I am unable to get the same output, The powerball number has to be align with the ticket number as well as the drawing along with the powerball random number as shown on the images. Kindey, would you please test all my code? Thank you. // Lotto Program #include #include #include #include using namespace std; // Functions declaration void printMenu(); int getSelect(int&); bool isUnique(int[], int, int); void printArray(int[], int); void selection2_output(int ticket[], int); //creating a fixed winner drawing int drawing[] = { 56,34,1,8,12 }; int main() { int sel; printMenu(); getSelect(sel); return 0; } // Functions implementation // Menu function. void printMenu() { cout << "\tWelcome to the Lottery\t\n"; cout << " ---------------------------------\n"; cout << " 1. Quick Pick\n"; cout << " 2. Pick Numbers\n"; cout << " 3. Exit\n"; } //for selection1 output void selection1_output() { //random 5 number generation int r[5]; for (int i = 0; i < 5; i++) { r[i] = 1 + (rand() % 69); } //Powerball generation cout << setw(2) << left << "Player's Ticket: "; for (int i = 0; i < 5; i++) { cout << r[i] << " "; } int pw[1]; for (int i = 0; i < 1; i++) { pw[i] = 1 + (rand() % 26); } cout << " "; for (int i = 0; i < 1; i++) { cout<< setw(5) << right << pw[i]; } //cout << setw(3) << right << pw[i]; //cout << "\n"; cout << setw(2) << right << "\nDrawing: " ; for (int i = 0; i < 5; i++) { cout << drawing[i] << " " ; } for (int i = 0; i < 1; i++) { pw[i] = 1 + (rand() % 26); } cout << " "; for (int i = 0; i < 1; i++) { cout << setw(6) << right << pw[i]; } //comparing to check the number of common numbers int same = 0; for (int i = 0; i < 5; i++) { for (int j = 0; j < 5; j++) { if (r[j] == drawing[i]) same++; } } //if all numbers are common its a winner if (same == 5) cout << "\nYou Won the grand prize\n"; else cout << "\nBetter luck next time!\n"; } //for selection2 void selection2_output(int ticket[]) { cout << "Drawings : "; for (int i = 0; i < 5; i++) { cout << setw(4) << right << drawing[i] << " "; } int same = 0; //comparing to check the number of common numbers for (int i = 0; i < 5; i++) { for (int j = 0; j < 5; j++) { if (ticket[j] == drawing[i]) same++; } } //if all numbers are common its a winner if (same == 5) cout << "\nYou Won the grand prize\n"; else cout << "\nBetter luck next time\n"; } // getSelection function int getSelect(int& sel) { int count = 0; int ticket[5]; //creating a fixed cout << " Selection: "; cin >> sel; // input validation. while (sel == 3) { cout << "Program ending..." << endl; return 0; } while (true) { while (sel <= 0 || sel > 3) { cout << " Please your selction must be between 1 and 3. Try again.\n"; return 0; } //sel = getSelect(sel); while (sel == 1) { selection1_output(); break; } while (sel == 2) { while (count < 5) { cout << "Enter a unique number (1 - 69): "; cin >> ticket[count]; while (ticket[count] < 1 || ticket[count] > 69 || !isUnique(ticket, ticket[count], count)) { cout << "Try again.\n"; cout << "Enter a unique number (1 - 69): "; cin >> ticket[count]; break; } count++; cout << endl; } isUnique(ticket, ticket[count], count); int pwball = 0; for (int i = 0; i < 1; i++) { while (pwball < 26) { cout << "\n"; cout << "Enter the powerball number (1 - 26): "; cin >> pwball; break; } } cout << "Player Ticket: ", printArray(ticket, count), cout << pwball; // powerball selection2_output(ticket); break; } return sel; } } // isUnique Function // This function determines if there is unique number // in the array. bool isUnique(int ticket[], int n, int count) { for (int i = 0; i < count; i++) { if (ticket[i] == n) { return false; } } return true; } // printArray Function. void printArray(int ticket[], int count) { for (int i = 0; i < count; i++) { cout << setw(4) << right << ticket[i] << " "; } cout << endl; }
I am unable to get the same output, The powerball number has to be align with the ticket number as well as the drawing along with the powerball random number as shown on the images.
Kindey, would you please test all my code?
Thank you.
// Lotto Program
#include <ctime>
#include<iomanip>
#include <cstdlib>
#include<iostream>
using namespace std;
// Functions declaration
void printMenu();
int getSelect(int&);
bool isUnique(int[], int, int);
void printArray(int[], int);
void selection2_output(int ticket[], int);
//creating a fixed winner drawing
int drawing[] = { 56,34,1,8,12 };
int main()
{
int sel;
printMenu();
getSelect(sel);
return 0;
}
// Functions implementation
// Menu function.
