I need help in creating 5 user defined function in C to solve the following problem as shown in the screenshot. Below is the skeleton code for the problem. The 5 user defined functions need to be created in accordance to the screenshot and should be tested in the int main function. The Skeleton Code #include #include typedef char String30[31]; typedef char String60[61]; /* TO DO: Implement the functions as specified in screenshot above RESTRICTIONS: 1. Do NOT call printf() or scanf() in any of the required function definition EXCEPT in the main() function. */ /* Step 1: Define the function that will achieve this step right after this comment. Do NOT call print(), scanf() and any other library functions not indicated above. User defined function has to be made in accordance to step 1 in the screenshot */ /* Step 2: Define the function that will achieve this step right after this comment. Do NOT call print(), scanf() and other library functions that we did not discuss in class. User defined function has to be made in accordance to step 2 in the screenshot */ /* Step 3: Define the function that will achieve this step right after this comment. Do NOT call print(), scanf() and other library functions that we did not discuss in class. User defined function has to be made in accordance to step 3 in the screenshot */ /* Step 4: Define the function that will achieve this step right after this comment. Do NOT call print(), scanf() and other library functions that we did not discuss in class. User defined function has to be made in accordance to step 4 in the screenshot */ /* Step 5: Define the function that will achieve this step right after this comment. Do NOT call print(), scanf() and other library functions that we did not discuss in class. User defined function has to be made in accordance to step 5 in the screenshot */ int main() { String30 firstName, lastName; String60 password; // Do NOT add any printf() for prompts before the scanf() function calls. scanf("%s", firstName); scanf("%s", lastName); // Call Step 1 function after this comment and before the printf() statement. printf("%s\n", password); // prints the password value after step 1. // Call Step 1 function after this comment and before the printf() statement. printf("%s\n", password) // prints the password value after step 1. // Call Step 2 function after this comment and before the printf() statement. printf("%s\n", password); // prints the password value after step 2. // Call Step 3 function after this comment and before the printf() statement. printf("%s\n", password); // prints the password value after step 3. // Call Step 4 function after this comment and before the printf() statement. printf("%s\n", password); // prints the password value after step 4. // Call Step 5 function after this comment and before the printf() statement. printf("%s\n", password); // prints the FINAL password value after step 5. return 0; }
I need help in creating 5 user defined function in C to solve the following problem as shown in the screenshot. Below is the skeleton code for the problem. The 5 user defined functions need to be created in accordance to the screenshot and should be tested in the int main function.
The Skeleton Code
#include <stdio.h>
#include <string.h>
typedef char String30[31];
typedef char String60[61];
/*
TO DO:
Implement the functions as specified in screenshot above
RESTRICTIONS:
1. Do NOT call printf() or scanf() in any of the required function definition
EXCEPT in the main() function.
*/
/*
Step 1: Define the function that will achieve this step right after this comment.
Do NOT call print(), scanf() and any other library functions not indicated above.
User defined function has to be made in accordance to step 1 in the screenshot
*/
/*
Step 2: Define the function that will achieve this step right after this comment.
Do NOT call print(), scanf() and other library functions that we did not
discuss in class.
User defined function has to be made in accordance to step 2 in the screenshot
*/
/*
Step 3: Define the function that will achieve this step right after this comment.
Do NOT call print(), scanf() and other library functions that we did not
discuss in class.
User defined function has to be made in accordance to step 3 in the screenshot
*/
/*
Step 4: Define the function that will achieve this step right after this comment.
Do NOT call print(), scanf() and other library functions that we did not
discuss in class.
User defined function has to be made in accordance to step 4 in the screenshot
*/
/*
Step 5: Define the function that will achieve this step right after this comment.
Do NOT call print(), scanf() and other library functions that we did not
discuss in class.
User defined function has to be made in accordance to step 5 in the screenshot
*/
int main()
{
String30 firstName, lastName;
String60 password;
// Do NOT add any printf() for prompts before the scanf() function calls.
scanf("%s", firstName);
scanf("%s", lastName);
// Call Step 1 function after this comment and before the printf() statement.
printf("%s\n", password); // prints the password value after step 1.
// Call Step 1 function after this comment and before the printf() statement.
printf("%s\n", password) // prints the password value after step 1.
// Call Step 2 function after this comment and before the printf() statement.
printf("%s\n", password); // prints the password value after step 2.
// Call Step 3 function after this comment and before the printf() statement.
printf("%s\n", password); // prints the password value after step 3.
// Call Step 4 function after this comment and before the printf() statement.
printf("%s\n", password); // prints the password value after step 4.
// Call Step 5 function after this comment and before the printf() statement.
printf("%s\n", password); // prints the FINAL password value after step 5.
return 0;
}


Step by step
Solved in 3 steps with 2 images

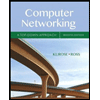
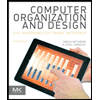
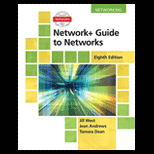
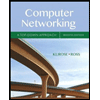
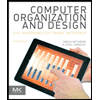
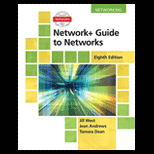
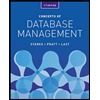
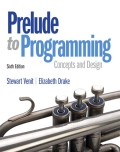
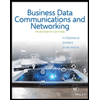