HW07-1 Create an SD diagram that will show the interaction between Simulator, Field, and a single Fox and a single Rabbit, based on your observations of runtime behavior and an examination of the source code for Foxes v-2. [Assume your single Fox instance can possibly eat your single Rabbit!] Diagram should use at least one Opt block and at least one Alt block. [Could also consider nested blocks!].
HW07-1 Create an SD diagram that will show the interaction between Simulator, Field, and a single Fox and a single Rabbit, based on your observations of runtime behavior and an examination of the source code for Foxes v-2. [Assume your single Fox instance can possibly eat your single Rabbit!] Diagram should use at least one Opt block and at least one Alt block. [Could also consider nested blocks!].
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%

Transcribed Image Text:4 #
Simulator.java X
10 import java.util.Random;
6
7 public class Simulator
9
10
DH23GS 8901
11
12
13
14
15
16
170
18
1000
19
200
21
22
22
23
24
25
26
27
28
29
30
31
32
33
340
35
36
37
380
39
40
41
125.
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
610
62
63
64
65
66
67
680
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
private static final int DEFAULT_WIDTH = 120;
private static final int DEFAULT_DEPTH = 80;
85
86
870
88
89
90
91
02
private static final double FOX_CREATION_PROBABILITY = 0.02;
private static final double RABBIT_CREATION_PROBABILITY = 0.08;
private List<Animal> animals;
private Field field;
private int step;
private SimulatorView view;
public Simulator()
{this (DEFAULT_DEPTH, DEFAULT_WIDTH) ;
}
public Simulator(int depth, int width)
{
42
}
43
}
440 public void simulateOneStep()
{
step++;
// Provide space for newborn animals.
List<Animal> newAnimals = new ArrayList<>();
if(width <= 0 || depth <= 0) {
System.out.println("The dimensions must be greater than zero.");
System.out.println("Using default values.");
depth =DEFAULT_DEPTH;
DEFAULT_WIDTH; }
width =
animals = new ArrayList<>();
field = new Field (depth, width);
view = new SimulatorView(depth, width);
view.setColor (Rabbit.class, Color. ORANGE) ;
view.setColor(Fox.class, Color.BLUE);
reset();
}
public void runLongSimulation ()
{
simulate (4000);
}
public void simulate(int numSteps)
{
for(int step = 1; step <= numSteps && view.isViable(field); step++) {
simulateOneStep();
// Let all rabbits act.
for (Iterator<Animal> it = animals.iterator(); it.hasNext(); ) {
Animal animal = it.next();
animal.act(newAnimals);
if(! animal.isAlive()) {
it.remove();
}
}
animals.addAll(newAnimals);
view.showStatus(step, field);
}
public void reset()
{
step = 0;
animals.clear();
populate();
view.showStatus (step, field);
}
private void populate()
{
Random rand = Randomizer.getRandom();
field.clear();
for(int row = 0; row < field.getDepth(); row++) {
for(int col =
0; col < field.getWidth(); col++) {
}
}
if(rand.nextDouble() <= FOX_CREATION_PROBABILITY) {
Location location = new Location (row, col);
Fox fox = new Fox(true, field, location);
animals.add(fox);
}
else if(rand.nextDouble() <= RABBIT_CREATION_PROBABILITY) {
Location location = new Location (row, col);
Rabbit rabbit = new Rabbit(true, field, location);
animals.add(rabbit);
}
}
private void delay(int millisec)
{
try {
catch
}
Thread.sleep (millisec);
stodExcon
s
J Rabbit.java X
10 import java.util.List;
3 public class Rabbit extends Animal
4957WNTOSO LASSE
4 {
5
6
7
8
' 10 11 12 13 24 15 16 7 18 192021222324 25 26 27 28 29 30 31 323343536738394444243445467 48 495051234 55 56 57 58 59
