I need help understanding what's wrong with my java code using methods. Task: Complete method printPopcornTime(), with int parameter bagOunces, and void return type. If bagOunces is less than 3, print "Too small". If greater than 10, print "Too large". Otherwise, compute and print 6 * bagOunces followed by "seconds". End with a newline. Example output for ounces = 7 expected output: 42 seconds My code: import java.util.Scanner; public class PopcornTimer { public void printPopcornTime(int bagOunces) { int weight = bagOunces; if (weight < 3) { System.out.println("Too small"); } else if (weight > 10) { System.out.println("Too large"); } else { System.out.prinln(6 * weight + " seconds"); } public static void main (String [] args) { PopcornTimer popcornBag = new PopcornTimer(); popcornBag.printPopcornTime(7); } } What I keep getting: opcornTimer.java:16: error: illegal start of expression public static void main (String [] args) { ^ 1 error
I need help understanding what's wrong with my java code using methods.
Task:
Complete method printPopcornTime(), with int parameter bagOunces, and void return type. If bagOunces is less than 3, print "Too small". If greater than 10, print "Too large". Otherwise, compute and print 6 * bagOunces followed by "seconds". End with a newline. Example output for ounces = 7
expected output: 42 seconds
My code:
import java.util.Scanner;
public class PopcornTimer {
public void printPopcornTime(int bagOunces) {
int weight = bagOunces;
if (weight < 3) {
System.out.println("Too small");
} else if
(weight > 10) {
System.out.println("Too large");
}
else {
System.out.prinln(6 * weight + " seconds");
}
public static void main (String [] args) {
PopcornTimer popcornBag = new PopcornTimer();
popcornBag.printPopcornTime(7);
}
}
What I keep getting:
opcornTimer.java:16: error: illegal start of expression public static void main (String [] args) { ^ 1 error

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

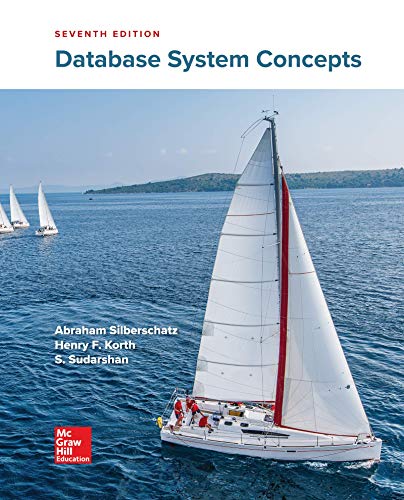
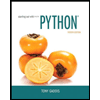
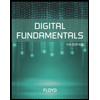
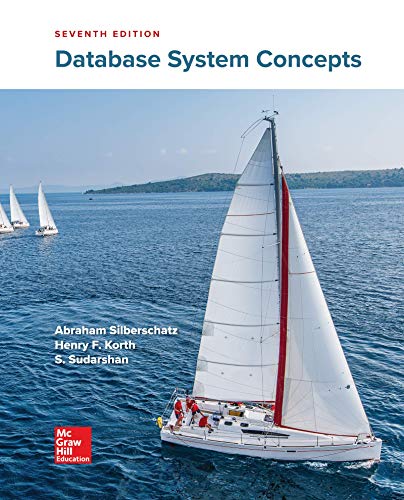
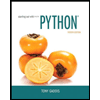
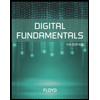
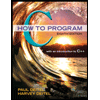
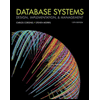
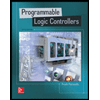