I need help in this C Program. Write the function displayExtractedNumbers() that displays the numbers in the given code in nCode based on the digit information nDigitInfo provided. nCode contains numbers merged as one integer, while nDigitInfo indicates the the number of digits of each number in nCode. Assume the following: nCode = 123456789 and nDigitInfo = 4113: The numbers found in nCode are: 1234, 5, 6, and 789. nCode: 1 2 3 4 5 6 7 8 9 nDigit Info ---------- ---------- ----------> 4 1 1 ------- -------> 3 Assume that the following functions are already defined. Use them in your solution. int getReverse (int nNum) returns an integer whose digits are in reverse order of the given positive integer nNum. Examples: getReverse(1234) returns 4321 getReverse(1) returns 1 int get10RaisedToExp (int nExp) returns the value of 10nExp, where nExp is a nonnegative integer. int extractNumber (int nSource, int nDigits) extracts the rightmost digits of nSource based on the given number of digits nDigits, and returns the number in reverse order of digits. The function returns -1 if it is impossible to extract the number. Examples: extractNumber(12345, 3) returns 543. extractNumber(12, 2) returns 21. extractNumber(123, 4) returns -1. Sample Run- Code: 123876 Digit info: 222 Extracted numbers: 12 38 76 Done int main() int main () { int nCode; int nDigitInfo; printf ("Code: "); scanf ("%d", &nCode); printf ("Digit info: "); scanf ("%d", &nDigitInfo); displayExtractedNumbers (nCode, nDigitInfo); return 0; }int main () { int nCode; int nDigitInfo; printf ("Code: "); scanf ("%d", &nCode); printf ("Digit info: "); scanf ("%d", &nDigitInfo); displayExtractedNumbers (nCode, nDigitInfo); return 0; }
I need help in this C Program.
Write the function displayExtractedNumbers() that displays the numbers in the given code in nCode based on the digit information nDigitInfo provided. nCode contains numbers merged as one integer, while nDigitInfo indicates the the number of digits of each number in nCode.
Assume the following: nCode = 123456789 and nDigitInfo = 4113:
The numbers found in nCode are: 1234, 5, 6, and 789.
nCode: | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
nDigit Info | ---------- | ---------- | ----------> | 4 | 1 | 1 | ------- | -------> | 3 |
Assume that the following functions are already defined. Use them in your solution.
- int getReverse (int nNum)
- returns an integer whose digits are in reverse order of the given positive integer nNum.
- Examples:
- getReverse(1234) returns 4321
- getReverse(1) returns 1
- int get10RaisedToExp (int nExp)
- returns the value of 10nExp, where nExp is a nonnegative integer.
- int extractNumber (int nSource, int nDigits)
- extracts the rightmost digits of nSource based on the given number of digits nDigits, and returns the number in reverse order of digits. The function returns -1 if it is impossible to extract the number.
- Examples:
- extractNumber(12345, 3) returns 543.
- extractNumber(12, 2) returns 21.
- extractNumber(123, 4) returns -1.
Sample Run-
Code: 123876
Digit info: 222
Extracted numbers:
12
38
76
Done
int main()
int main ()
{
int nCode;
int nDigitInfo;
printf ("Code: ");
scanf ("%d", &nCode);
printf ("Digit info: ");
scanf ("%d", &nDigitInfo);
displayExtractedNumbers (nCode, nDigitInfo);
return 0;
}int main ()
{
int nCode;
int nDigitInfo;
printf ("Code: ");
scanf ("%d", &nCode);
printf ("Digit info: ");
scanf ("%d", &nDigitInfo);
displayExtractedNumbers (nCode, nDigitInfo);
return 0;
}

Step by step
Solved in 2 steps with 2 images

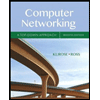
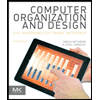
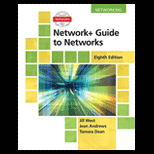
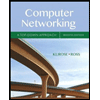
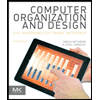
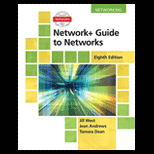
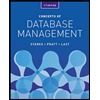
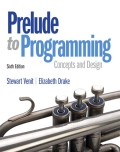
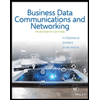