I need help with my java compiler program by Generating intermediate code from the AST
I need help with my java compiler program by Generating intermediate code from the AST, such as three-address code or bytecode
import java.util.*;
public class SimpleCalculator {
private final String input;
private int position;
private boolean hasDivByZero = false;
private Map<String, String> symbolTable;
public SimpleCalculator(String input) {
this.input = input;
this.position = 0;
this.symbolTable = new HashMap<>();
}
public static void main(String[] args) {
SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)");
int result = calculator.parseExpression();
if (calculator.hasDivByZero) {
System.out.println("Error: division by zero");
} else {
System.out.println("Result: " + result);
}
}
public int parseExpression() {
int value = parseTerm();
while (true) {
if (consume('+')) {
value += parseTerm();
} else if (consume('-')) {
value -= parseTerm();
} else {
break;
}
}
return value;
}
public int parseTerm() {
int value = parseFactor();
while (true) {
if (consume('*')) {
value *= parseFactor();
} else if (consume('/')) {
int divisor = parseFactor();
if (divisor == 0) {
hasDivByZero = true;
break;
}
value /= divisor;
} else {
break;
}
}
return value;
}
public int parseFactor() {
if (consume('(')) {
int value = parseExpression();
consume(')');
return value;
}
StringBuilder sb = new StringBuilder();
while (Character.isDigit(peek())) {
sb.append(consume());
}
String variable = sb.toString();
if (symbolTable.containsKey(variable)) {
String type = symbolTable.get(variable);
if (!type.equals("int")) {
System.out.println("Error: " + variable + " is not an integer");
}
} else {
System.out.println("Error: " + variable + " is not defined");
}
return Integer.parseInt(variable);
}
private char consume() {
return input.charAt(position++);
}
private boolean consume(char c) {
if (peek() == c) {
position++;
return true;
}
return false;
}
private char peek() {
return position < input.length() ? input.charAt(position) : '\0';
}
}
please make sure the program runs properly and post the results

Step by step
Solved in 3 steps

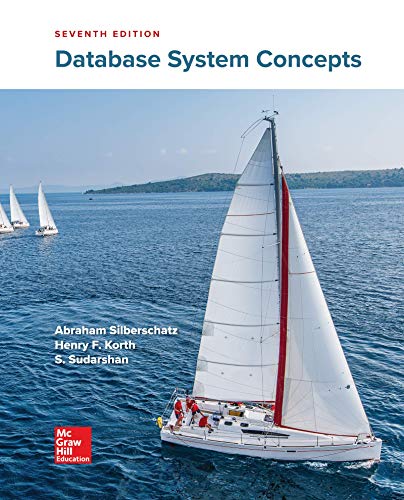
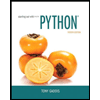
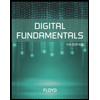
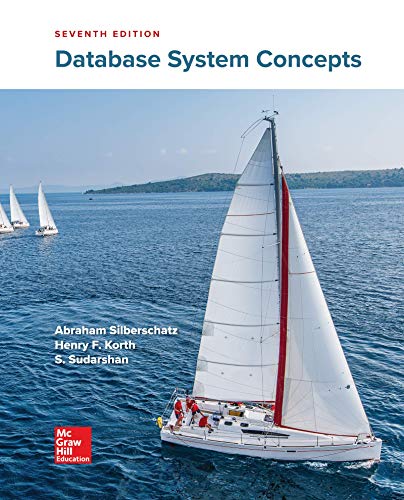
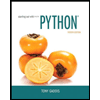
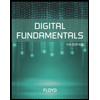
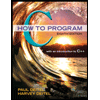
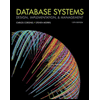
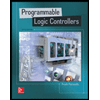