100
14
17
40
41
430
470
452
550
58 }
private static final int BREEDING_AGE = 5;
private static final int MAX_AGE = 40;
private static final double BREEDING_PROBABILITY = 0.12;
private static final int MAX_LITTER_SIZE = 4;
Randomizer.getRandom();
private static final Random rand =
public Rabbit (boolean randomAge, Field field, Location location)
{
{
}
public void act (List<Animal> newRabbits)
}
super(field, location);
setAge(0);
if (randomAge) {
}
}
setAge(rand.nextInt (MAX_AGE));
incrementAge();
if(isAlive()) {
}
giveBirth(newRabbits);
}
private void giveBirth (List<Animal> newRabbits)
{
}
Location newLocation = getField().freeAdjacent Location (getLocatio
if(newLocation != null) {
setLocation (newLocation);
}
else {
}
setDead ();
}
}
protected int getBreeding Age() {
return BREEDING_AGE;
Field field
getField();
List<Location> free = field.getFreeAdjacent Locations (getLocation () );
int births = breed();
protected int getMaxAge() {
return MAX_AGE;
@Override
=
for(int b = 0; b < births && free.size() > 0; b++) {
Location loc = free.remove(0);
Rabbit young = new Rabbit(false, field, loc);
newRabbits.add(young);
OOPDA - Foxes_v3_copy/src/Fox.java - Eclipse IDE
protected double getBREEDING_PROBABILITY() {
return BREEDING_PROBABILITY;
protected int getMAX_LITTER_SIZE() {
return MAX_LITTER_SIZE;
Animal.java X
10 import java.util.List;
3456789OHATOONMANORA
4 public abstract class Animal
5 {
10
11
120
13
14
15
16
17
18
190
20
21
22
230
24
25
26
27
28
290
30
31
32
330
40
41
42
430
44
480
49
50
51
52
53
54
55
56
hneddoTRANSFEREHEERE
58
59
60
61
62
63
640
65
66
67
68
69
700
570 protected Field getField()
return field;
71
72
73
74
75
76
77
78
79
800
81
82
83
84
85
86
87
private boolean alive;
private Field field;
private Location location;
private int age;
private static final Random rand = Randomizer.getRandom();
public Animal(Field field, Location location)
{
alive = true;
this.field = field;
set Location (location);
age = 0;
}
88}
protected int getAge() {
return age;
}
protected void setAge(int newAge) {
age = newAge;
}
abstract public void act(List<Animal> newAnimals);
protected boolean is Alive ()
return alive;
{
}
protected void setDead()
{
alive = false;
if(location != null) {
}
{
}
protected Location getLocation()
return location;
}
protected void setLocation (Location newLocation)
{
if(location != null) {
}
}
field.clear(location);
location = null;
field = null;
}
}
field.clear(location);
}
location = newLocation;
field.place(this, newLocation);
abstract protected int getBreedingAge();
protected boolean canBreed() {
return getAge() >= getBreedingAge();
abstract protected int getMaxAge();
protected void incrementAge() {
setAge(getAge()+1);
if (getAge() > getMaxAge()) {
set Dead ();
}
abstract protected double getBREEDING_PROBABILITY();
abstract protected int getMAX_LITTER_SIZE();
}
protected int breed()
{
int births = 0;
if (canBreed() && rand.nextDouble() <= getBREEDING_PROBABILITY())
births = rand.nextInt (getMAX_LITTER_SIZE()) + 1;
}
return births;
Writable
Fox.java XJ Tester.java
{
56789
10
AAAAAAAAAN~~~~~~~~28
11
12
130
14
15
16
17
18
19
20
21
22
23
24
25
▲260
27
29
30
31
32
33
34
35
36
37
38
39
46 4 42 45 4 45 46 47 48 49 50 51 52 58
40
41
43
440
510
52
53
54
56
55
56
57
58
59
60
61
62
63
64
HTCORNER52
65
66
67
68
69
700
71
72
73
74
75
76
77
78
79
80
810
82
83
840
85
86
870
88
89
900
91
92
private static final int BREEDING_AGE = 15;
private static final int MAX_AGE = 150;
