I have these two exercises and their answers, but I am not quite sure what is the behavior and why those are the results. I will really appreciate a detailed explanation. Thank you.
I have these two exercises and their answers, but I am not quite sure what is the behavior and why those are the results. I will really appreciate a detailed explanation. Thank you.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I have these two exercises and their answers, but I am not quite sure what is the behavior and why those are the results. I will really appreciate a detailed explanation. Thank you.

Transcribed Image Text:### Understanding the `mystery` Method in Java
Given the following method in Java:
```java
public static void mystery(int x) {
int y = 1;
int z = 0;
while (2 * y <= x) {
y = y * 2;
z++;
}
System.out.println(y + " " + z);
}
```
This method takes an integer `x` as input and performs the following operations:
- It initializes `y` to 1 and `z` to 0.
- It then enters a `while` loop that runs as long as `2 * y` is less than or equal to `x`.
- Inside the loop, `y` is doubled, and `z` is incremented by 1.
- Finally, it prints the values of `y` and `z`.
Let's analyze the output of this method for different values of `x`.
### Outputs for Various Calls
| Method Call | Output |
|---------------|--------|
| mystery(1) | 1 0 |
| mystery(6) | 4 2 |
| mystery(19) | 16 4 |
| mystery(39) | 32 5 |
| mystery(74) | 64 6 |
Understanding these results:
- For `mystery(1)`: The while condition `2 * y <= 1` is not met initially, so the loop does not execute, resulting in `y = 1` and `z = 0`.
- For `mystery(6)`:
- Initial (`y = 1`, `z = 0`).
- First iteration (`y = 1 * 2 = 2`, `z = 1`).
- Second iteration (`y = 2 * 2 = 4`, `z = 2`).
- Loop condition fails on the next check (`2 * 4 = 8`, which is not `<= 6`).
- Result is `y = 4` and `z = 2`.
Similar reasoning can be applied for the other function calls, leading to the observed outputs.
This method demonstrates how to implement a sequence of operations based on a condition and is useful for understanding loops and conditionals in programming.
![## Code Analysis and Array Transformation
The following code contains a method named `mystery` that modifies the first array using elements from the second array:
```java
public static void mystery(int[] a, int[] b) {
for (int i = 0; i < a.length; i++) {
a[i] += b[b.length - 1 - i];
}
}
```
### Problem Statement
What are the values of the elements in array `a1` after the following code executes?
```java
int[] a1 = {1, 3, 5, 7, 9};
int[] a2 = {1, 4, 9, 16, 25};
mystery(a1, a2);
```
### Array Contents Before Function Call
- `a1`: {1, 3, 5, 7, 9}
- `a2`: {1, 4, 9, 16, 25}
### Explanation
The method iterates through each element in array `a1` and adds the corresponding element from array `a2`, starting from the last element of `a2`:
1. In the first iteration (`i = 0`):
- `a1[0] += a2[4]` => `1 += 25` => `a1[0] = 26`
2. In the second iteration (`i = 1`):
- `a1[1] += a2[3]` => `3 += 16` => `a1[1] = 19`
3. In the third iteration (`i = 2`):
- `a1[2] += a2[2]` => `5 += 9` => `a1[2] = 14`
4. In the fourth iteration (`i = 3`):
- `a1[3] += a2[1]` => `7 += 4` => `a1[3] = 11`
5. In the fifth iteration (`i = 4`):
- `a1[4] += a2[0]` => `9 += 1` => `a1[4] = 10`
### Array Contents After Function Call
- `a1`: {26, 19, 14, 11,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F416064f9-c57b-46ee-9b50-14b257515654%2F70199959-f05a-46d6-a98f-62421fb09e97%2Fbeeic79.png&w=3840&q=75)
Transcribed Image Text:## Code Analysis and Array Transformation
The following code contains a method named `mystery` that modifies the first array using elements from the second array:
```java
public static void mystery(int[] a, int[] b) {
for (int i = 0; i < a.length; i++) {
a[i] += b[b.length - 1 - i];
}
}
```
### Problem Statement
What are the values of the elements in array `a1` after the following code executes?
```java
int[] a1 = {1, 3, 5, 7, 9};
int[] a2 = {1, 4, 9, 16, 25};
mystery(a1, a2);
```
### Array Contents Before Function Call
- `a1`: {1, 3, 5, 7, 9}
- `a2`: {1, 4, 9, 16, 25}
### Explanation
The method iterates through each element in array `a1` and adds the corresponding element from array `a2`, starting from the last element of `a2`:
1. In the first iteration (`i = 0`):
- `a1[0] += a2[4]` => `1 += 25` => `a1[0] = 26`
2. In the second iteration (`i = 1`):
- `a1[1] += a2[3]` => `3 += 16` => `a1[1] = 19`
3. In the third iteration (`i = 2`):
- `a1[2] += a2[2]` => `5 += 9` => `a1[2] = 14`
4. In the fourth iteration (`i = 3`):
- `a1[3] += a2[1]` => `7 += 4` => `a1[3] = 11`
5. In the fifth iteration (`i = 4`):
- `a1[4] += a2[0]` => `9 += 1` => `a1[4] = 10`
### Array Contents After Function Call
- `a1`: {26, 19, 14, 11,
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
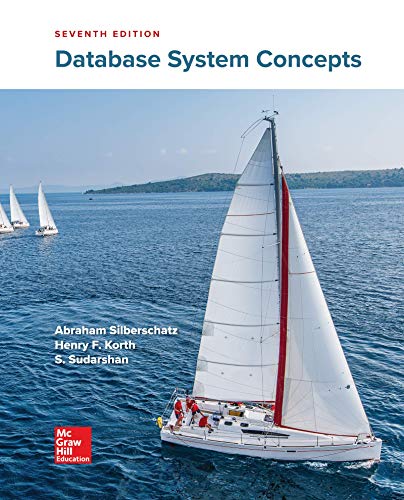
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
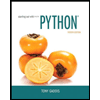
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
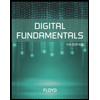
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
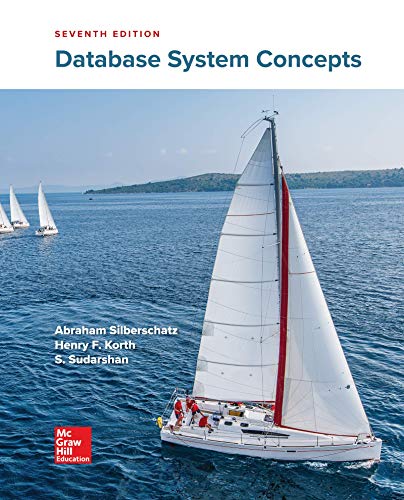
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
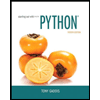
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
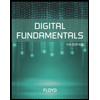
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
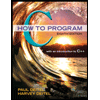
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
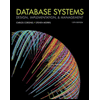
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
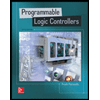
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education