void printMenu()
{
cout << "\tWelcome to the Lottery\t\n";
cout << " ---------------------------------\n";
cout << " 1. Quick Pick\n";
cout << " 2. Pick Numbers\n";
cout << " 3. Exit\n";
}
//for selection1 output
void selection1_output()
{
//random 5 number generation
int r[5];
for (int i = 0; i < 5; i++)
{
r[i] = 1 + (rand() % 69);
}
//Powerball generation
cout << setw(2) << left << "Player's Ticket: ";
for (int i = 0; i < 5; i++)
{
cout << r[i] << " ";
}
int pw[1];
for (int i = 0; i < 1; i++)
{
pw[i] = 1 + (rand() % 26);
}
cout << " ";
for (int i = 0; i < 1; i++)
{
cout<< setw(5) << right << pw[i];
}
//cout << setw(3) << right << pw[i];
//cout << "\n";
cout << setw(2) << right << "\nDrawing: " ;
for (int i = 0; i < 5; i++)
{
cout << drawing[i] << " " ;
}
for (int i = 0; i < 1; i++)
{
pw[i] = 1 + (rand() % 26);
}
cout << " ";
for (int i = 0; i < 1; i++)
{
cout << setw(6) << right << pw[i];
}
//comparing to check the number of common numbers
int same = 0;
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 5; j++)
{
if (r[j] == drawing[i])
same++;
}
}
//if all numbers are common its a winner
if (same == 5)
cout << "\nYou Won the grand prize\n";
else
cout << "\nBetter luck next time!\n";
}
//for selection2
void selection2_output(int ticket[])
{
cout << "Drawings : ";
for (int i = 0; i < 5; i++)
{
cout << setw(4) << right << drawing[i] << " ";
}
int same = 0;
//comparing to check the number of common numbers
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 5; j++)
{
if (ticket[j] == drawing[i])
same++;
}
}
//if all numbers are common its a winner
if (same == 5)
cout << "\nYou Won the grand prize\n";
else
cout << "\nBetter luck next time\n";
}
// getSelection function
int getSelect(int& sel)
{
int count = 0;
int ticket[5];
//creating a fixed
cout << " Selection: ";
cin >> sel;
// input validation.
while (sel == 3)
{
cout << "Program ending..." << endl;
return 0;
}
while (true)
{
while (sel <= 0 || sel > 3)
{
cout << " Please your selction must be between 1 and 3. Try again.\n";
return 0;
}
//sel = getSelect(sel);
while (sel == 1)
{
selection1_output();
break;
}
while (sel == 2)
{
while (count < 5)
{
cout << "Enter a unique number (1 - 69): ";
cin >> ticket[count];
while (ticket[count] < 1 || ticket[count] > 69 || !isUnique(ticket, ticket[count], count))
{
cout << "Try again.\n";
cout << "Enter a unique number (1 - 69): ";
cin >> ticket[count];
break;
}
count++;
cout << endl;
}
isUnique(ticket, ticket[count], count);
int pwball = 0;
for (int i = 0; i < 1; i++)
{
while (pwball < 26)
{
cout << "\n";
cout << "Enter the powerball number (1 - 26): ";
cin >> pwball;
break;
}
}
cout << "Player Ticket: ",
printArray(ticket, count),
cout << pwball;
// powerball
selection2_output(ticket);
break;
}
return sel;
}
}
// isUnique Function
// This function determines if there is unique number
// in the array.
bool isUnique(int ticket[], int n, int count)
{
for (int i = 0; i < count; i++)
{
if (ticket[i] == n)
{
return false;
}
}
return true;
}
// printArray Function.
void printArray(int ticket[], int count)
{
for (int i = 0; i < count; i++)
{
cout << setw(4) << right << ticket[i] << " ";
}
cout << endl;
}



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

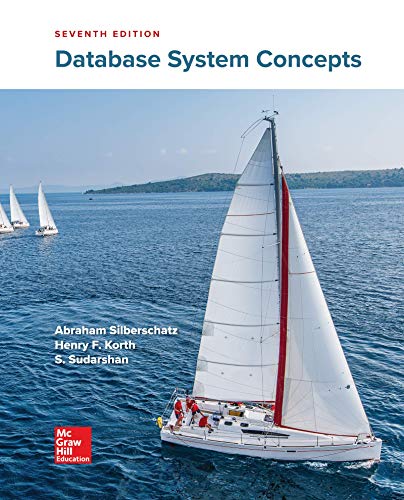
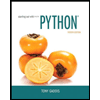
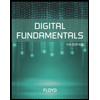
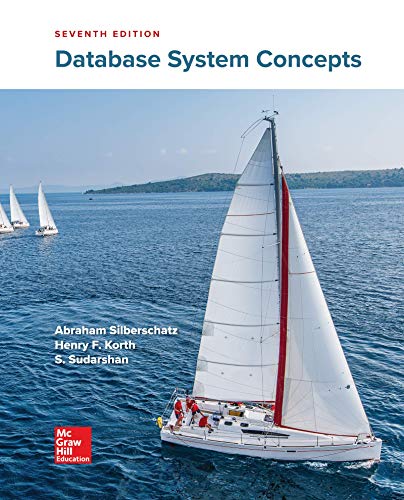
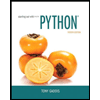
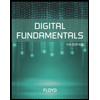
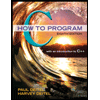
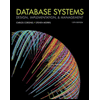
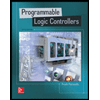