I need help in creating a function based on the program below. The program below prints out the particular line in a text file . I want to modify this program to a function whose parameter is the name of the particular text file and it returns the string present on the specific line. I however also want the particular line number to be randomly generated ranging from 1 till 50. Restrictions are that I can't use break,exit,continue to get out of a loop. #include #include // constants for size of char arrays to store filename, the line from the file #define FILENAME_SIZE 1024 #define MAX_LINE 2048 int main() { // file pointer will be used to open/read the file FILE *file; char filename[FILENAME_SIZE]; char buffer[MAX_LINE]; int read_line = 0; printf("File: "); scanf("%s", filename); printf("Read Line: "); scanf("%d", &read_line); file = fopen(filename, "r"); if (file == NULL) { printf("Error opening file.\n"); return 1; } bool keep_reading = true; int current_line = 1; do { fgets(buffer, MAX_LINE, file); if (feof(file)) { keep_reading = false; printf("File %d lines.\n", current_line-1); printf("Couldn't find line %d.\n", read_line); } else if (current_line == read_line) { keep_reading = false; printf("Line:\n%s", buffer); } current_line++; } while (keep_reading); fclose(file); return 0; }
I need help in creating a function based on the program below. The program below prints out the particular line in a text file . I want to modify this program to a function whose parameter is the name of the particular text file and it returns the string present on the specific line. I however also want the particular line number to be randomly generated ranging from 1 till 50. Restrictions are that I can't use break,exit,continue to get out of a loop.
#include <stdio.h>
#include <stdbool.h>
// constants for size of char arrays to store filename, the line from the file
#define FILENAME_SIZE 1024
#define MAX_LINE 2048
int main()
{
// file pointer will be used to open/read the file
FILE *file;
char filename[FILENAME_SIZE];
char buffer[MAX_LINE];
int read_line = 0;
printf("File: ");
scanf("%s", filename);
printf("Read Line: ");
scanf("%d", &read_line);
file = fopen(filename, "r");
if (file == NULL)
{
printf("Error opening file.\n");
return 1;
}
bool keep_reading = true;
int current_line = 1;
do
{
fgets(buffer, MAX_LINE, file);
if (feof(file))
{
keep_reading = false;
printf("File %d lines.\n", current_line-1);
printf("Couldn't find line %d.\n", read_line);
}
else if (current_line == read_line)
{
keep_reading = false;
printf("Line:\n%s", buffer);
}
current_line++;
} while (keep_reading);
fclose(file);
return 0;
}

Step by step
Solved in 2 steps with 1 images

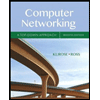
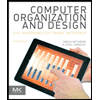
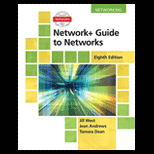
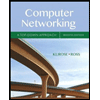
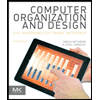
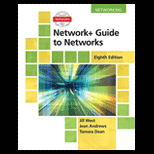
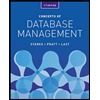
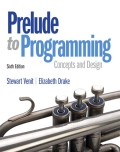
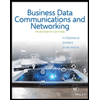