I already have the class working. I just need to print it sorted by the length of name, and by GPA from class collections and arrays. My current code is as follows.
I already have the class working. I just need to print it sorted by the length of name, and by GPA from class collections and arrays. My current code is as follows.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
I already have the class working. I just need to print it sorted by the length of name, and by GPA from class collections and arrays. My current code is as follows.

Transcribed Image Text:The image contains a segment of Java code that demonstrates sorting a list of `Student` objects based on two different criteria: name length and GPA. The code is structured in a block with comments and is divided into several key parts:
1. **Copying and Sorting by Name Length (Ascending):**
- The code first displays a message indicating that the students are being sorted by name length in ascending order.
- It uses a `for` loop to iterate over the `copy1` list and prints out each `Student` object.
2. **Copying the List and Sorting by GPA (Descending):**
- A new copy of the `Student` list is created as `copy2`.
- The list is then sorted by GPA in descending order using the `Collections.sort()` method with a custom `Comparator<Student>`:
- If the GPA of `s1` is greater than `s2`, it returns -1.
- If the GPA of `s1` is less than `s2`, it returns 1.
- Otherwise, it returns 0 for equal GPAs.
- A message is printed to indicate the sorted order.
3. **Copying to an Array and Sorting by Name Length (Ascending):**
- An array `array1` of `Student` is created with the same size as the original list.
- The list is converted to an array using `list.toArray(array)`.
- The array is sorted by name length using `Arrays.sort()` with a custom `Comparator<Student>`:
- It compares the lengths of the names and sorts them in ascending order.
- It then iterates over the array and prints out each `Student` object.
This code is a practical example of using Java collections and arrays to manipulate and sort data, illustrating the implementation of custom comparator logic for sorting purposes.
![```java
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedList;
public class Student {
private String name;
private double gpa;
public Student(String name, double gpa) {
this.name = name;
this.gpa = gpa;
}
public String getName() {
return name;
}
public double getGpa() {
return gpa;
}
public String toString() {
return "(name," + name + " Gpa: " + gpa + ")";
}
public static void main(String[] args) {
LinkedList<Student> list = new LinkedList<Student>();
list.add(new Student("Kyle", 3.4));
list.add(new Student("Dog", 4.0));
list.add(new Student("Jeff", 3.97));
list.add(new Student("Manny", 1.9));
list.add(new Student("Wog", 5.1));
// taking a copy of list for sorting, so that sorting will not affect the initial list
LinkedList<Student> copy1 = new LinkedList<Student>(list);
// with a custom Comparator, sorting copy1 list using compare method by name length
Collections.sort(copy1, new Comparator<Student>() {
public int compare(Student s1, Student s2) {
// this method will return a negative value if s1 name length
// is before s2, positive value if s1 needs to come after,
// zero if they have same order.
return s1.getName().length() - s2.getName().length();
}
});
for (Student s : copy1) {
System.out.println(s);
}
}
}
```
### Explanation:
This Java program defines a `Student` class with two private fields, `name` and `gpa`, and provides methods to access these fields (`getName` and `getGpa`). The `toString` method returns a string representation of the `Student` object.
In the `main` method, a `LinkedList` of `Student` objects is created and populated with students having different names and GPAs. A copy of this list (`copy1`) is created for sorting purposes, ensuring the original list remains unaffected.
The program utilizes the `Collections.sort` method with a custom `Comparator` to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4d68e090-7e11-4ab8-a19a-f8ec202a32a2%2F96537b95-9c2b-46cb-9c0e-9b9430d721e6%2Fwpdy31_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```java
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedList;
public class Student {
private String name;
private double gpa;
public Student(String name, double gpa) {
this.name = name;
this.gpa = gpa;
}
public String getName() {
return name;
}
public double getGpa() {
return gpa;
}
public String toString() {
return "(name," + name + " Gpa: " + gpa + ")";
}
public static void main(String[] args) {
LinkedList<Student> list = new LinkedList<Student>();
list.add(new Student("Kyle", 3.4));
list.add(new Student("Dog", 4.0));
list.add(new Student("Jeff", 3.97));
list.add(new Student("Manny", 1.9));
list.add(new Student("Wog", 5.1));
// taking a copy of list for sorting, so that sorting will not affect the initial list
LinkedList<Student> copy1 = new LinkedList<Student>(list);
// with a custom Comparator, sorting copy1 list using compare method by name length
Collections.sort(copy1, new Comparator<Student>() {
public int compare(Student s1, Student s2) {
// this method will return a negative value if s1 name length
// is before s2, positive value if s1 needs to come after,
// zero if they have same order.
return s1.getName().length() - s2.getName().length();
}
});
for (Student s : copy1) {
System.out.println(s);
}
}
}
```
### Explanation:
This Java program defines a `Student` class with two private fields, `name` and `gpa`, and provides methods to access these fields (`getName` and `getGpa`). The `toString` method returns a string representation of the `Student` object.
In the `main` method, a `LinkedList` of `Student` objects is created and populated with students having different names and GPAs. A copy of this list (`copy1`) is created for sorting purposes, ensuring the original list remains unaffected.
The program utilizes the `Collections.sort` method with a custom `Comparator` to
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
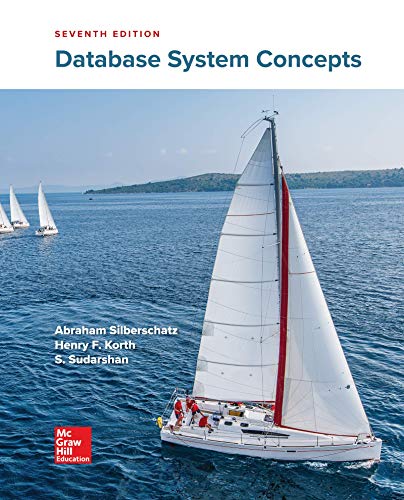
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
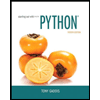
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
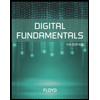
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
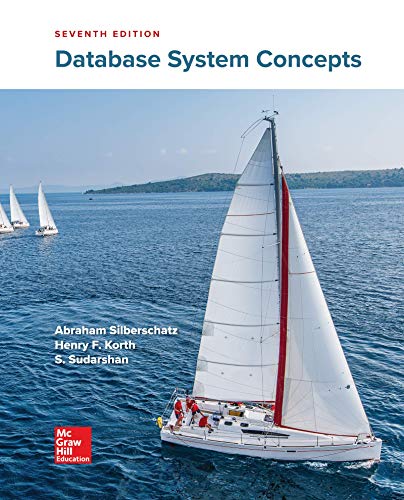
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
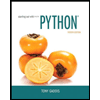
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
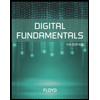
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
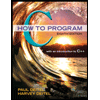
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
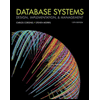
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
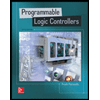
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education