I am doint a homework problem for class in which I am supposed to: Input the following names in the array in a stack (as shown in the class lecture) and print out the names in reverse order char* names[] = {"Aaron", "Alizia", "Andrew", "Anthony", "Ashleigh"}; Here is my code and the output. I can't figure out what is wrong with my code. Can you let me know what is causing the output? #include #include #include #include typedef struct { char* ar; int usedlength; int totalLength; }stack; void stackConstruct(stack* s) { s->usedlength = 0; s->totalLength = 6; s->ar = malloc(sizeof(char*) * 6); //s->ar = {"Aaron", "Andrew", "Alizia", "Anthony", "Ashleigh"}; } void stackDestruct(stack* s) { free(s->ar); } void stackPush(stack* s, char val) { if (s->usedlength == s->totalLength) { s->totalLength *= 2; s->ar = realloc(s->ar, sizeof(int) * s->totalLength); //realloc does malloc the size *2 then copy it; } s->ar[s->usedlength] = val; s->usedlength++; } void stackPop(stack* s) { assert(s->usedlength != 0); s->usedlength--; } int stackTop(stack* s) { assert(s->usedlength != 0); return s->ar[s->usedlength - 1]; } int isEmpty(stack* s) { return s->usedlength == 0; } int main() { stack n; stackConstruct(&n); for (int i = 0; i < 5; i++) { switch (i) { case 0: { stackPush(&n, "Aaron"); break; } case 1: { stackPush(&n, "Alizia"); break; } case 2: { stackPush(&n, "Andrew"); break; } case 3: { stackPush(&n, "Anthony"); break; } case 4: { stackPush(&n, "Ashleigh"); break; } default: { printf("Error\n"); } } } //printf("This is just a test: %s\n", n.ar[0]); while (!isEmpty(&n)) { char c[] = {stackTop(&n)}; printf("%s ", c); stackPop(&n); } stackDestruct(&n); return 0; }
I am doint a homework problem for class in which I am supposed to: Input the following names in the array in a stack (as shown in the class lecture) and print out the names in reverse order char* names[] = {"Aaron", "Alizia", "Andrew", "Anthony", "Ashleigh"}; Here is my code and the output. I can't figure out what is wrong with my code. Can you let me know what is causing the output? #include #include #include #include typedef struct { char* ar; int usedlength; int totalLength; }stack; void stackConstruct(stack* s) { s->usedlength = 0; s->totalLength = 6; s->ar = malloc(sizeof(char*) * 6); //s->ar = {"Aaron", "Andrew", "Alizia", "Anthony", "Ashleigh"}; } void stackDestruct(stack* s) { free(s->ar); } void stackPush(stack* s, char val) { if (s->usedlength == s->totalLength) { s->totalLength *= 2; s->ar = realloc(s->ar, sizeof(int) * s->totalLength); //realloc does malloc the size *2 then copy it; } s->ar[s->usedlength] = val; s->usedlength++; } void stackPop(stack* s) { assert(s->usedlength != 0); s->usedlength--; } int stackTop(stack* s) { assert(s->usedlength != 0); return s->ar[s->usedlength - 1]; } int isEmpty(stack* s) { return s->usedlength == 0; } int main() { stack n; stackConstruct(&n); for (int i = 0; i < 5; i++) { switch (i) { case 0: { stackPush(&n, "Aaron"); break; } case 1: { stackPush(&n, "Alizia"); break; } case 2: { stackPush(&n, "Andrew"); break; } case 3: { stackPush(&n, "Anthony"); break; } case 4: { stackPush(&n, "Ashleigh"); break; } default: { printf("Error\n"); } } } //printf("This is just a test: %s\n", n.ar[0]); while (!isEmpty(&n)) { char c[] = {stackTop(&n)}; printf("%s ", c); stackPop(&n); } stackDestruct(&n); return 0; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I am doint a homework problem for class in which I am supposed to:
Input the following names in the array in a stack (as shown in the class lecture) and print out the names in reverse order
char* names[] = {"Aaron", "Alizia", "Andrew", "Anthony", "Ashleigh"};
Here is my code and the output. I can't figure out what is wrong with my code. Can you let me know what is causing the output?
