I am having a problem with my static casting. I keep getting only one response no matter what values I use in the program. It's as the bottom half of my program is not executing. I cannot figure out what I am doing incorrectly. I am sure it is obvious, but after looking for two days I don't see it. /*Program: CL8_Zick_UrsulaAuthor: Ursula ZickDate: 02/13/2019Purpose: Calculate the percentage of calories from fat*/ #include<iostream>#include<iomanip>using namespace std; int main(){// Variantsint fatGrams; // Total number of calories in one gram of fatint fatCalories; // Total amount of calories from fatint totalCalories; // Total number of calories from fatint fatPercentage; // Total fat percentage from calories cout << "Enter the requested information to determine the percentage of fat in food item; \n\n"; // Get the total calories from food itemcout << "Enter the total calories: ";cin >> totalCalories; // Get the total fat grams from food itemcout << "Enter the total fat grams: ";cin >> fatGrams; // Convert the fat calories to fat gramsfatCalories = fatGrams * 9;// Determine if fat gram is less than zeroif (totalCalories < 0 || fatGrams < 0)cout << " Total calories or fat grams can not be less than zero ";else{if (fatCalories > totalCalories)cout << "\nInvalid - There are more calories in fatGrams \n"<< "grams of fat than you entered for total calories: ";}// Static cast on the fat percentagefatPercentage = static_cast <double> (fatCalories) / totalCalories; // Calculate fat percentageif (fatPercentage *= 100)cout << "Percentage of fat: ";else{if (fatPercentage < 30.0)cout << "\nThat food is low in fat: \n\n";else cout << "\nThat food is not low in fat:";}return 0;}
I am having a problem with my static casting. I keep getting only one response no matter what values I use in the
/*
Program: CL8_Zick_Ursula
Author: Ursula Zick
Date: 02/13/2019
Purpose: Calculate the percentage of calories from fat
*/
#include<iostream>
#include<iomanip>
using namespace std;
int main()
{
// Variants
int fatGrams; // Total number of calories in one gram of fat
int fatCalories; // Total amount of calories from fat
int totalCalories; // Total number of calories from fat
int fatPercentage; // Total fat percentage from calories
cout << "Enter the requested information to determine the percentage of fat in food item; \n\n";
// Get the total calories from food item
cout << "Enter the total calories: ";
cin >> totalCalories;
// Get the total fat grams from food item
cout << "Enter the total fat grams: ";
cin >> fatGrams;
// Convert the fat calories to fat grams
fatCalories = fatGrams * 9;
// Determine if fat gram is less than zero
if (totalCalories < 0 || fatGrams < 0)
cout << " Total calories or fat grams can not be less than zero ";
else
{
if (fatCalories > totalCalories)
cout << "\nInvalid - There are more calories in fatGrams \n"
<< "grams of fat than you entered for total calories: ";
}
// Static cast on the fat percentage
fatPercentage = static_cast <double> (fatCalories) / totalCalories;
// Calculate fat percentage
if (fatPercentage *= 100)
cout << "Percentage of fat: ";
else
{
if (fatPercentage < 30.0)
cout << "\nThat food is low in fat: \n\n";
else
cout << "\nThat food is not low in fat:";
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

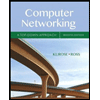
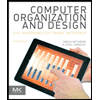
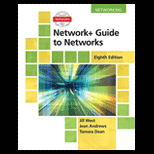
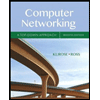
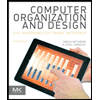
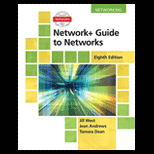
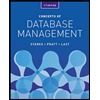
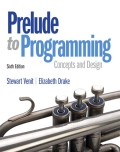
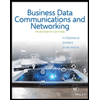