I already have this python code for json. I just need to make it display the data in tabular format, including an additional column showing each student’s average to the right of that student’s three exam grades and an additional row showing the class average on each exam below that exam’s column. There is also a example pic of how it should look like. Code below: import json def main(): n = int(input("Enter the number of students: ")) data=[] for i in range(n): first = input("\nEnter First Name of Student "+str(i+1)+": ") last = input("Enter Last Name of Student "+str(i+1)+": ") te1 = int(input("Enter Te_st 1 Grade of Student "+str(i+1)+": ")) te2 = int(input("Enter Te_st 2 Grade of Student "+str(i+1)+": ")) te3 = int(input("Enter Te_st 3 Grade of Student "+str(i+1)+": ")) info={} info['first_name']=first info['last_name']=last info['ex_am1']=te1 info['ex_am2']=te2 info['ex_am3']=te3 data.append(info) gradebook_dict ={'students':data} with open("grades.json",'w') as _file: json.dump(gradebook_dict ,_file,indent=4) print("\nWriting Done.\n") with open("grades.json",'r') as _file: data = (json.load(_file))['students'] for i in data: print(i) main()
I already have this python code for json. I just need to make it display the data in tabular format, including an additional column showing each student’s average to the right of that student’s three exam grades and an additional row showing the class average on each exam below that exam’s column. There is also a example pic of how it should look like.
Code below:


Code:-
import json
def main():
n = int(input("Enter the number of students: "))
data=[]
for i in range(n):
first = input("\nEnter First Name of Student "+str(i+1)+": ")
last = input("Enter Last Name of Student "+str(i+1)+": ")
te1 = int(input("Enter Te_st 1 Grade of Student "+str(i+1)+": "))
te2 = int(input("Enter Te_st 2 Grade of Student "+str(i+1)+": "))
te3 = int(input("Enter Te_st 3 Grade of Student "+str(i+1)+": "))
info={}
info['first_name']=first
info['last_name']=last
info['ex_am1']=te1
info['ex_am2']=te2
info['ex_am3']=te3
data.append(info)
gradebook_dict ={'students':data}
with open("grades.json",'w') as _file:
json.dump(gradebook_dict ,_file,indent=4)
print("\nWriting Done.\n")
with open("grades.json",'r') as _file:
data = (json.load(_file))['students']
print(f'{"First Name":>15} {"Last Name":>15} {"test 1":>6} {"test 2":>6} {"test 3":>6} {"average":>7}')
totalTest1=0
totalTest2=0
totalTest3=0
count=0
for i in data:
t1=i['ex_am1']
t2=i['ex_am2']
t3=i['ex_am3']
totalTest1+=t1
totalTest2+=t2
totalTest3+=t3
count+=1
fname=i['first_name']
lname=i['last_name']
avg=(t1+t2+t3)/3
print(f"{fname:>15} {lname:>15} {t1:>6d} {t2:>6d} {t3:>6d} {avg:>7.2f}")
print("-"*60)
print(f"{'Test average':<32} {totalTest1/count:>6.2f} {totalTest2/count:>6.2f} {totalTest3/count:>6.2f}")
main()
Step by step
Solved in 2 steps with 3 images

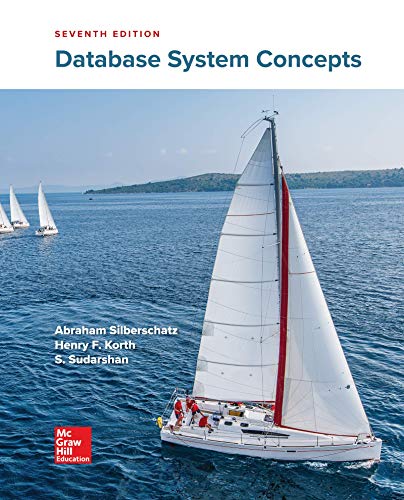
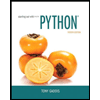
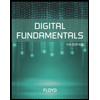
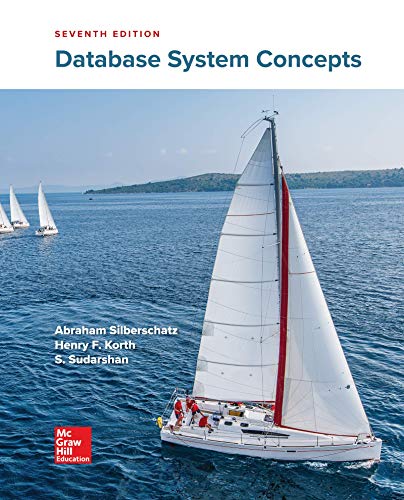
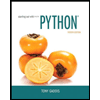
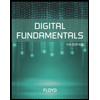
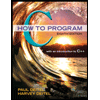
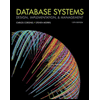
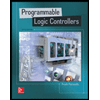