I am writing a program that calculates the are of a triangle using the following formula: Area = (a2/2) * [sin α1 * sin αmgle2)/sin(αngle1 + αngle2). I am having issues with the angles because the angles need to always be greater than 0 and the sum of the 2 angles need to always be less than 180. Also, the area can't be a negative number. I almost got it down, but of course with an if statement nothing will print in the terminal if the conditions aren't meet. I've tried with a while loop, but was unsuccessful with that. What can I do so that this condition if(my_rank != 0 && alpha1 >= 1 && alpha2 >= 1 && alpha1 + alpha2 < 180) is always met? int a; int b; float c; bool m = false; alpha1 = rand()%180; alpha2 = rand()%180; baseA= (((float)rand()/(float)(RAND_MAX)) * 11 + 10) + ((float)rand()/(float)(RAND_MAX)); if(my_rank != 0 && alpha1 >= 1 && alpha2 >= 1 && alpha1 + alpha2 < 180){ m = true; MPI_Send(&alpha1, 1, MPI_FLOAT, 0, 0, MPI_COMM_WORLD); MPI_Send(&alpha2, 1, MPI_INT, 0, 0, MPI_COMM_WORLD); MPI_Send(&baseA, 1, MPI_INT, 0, 0, MPI_COMM_WORLD); printf("a = %.2f, alpha1 = %d, alpha2 = %d \n", baseA, alpha1, alpha2); }else{ a = alpha1; b = alpha2; c = baseA; area = areaTri(baseA, alpha1, alpha2); for(i = 1; i < comm_sz; i++){ MPI_Recv(&alpha1, 1, MPI_FLOAT, i, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE); MPI_Recv(&alpha2, 1, MPI_INT, i, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE); MPI_Recv(&baseA, 1, MPI_INT, i, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE); area = areaTri(baseA, alpha1, alpha2); my_rank = my_rank + i; } printf("a = %.2f, alpha1 = %d, alpha2 = %d \n", c, a, b); printf("Area of %d triangles is %.2f\n", my_rank, area); } MPI_Finalize(); return 0; } float areaTri (int base, float alpha1, float alpha2){ float area = pow(base, 2) * (sin(alpha1) * sin(alpha2)/sin(alpha1 +alpha2)); return area; }
I am writing a program that calculates the are of a triangle using the following formula: Area = (a2/2) * [sin α1 * sin αmgle2)/sin(αngle1 + αngle2).
I am having issues with the angles because the angles need to always be greater than 0 and the sum of the 2 angles need to always be less than 180. Also, the area can't be a negative number. I almost got it down, but of course with an if statement nothing will print in the terminal if the conditions aren't meet. I've tried with a while loop, but was unsuccessful with that. What can I do so that this condition if(my_rank != 0 && alpha1 >= 1 && alpha2 >= 1 && alpha1 + alpha2 < 180) is always met?
int a;
int b;
float c;
bool m = false;
alpha1 = rand()%180;
alpha2 = rand()%180;
baseA= (((float)rand()/(float)(RAND_MAX)) * 11 + 10) + ((float)rand()/(float)(RAND_MAX));
if(my_rank != 0 && alpha1 >= 1 && alpha2 >= 1 && alpha1 + alpha2 < 180){
m = true;
MPI_Send(&alpha1, 1, MPI_FLOAT, 0, 0, MPI_COMM_WORLD);
MPI_Send(&alpha2, 1, MPI_INT, 0, 0, MPI_COMM_WORLD);
MPI_Send(&baseA, 1, MPI_INT, 0, 0, MPI_COMM_WORLD);
printf("a = %.2f, alpha1 = %d, alpha2 = %d \n", baseA, alpha1, alpha2);
}else{
a = alpha1;
b = alpha2;
c = baseA;
area = areaTri(baseA, alpha1, alpha2);
for(i = 1; i < comm_sz; i++){
MPI_Recv(&alpha1, 1, MPI_FLOAT, i, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
MPI_Recv(&alpha2, 1, MPI_INT, i, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
MPI_Recv(&baseA, 1, MPI_INT, i, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
area = areaTri(baseA, alpha1, alpha2);
my_rank = my_rank + i;
}
printf("a = %.2f, alpha1 = %d, alpha2 = %d \n", c, a, b);
printf("Area of %d triangles is %.2f\n", my_rank, area);
}
MPI_Finalize();
return 0;
}
float areaTri (int base, float alpha1, float alpha2){
float area = pow(base, 2) * (sin(alpha1) * sin(alpha2)/sin(alpha1 +alpha2));
return area;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

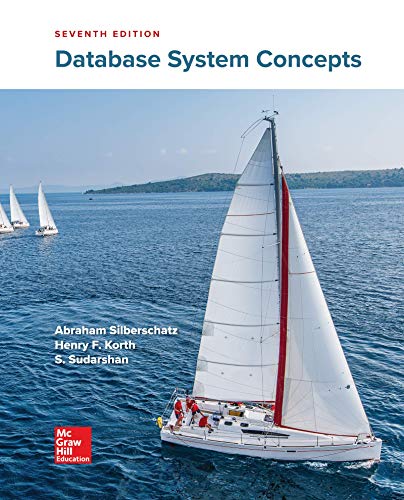
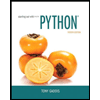
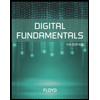
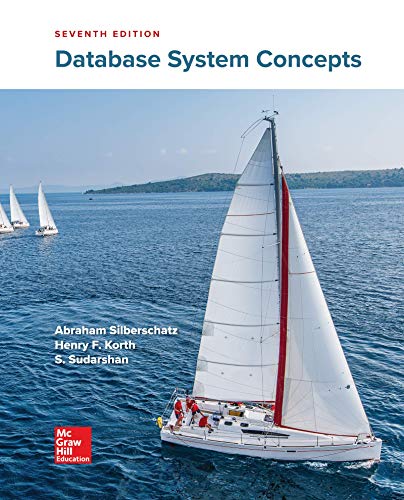
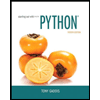
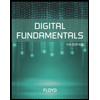
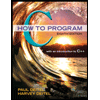
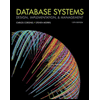
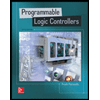