i am having problems making my program works. the following is what I have to do and underneath it is my program which I cant get it to work. i am not sure what my problem is. if you can figure it out that would be great thanks!! Write a C++ program that takes a date in the following format: December 24th, 2021 and extracts the day, month, and year as 3 integers. Your program should have and use at least the following functions: (a) Write a function getDate that prompts a user to enter a date as a string and returns it. (b) Write a function extract that takes as its parameter a date and returns the day, month, and year as 3 integers. (Hint: you can use the function isdigit(c) that returns true if c is a digit character and false otherwise.) (c) Write a function convertDigits that takes as its parameter a string of digits and converts it to an int. (Hint: to convert a digit character to a digit number use static cast (’d’) - static cast(’0’) where d is 0, 1, ..., 9) (d) Write a function convertMonths that takes as its parameter a month as a string and returns a corresponding number from 1 to 12 (1 for January, . . . , 12 for December). Write a program that repeatedly prompts the user to enter the date in the previous format. The program should then output it as in the examples below.
i am having problems making my program works. the following is what I have to do and underneath it is my program which I cant get it to work. i am not sure what my problem is. if you can figure it out that would be great thanks!!
Write a C++ program that takes a date in the following format: December 24th, 2021 and extracts the day, month, and year as 3 integers. Your program should have and use at least the following functions:
(a) Write a function getDate that prompts a user to enter a date as a string and returns it.
(b) Write a function extract that takes as its parameter a date and returns the day, month, and year as 3 integers. (Hint: you can use the function isdigit(c) that returns true if c is a digit character and false otherwise.)
(c) Write a function convertDigits that takes as its parameter a string of digits and converts it to an int. (Hint: to convert a digit character to a digit number use static cast (’d’) - static cast(’0’) where d is 0, 1, ..., 9)
(d) Write a function convertMonths that takes as its parameter a month as a string and returns a corresponding number from 1 to 12 (1 for January, . . . , 12 for December).
Write a program that repeatedly prompts the user to enter the date in the previous format.
The program should then output it as in the examples below.
For example,
Enter a date -- December 24th, 2021
24/12/2021
Try again (Y/N) -- Y
Enter a date -- February 28th, 2021
28/2/2021
Try again (Y/N) -- Y
Enter a date -- February 2nd, 2021
2/2/2021
Try again (Y/N) -- N
This is my program:
#include <iostream>
#include <string>
//Function prototypes
void getDate();
void extractDate(int&);
int convertDigits(string);
void convertMonth(string, int&);
int main(){
string date;
int day, month, year;
date = getDate();
cout << " " << day << "/" << month << "" << year << "\n";
return 0;
}
void getDate()
{
string date;
cout << "Enter any date -- ";
getline(cin,date);
extractDate(date);
return date;
}
void extractDate(int& date)
{
int y, z;
string mdn
y = date.find(" ");
mdn = date.subtr(0, y);
convertMonth(mdn, month);
z = date.find(" ", y+1);
mdn = date.substr(y+1, z-3);
day = convertDigits(mdn);
mdn = date.substr(y+1, date.length());
year = convertDigits(mdn);
}
int convertDigits(string myDate)
{
int n;
int sum = 0;
n = pow(10, myDate.length()-1);
for (int i = 0; i<myDate.length(); i++)
{
sum = sum+(myDate[i]-48)*n;
n=n/10;
}
return sum;
}
void convertMonth(string month, int& Num)
{
if (month == January){
Num = 1;
}
else if(month == February){
Num = 2;
}
else if (month == March){
Num = 3;
}
else if (month == April){
Num = 4;
}
else if (month == May){
Num = 5;
}
else if (month == June){
Num = 6;
}
else if (month == July){
Num = 7;
}
else if (month == August){
Num = 8;
}
else if (month == September){
Num = 9;
}
else if (month == October){
Num = 10;
}
else if (month == November){
Num = 11;
}
else if (month == december){
Num = 12;
}
else{
cout << "invalid \n";
}
}

Step by step
Solved in 3 steps with 1 images

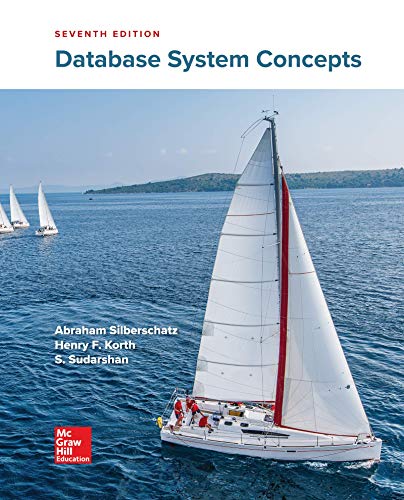
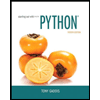
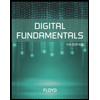
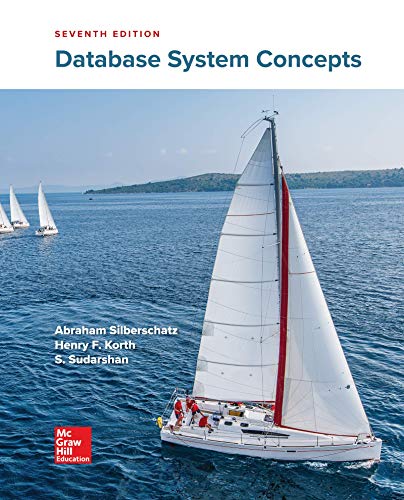
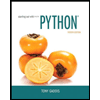
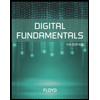
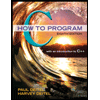
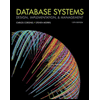
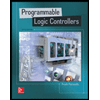