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<assert.h>
typedef struct {
char* ar;
int usedlength;
int totalLength;
}stack;
void stackConstruct(stack* s) {
s->usedlength = 0;
s->totalLength = 6;
s->ar = malloc(sizeof(char*) * 6);
//s->ar = {"Aaron", "Andrew", "Alizia", "Anthony", "Ashleigh"};
}
void stackDestruct(stack* s) {
free(s->ar);
}
void stackPush(stack* s, char val) {
if (s->usedlength == s->totalLength) {
s->totalLength *= 2;
s->ar = realloc(s->ar, sizeof(int) * s->totalLength); //realloc does malloc the size *2 then copy it;
}
s->ar[s->usedlength] = val;
s->usedlength++;
}
void stackPop(stack* s) {
assert(s->usedlength != 0);
s->usedlength--;
}
int stackTop(stack* s) {
assert(s->usedlength != 0);
return s->ar[s->usedlength - 1];
}
int isEmpty(stack* s) {
return s->usedlength == 0;
}
int main() {
stack n;
stackConstruct(&n);
for (int i = 0; i < 5; i++) {
switch (i) {
case 0: {
stackPush(&n, "Aaron");
break;
}
case 1: {
stackPush(&n, "Alizia");
break;
}
case 2: {
stackPush(&n, "Andrew");
break;
}
case 3: {
stackPush(&n, "Anthony");
break;
}
case 4: {
stackPush(&n, "Ashleigh");
break;
}
default: {
printf("Error\n");
}
}
}
//printf("This is just a test: %s\n", n.ar[0]);
while (!isEmpty(&n)) {
char c[] = {stackTop(&n)};
printf("%s ", c);
stackPop(&n);
}
stackDestruct(&n);
return 0;
}

Transcribed Image Text:```plaintext
La┘-╩3┐ÿÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍ-╩3┐¿ÍÍÍÍÍÍ
•─╖3 á╓ÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍÍİ-╩3┐¿
C:\Users\caleb\OneDrive\Desktop\Programing Languages\Classwork10\x64\Debug\Classwork10.exe (process 1052) exited with code 0.
To automatically close the console when debugging stops, enable Tools->Options->Debugging->Automatically close the console when debugging stops.
Press any key to close this window . . .
```
**Description**
This image shows a command-line console, likely from the Microsoft Visual Studio debugger. The first few lines display non-standard ASCII characters, possibly resulting from an error or data corruption in program output. Below this, the file path and process information are displayed, indicating that the executable `Classwork10.exe` has exited with code 0, which typically signifies a successful execution without errors.
Additional instructions are provided to automatically close the console when debugging stops. This can be done by navigating to `Tools->Options->Debugging->Automatically close the console when debugging stops` in Visual Studio. The message ends by indicating that the user can press any key to close the console window.
There are no graphs or diagrams present in the image.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
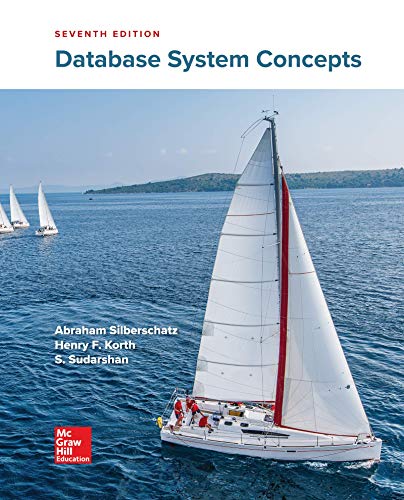
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
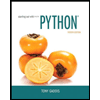
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
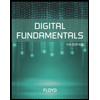
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
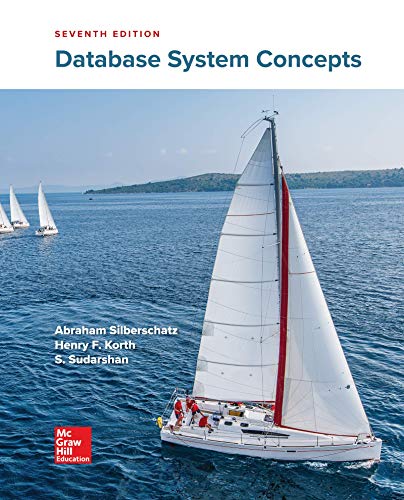
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
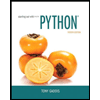
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
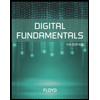
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
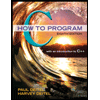
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
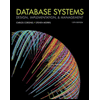
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
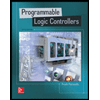
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education