I need help with my java code Implementing a semantic analyzer to check for type errors, undefined variables, and other semantic errors in the program. import java.util.*; public class SimpleCalculator { private final String input; private int position; public SimpleCalculator(String input) { this.input = input; this.position = 0; } public static void main(String[] args) { SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)"); int result = calculator.parseExpression(); System.out.println("Result: " + result); } public int parseExpression() { int value = parseTerm(); while (true) { if (consume('+')) { value += parseTerm(); } else if (consume('-')) { value -= parseTerm(); } else { break; } } return value; } public int parseTerm() { int value = parseFactor(); while (true) { if (consume('*')) { value *= parseFactor(); } else if (consume('/')) { value /= parseFactor(); } else { break; } } return value; } public int parseFactor() { if (consume('(')) { int value = parseExpression(); consume(')'); return value; } StringBuilder sb = new StringBuilder(); while (Character.isDigit(peek())) { sb.append(consume()); } return Integer.parseInt(sb.toString()); } private char consume() { return input.charAt(position++); } private boolean consume(char c) { if (peek() == c) { position++; return true; } return false; } private char peek() { return position < input.length() ? input.charAt(position) : '\0'; }
I need help with my java code
Implementing a semantic analyzer to check for type errors, undefined variables, and other
semantic errors in the program.
import java.util.*;
public class SimpleCalculator {
private final String input;
private int position;
public SimpleCalculator(String input) {
this.input = input;
this.position = 0;
}
public static void main(String[] args) {
SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)");
int result = calculator.parseExpression();
System.out.println("Result: " + result);
}
public int parseExpression() {
int value = parseTerm();
while (true) {
if (consume('+')) {
value += parseTerm();
} else if (consume('-')) {
value -= parseTerm();
} else {
break;
}
}
return value;
}
public int parseTerm() {
int value = parseFactor();
while (true) {
if (consume('*')) {
value *= parseFactor();
} else if (consume('/')) {
value /= parseFactor();
} else {
break;
}
}
return value;
}
public int parseFactor() {
if (consume('(')) {
int value = parseExpression();
consume(')');
return value;
}
StringBuilder sb = new StringBuilder();
while (Character.isDigit(peek())) {
sb.append(consume());
}
return Integer.parseInt(sb.toString());
}
private char consume() {
return input.charAt(position++);
}
private boolean consume(char c) {
if (peek() == c) {
position++;
return true;
}
return false;
}
private char peek() {
return position < input.length() ? input.charAt(position) : '\0';
}

Step by step
Solved in 3 steps

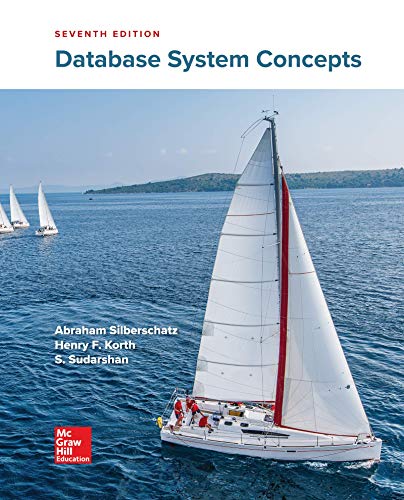
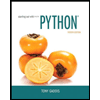
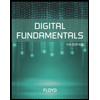
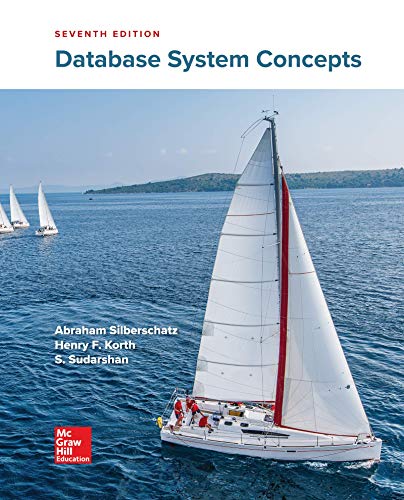
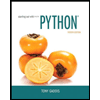
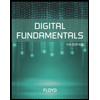
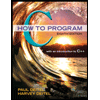
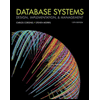
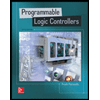