I need help fixing this python code so that it can display the output like shown below class FoodItem: def __init__(self): self.name = "Water" self.fat = 0.00 self.carbs = 0.00 self.protein = 0.00 def foodItem(self,name,fat,carbs,protein,servings): print(f"\nNutritional information per serving of {name}:") if name == self.name: print("Enter food name: Enter fat:") else: print(f'Fat: {fat}g') print(f'Carbohydrates: {carbs}g') print(f'Protein: {protein}g') calories = fat*9 + carbs*4 + protein*4 print(f'Number of calories for 1.00 serving(s): {calories}') print(f'Number of calories for {servings} serving(s): {servings*calories}') if __name__ == '__main__': name = input("Enter food name: ") fat = float(input("Enter fat: ")) carbs = float(input("Enter carbs: ")) protein = float(input("Enter protein: ")) servings = float(input("Enter number of servings: ")) food = FoodItem() food.foodItem(name,fat,carbs,protein,servings)
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
I need help fixing this python code so that it can display the output like shown below
class FoodItem:
def __init__(self):
self.name = "Water"
self.fat = 0.00
self.carbs = 0.00
self.protein = 0.00
def foodItem(self,name,fat,carbs,protein,servings):
print(f"\nNutritional information per serving of {name}:")
if name == self.name:
print("Enter food name: Enter fat:")
else:
print(f'Fat: {fat}g')
print(f'Carbohydrates: {carbs}g')
print(f'Protein: {protein}g')
calories = fat*9 + carbs*4 + protein*4
print(f'Number of calories for 1.00 serving(s): {calories}')
print(f'Number of calories for {servings} serving(s): {servings*calories}')
if __name__ == '__main__':
name = input("Enter food name: ")
fat = float(input("Enter fat: "))
carbs = float(input("Enter carbs: "))
protein = float(input("Enter protein: "))
servings = float(input("Enter number of servings: "))
food = FoodItem()
food.foodItem(name,fat,carbs,protein,servings)


Step by step
Solved in 3 steps with 3 images

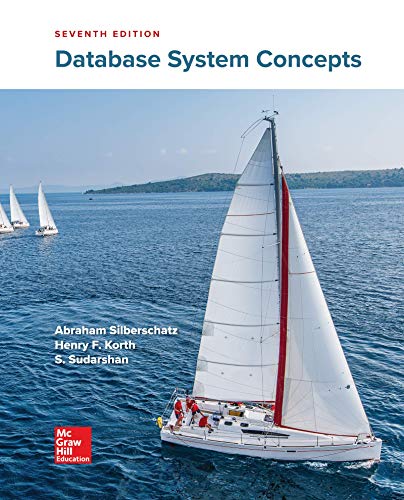
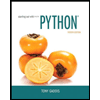
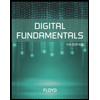
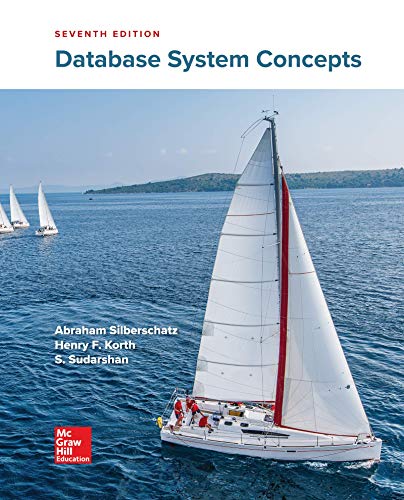
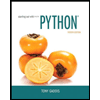
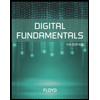
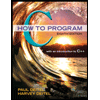
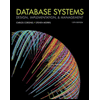
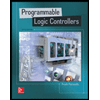