I am trying to solve a coding problem where I need to first push the elements of the array into a stack and then print the minimum in the stack at each pop until the stack becomes empty. Here is some Python code that solves this problem. Please explain to me the algorithm in this code step-by-step. Especially, tell me why stack reversal is important here, and why are we pushing the last element of the reversed stack back into the original stack. Python Code: def _push(a,n): stack = [] #iterating over the array elements and pushing them into the stack. for i in a: stack.append(i) return stack #Function to print minimum value in stack each time while popping. def _getMinAtPop(stack): reverseStack = [] #storing the stack elements in reverse order in reverseStack. while len(stack): reverseStack.append( stack.pop() ) #now stack will be used as minStack: stack.append( reverseStack.pop() ) while len(reverseStack): #comparing the top element of original stack with top element of #reverseStack and pushing the minimum value into stack. if stack[-1] < reverseStack[-1]: stack.append( stack[-1] ) reverseStack.pop() else: stack.append(reverseStack.pop()) #printing all the elements in stack one by one. while len(stack): print(stack.pop(),end=' ') s = [1, 6, 43, 1, 2, 0, 5] _push(s, 5) _getMinAtPop(s)
I am trying to solve a coding problem where I need to first push the elements of the array into a stack and then print the minimum in the stack at each pop until the stack becomes empty. Here is some Python code that solves this problem.
Please explain to me the
Python Code:
def _push(a,n):
stack = []
#iterating over the array elements and pushing them into the stack.
for i in a:
stack.append(i)
return stack
#Function to print minimum value in stack each time while popping.
def _getMinAtPop(stack):
reverseStack = []
#storing the stack elements in reverse order in reverseStack.
while len(stack):
reverseStack.append( stack.pop() )
#now stack will be used as minStack:
stack.append( reverseStack.pop() )
while len(reverseStack):
#comparing the top element of original stack with top element of
#reverseStack and pushing the minimum value into stack.
if stack[-1] < reverseStack[-1]:
stack.append( stack[-1] )
reverseStack.pop()
else:
stack.append(reverseStack.pop())
#printing all the elements in stack one by one.
while len(stack):
print(stack.pop(),end=' ')
s = [1, 6, 43, 1, 2, 0, 5]
_push(s, 5)
_getMinAtPop(s)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

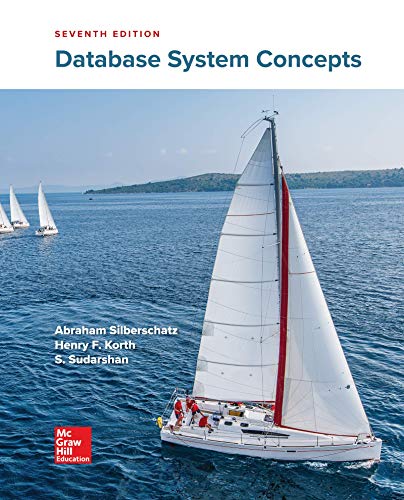
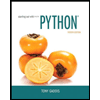
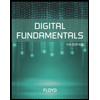
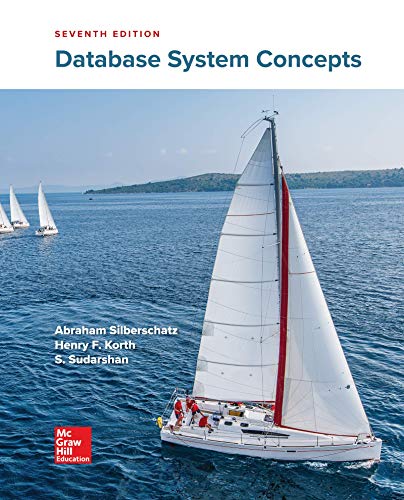
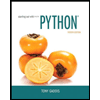
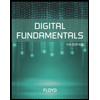
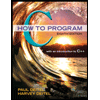
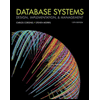
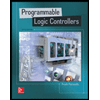