I have a django project that I am working on an I am trying to add a UserList to my Allgroups class. However, I'm getting these errors: ERRORS: allgroups.Allgroups.UserList: (fields.E304) Reverse accessor for 'Allgroups.UserList' clashes with reverse accessor for 'Allgroups.author'. HINT: Add or change a related_name argument to the definition for 'Allgroups.UserList' or 'Allgroups.author'. allgroups.Allgroups.author: (fields.E304) Reverse accessor for 'Allgroups.author' clashes with reverse accessor for 'Allgroups.UserList'. HINT: Add or change a related_name argument to the definition for 'Allgroups.author' or 'Allgroups.UserList'. I need both an author and a UserList for the Allgroups class, so I'm not sure what I need to do.
I have a django project that I am working on an I am trying to add a UserList to my Allgroups class. However, I'm getting these errors:
ERRORS:
allgroups.Allgroups.UserList: (fields.E304) Reverse accessor for 'Allgroups.UserList' clashes with reverse accessor for 'Allgroups.author'.
HINT: Add or change a related_name argument to the definition for 'Allgroups.UserList' or 'Allgroups.author'.
allgroups.Allgroups.author: (fields.E304) Reverse accessor for 'Allgroups.author' clashes with reverse accessor for 'Allgroups.UserList'.
HINT: Add or change a related_name argument to the definition for 'Allgroups.author' or 'Allgroups.UserList'.
I need both an author and a UserList for the Allgroups class, so I'm not sure what I need to do.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

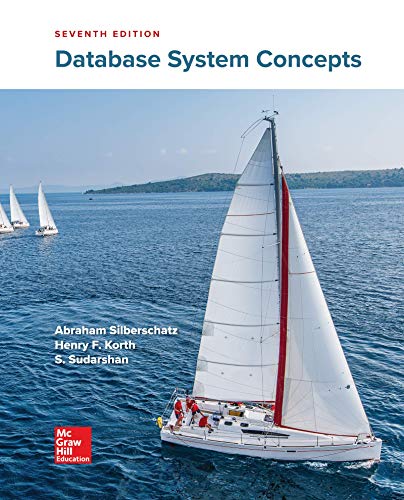
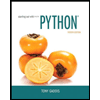
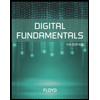
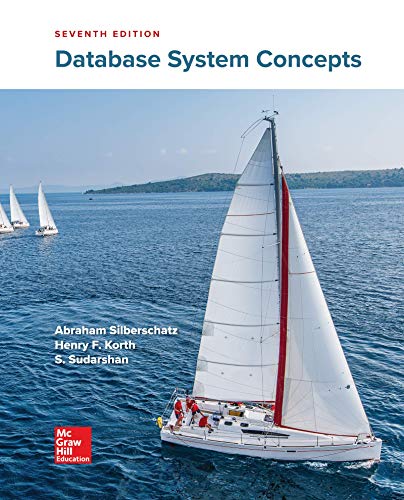
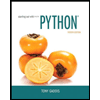
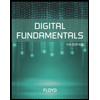
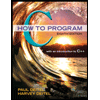
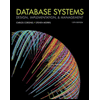
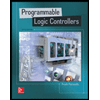