompt them must be the same. For grading,we test your code using auto-generated input in a specific order. Ask the user for (1) distance,then (2) hours, then (3) minutes We can use the following test case: 10 k race, 1 hour and 1 minute6.21 miles, 9:49 pace, 6.11 MPH Things to Note: ● There are 1.61 kilometers in a mile● You’ll want the number of miles rounded off to two decimal places, similar to what wedid in lab this week, but not identical -- if it’s a whole number of miles, print 10.0 not 10.00.● Python’s math library has a floor function should you need it, which will tell you thelargest integer value less than or equal to a number. You need to import the math libraryto use it, so you need two steps to make this part hapen: ○ At the top of your .py file, include the line import math ○ When you need to know the floor of a number, use the function math.floor, as in math.floor(10.85), which would give you back just a plain old 10. ● Prompt the user for the number of kilometers, hours, and minutes -- in that order.● Compute the total number of miles ran, rounded off to two decimal places● Compute the minutes and seconds pace-per-mile (head’s up!!! If you do somecalculation and end up with, say, 10.8 minutes, that does not mean 10 minutes and 80seconds... it means 10 minutes and 8/10 of a minute. You need to make that conversioninto minutes and seconds.)● Compute the average miles per hour, rounded off to two decimal places● Report all of this information to the user ● Use a constant variable to store the number of kilometers in a mile.● Use round or format functions only for printing out, and do not save any values that havebeen rounded off, floored, etc. Example Output: How many kilometers did you run?10 How many hours did it take you?1 How many minutes?1 You ran 6.21 milesYour pace: 9 min 49 sec per mileYour MPH: 6.11 >>>
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
I need help with the following Python 3
Given a distance (in kilometers) and a finish time (in hours & minutes), you want to
figure out three things:
1. Miles ran, converted from kilometers.
2. What was the average pace per mile?
3. What was the average miles per hour?
Write a python program that will figure this out for you and your running buddies. Your program
should prompt the user for three inputs:
1. Number of kilometers they ran, a floating-point number
2. Number of hours, a whole number
3. Number of minutes, a whole number
You can use any wording you like to gather information for the user, it doesn’t have to match our
example below. However, the order in which you prompt them must be the same. For grading,
we test your code using auto-generated input in a specific order. Ask the user for (1) distance,
then (2) hours, then (3) minutes
We can use the following test case:
10 k race, 1 hour and 1 minute
6.21 miles, 9:49 pace, 6.11 MPH
Things to Note:
● There are 1.61 kilometers in a mile
● You’ll want the number of miles rounded off to two decimal places, similar to what we
did in lab this week, but not identical -- if it’s a whole number of miles, print 10.0 not 10.00.
● Python’s math library has a floor function should you need it, which will tell you the
largest integer value less than or equal to a number. You need to import the math library
to use it, so you need two steps to make this part hapen:
○ At the top of your .py file, include the line import math
○ When you need to know the floor of a number, use the function math.floor, as in
math.floor(10.85), which would give you back just a plain old 10.
● Prompt the user for the number of kilometers, hours, and minutes -- in that order.
● Compute the total number of miles ran, rounded off to two decimal places
● Compute the minutes and seconds pace-per-mile (head’s up!!! If you do some
calculation and end up with, say, 10.8 minutes, that does not mean 10 minutes and 80
seconds... it means 10 minutes and 8/10 of a minute. You need to make that conversion
into minutes and seconds.)
● Compute the average miles per hour, rounded off to two decimal places
● Report all of this information to the user
● Use a constant variable to store the number of kilometers in a mile.
● Use round or format functions only for printing out, and do not save any values that have
been rounded off, floored, etc.
Example Output:
How many kilometers did you run?
10
How many hours did it take you?
1
How many minutes?
1
You ran 6.21 miles
Your pace: 9 min 49 sec per mile
Your MPH: 6.11
>>>

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

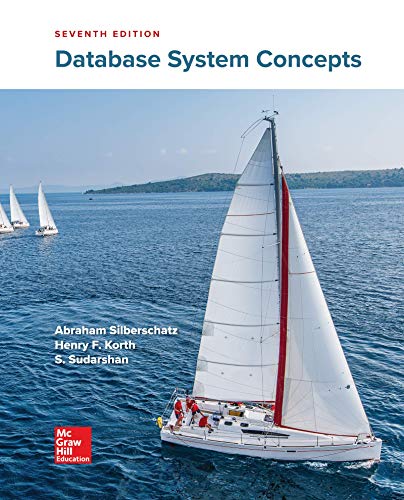
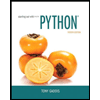
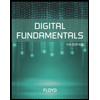
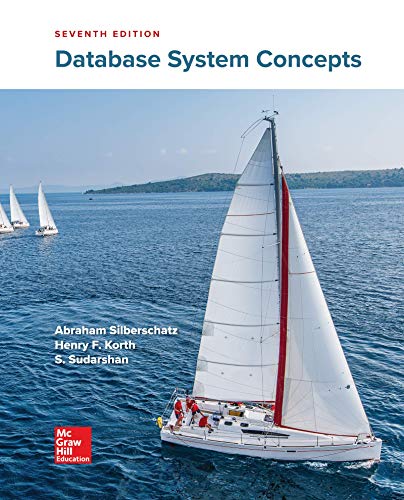
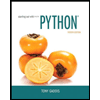
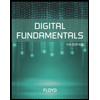
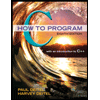
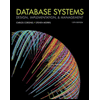
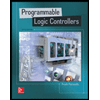