I have a server code: import socket # Define the server address and port server_address = ('localhost', 12345) # Create a TCP socket server_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Bind the socket to the server address server_sock.bind(server_address) # Listen for incoming connections server_sock.listen(1) while True: print('Waiting for a connection...') # Accept a client connection client_sock, client_address = server_sock.accept() print('Connected to:', client_address) try: # Receive data from the client data = client_sock.recv(1024) # Process the received data processed_data = data.swapcase() # Send the processed data back to the client client_sock.sendall(processed_data) finally: # Close the client socket client_sock.close() print('Connection closed.') break # Close the server socket server_sock.close() And the Client code: import argparse import socket # Parse the command-line arguments parser = argparse.ArgumentParser(description="This is a very basic client program") parser.add_argument('-f', type=str, help='Source file for the strings to reverse', default='source_strings.txt', action='store', dest='in_file') parser.add_argument('-o', type=str, help='Destination file for the reversed strings', default='results.txt', action='store', dest='out_file') parser.add_argument('server_location', type=str, help='Domain name or IP address of the server', action='store') parser.add_argument('port', type=int, help='Port to connect to the server on', action='store') args = parser.parse_args() # Create a client socket client_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to the server server_addr = (args.server_location, args.port) client_sock.connect(server_addr) # Open the source and destination files with open(args.out_file, 'wb') as write_file, open(args.in_file, 'rb') as read_file: # Read each line from the source file for line in read_file: # Send the line to the server client_sock.sendall(line) # Receive the processed line from the server processed_line = client_sock.recv(512) # Write the processed line to the destination file write_file.write(processed_line) # Close the client socket client_sock.close() I need the server code to be modified to make sure that the program would give valid results even if both server and client are run on separate machines and also on local or remote socket connections. NOTE: Right now the server is hard coded to a certain port number and I would like to make it work with different port numbers as long as the connection is established. Pictures of the code working fine would be appreciated.
I have a server code: import socket # Define the server address and port server_address = ('localhost', 12345) # Create a TCP socket server_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Bind the socket to the server address server_sock.bind(server_address) # Listen for incoming connections server_sock.listen(1) while True: print('Waiting for a connection...') # Accept a client connection client_sock, client_address = server_sock.accept() print('Connected to:', client_address) try: # Receive data from the client data = client_sock.recv(1024) # Process the received data processed_data = data.swapcase() # Send the processed data back to the client client_sock.sendall(processed_data) finally: # Close the client socket client_sock.close() print('Connection closed.') break # Close the server socket server_sock.close() And the Client code: import argparse import socket # Parse the command-line arguments parser = argparse.ArgumentParser(description="This is a very basic client program") parser.add_argument('-f', type=str, help='Source file for the strings to reverse', default='source_strings.txt', action='store', dest='in_file') parser.add_argument('-o', type=str, help='Destination file for the reversed strings', default='results.txt', action='store', dest='out_file') parser.add_argument('server_location', type=str, help='Domain name or IP address of the server', action='store') parser.add_argument('port', type=int, help='Port to connect to the server on', action='store') args = parser.parse_args() # Create a client socket client_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to the server server_addr = (args.server_location, args.port) client_sock.connect(server_addr) # Open the source and destination files with open(args.out_file, 'wb') as write_file, open(args.in_file, 'rb') as read_file: # Read each line from the source file for line in read_file: # Send the line to the server client_sock.sendall(line) # Receive the processed line from the server processed_line = client_sock.recv(512) # Write the processed line to the destination file write_file.write(processed_line) # Close the client socket client_sock.close() I need the server code to be modified to make sure that the program would give valid results even if both server and client are run on separate machines and also on local or remote socket connections. NOTE: Right now the server is hard coded to a certain port number and I would like to make it work with different port numbers as long as the connection is established. Pictures of the code working fine would be appreciated.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I have a server code:
import socket
# Define the server address and port
server_address = ('localhost', 12345)
# Create a TCP socket
server_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Bind the socket to the server address
server_sock.bind(server_address)
# Listen for incoming connections
server_sock.listen(1)
while True:
print('Waiting for a connection...')
# Accept a client connection
client_sock, client_address = server_sock.accept()
print('Connected to:', client_address)
try:
# Receive data from the client
data = client_sock.recv(1024)
# Process the received data
processed_data = data.swapcase()
# Send the processed data back to the client
client_sock.sendall(processed_data)
finally:
# Close the client socket
client_sock.close()
print('Connection closed.')
break
# Close the server socket
server_sock.close()
And the Client code:
import argparse
import socket
# Parse the command-line arguments
parser = argparse.ArgumentParser(description="This is a very basic client program")
parser.add_argument('-f', type=str, help='Source file for the strings to reverse', default='source_strings.txt', action='store', dest='in_file')
parser.add_argument('-o', type=str, help='Destination file for the reversed strings', default='results.txt', action='store', dest='out_file')
parser.add_argument('server_location', type=str, help='Domain name or IP address of the server', action='store')
parser.add_argument('port', type=int, help='Port to connect to the server on', action='store')
args = parser.parse_args()
# Create a client socket
client_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Connect to the server
server_addr = (args.server_location, args.port)
client_sock.connect(server_addr)
# Open the source and destination files
with open(args.out_file, 'wb') as write_file, open(args.in_file, 'rb') as read_file:
# Read each line from the source file
for line in read_file:
# Send the line to the server
client_sock.sendall(line)
# Receive the processed line from the server
processed_line = client_sock.recv(512)
# Write the processed line to the destination file
write_file.write(processed_line)
# Close the client socket
client_sock.close()
I need the server code to be modified to make sure that the program would give valid results even if both server and client are run on separate machines and also on local or remote socket connections.
NOTE: Right now the server is hard coded to a certain port number and I would like to make it work with different port numbers as long as the connection is established.
Pictures of the code working fine would be appreciated.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
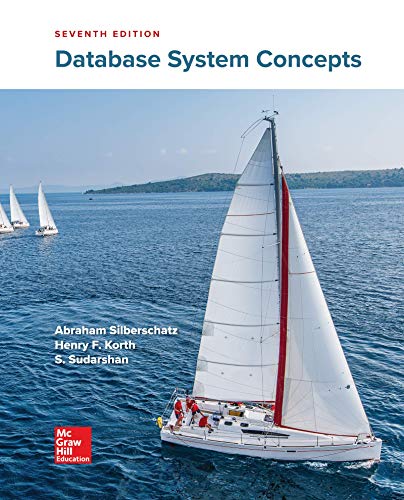
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
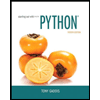
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
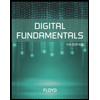
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
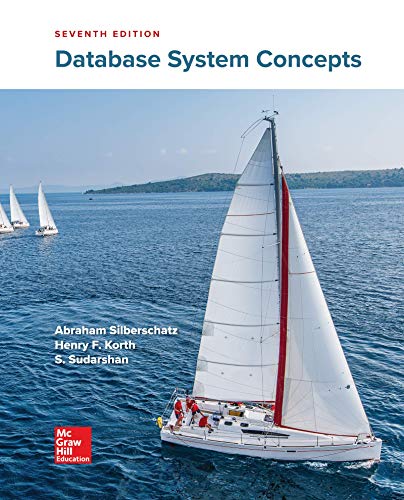
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
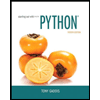
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
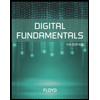
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
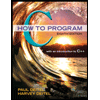
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
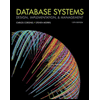
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
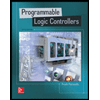
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education