private static final double BREEDING_PROBABILITY = 0.08;
private static final int MAX_LITTER_SIZE = 2;
private static final int RABBIT_FOOD_VALUE = 9;
private static final Random rand = Randomizer.getRandom();
private int foodLevel;
public Fox (boolean randomAge, Field field, Location location)
{
super(field, location);
if (randomAge) {
setAge(rand.nextInt (MAX_AGE));
foodLevel = rand.nextInt (RABBIT_FOOD_VALUE);
}
else {
}
}
public void act(List<Animal> newFoxes)
{
setAge(0);
foodLevel = RABBIT_FOOD_VALUE;
incrementAge();
incrementHunger();
if(isAlive()) {
giveBirth(newFoxes);
Location newLocation = findFood ();
if (newLocation
null) {
newLocation = getField().freeAdjacent Location (get Location());
}
}
if(newLocation != null) {
}
set Location (newLocation);
}
else {
}
}
}
private void incrementHunger ()
{
foodLevel--
<=
if (foodLevel
setDead ();
}
}
private Location findFood ()
{
Field field = getField();
List<Location> adjacent = field.adjacent Locations (getLocation());
setDead ();
Iterator<Location> it = adjacent.iterator();
while(it.hasNext()) {
Location where = it.next();
Object animal = field.getObjectAt (where);
if(animal instanceof Rabbit) {
0) {
}
Rabbit rabbit = (Rabbit) animal;
if(rabbit.isAlive()) {
rabbit.setDead ();
}
}
return null;
}
private void giveBirth (List<Animal> newFoxes)
{
foodLevel = RABBIT_FOOD_VALUE;
return where;
Field field =
getField();
List<Location> free = field.getFreeAdjacent Locations (getLocation());
int births
= breed();
for(int b = 0; b < births && free.size() > 0; b++) {
Location loc = free.remove(0);
}
}
protected int get Breeding Age() {
return BREEDING_AGE;
Fox young = new Fox(false, field, loc);
newFoxes.add(young);
}
protected int getMaxAge() {
return MAX_AGE;
Smart Insert
}
protected double getBREEDING_PROBABILITY() {
return BREEDING_PROBABILITY;
protected int getMAX_LITTER_SIZE() {
return MAX_LITTER_SIZE;
89: 6:2671
a
m
M
![HW07-1 Create an SD diagram that will show the interaction between Simulator, Field, and a single Fox
and a single Rabbit, based on your observations of runtime behavior and an examination of the source
code for Foxes v-2. [Assume your single Fox instance can possibly eat your single Rabbit!] Diagram
should use at least one Opt block and at least one Alt block. [Could also consider nested blocks!].
Note: There may be 3-5 actors with timelines in your SD: AFox, ARabbit, theSimulator, and maybe ???
and ???. Try to show messages between the timelines. Example "setDead()-→"
Include a WORD document that describes your analysis for this, as well as the pdf for your diagram.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3199efa8-af35-43b1-95a6-3405e7d6b09b%2Fb1fe537e-1b49-43fe-a3d6-80993cee63fb%2Fxso7jq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:HW07-1 Create an SD diagram that will show the interaction between Simulator, Field, and a single Fox
and a single Rabbit, based on your observations of runtime behavior and an examination of the source
code for Foxes v-2. [Assume your single Fox instance can possibly eat your single Rabbit!] Diagram
should use at least one Opt block and at least one Alt block. [Could also consider nested blocks!].
Note: There may be 3-5 actors with timelines in your SD: AFox, ARabbit, theSimulator, and maybe ???
and ???. Try to show messages between the timelines. Example "setDead()-→"
Include a WORD document that describes your analysis for this, as well as the pdf for your diagram.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
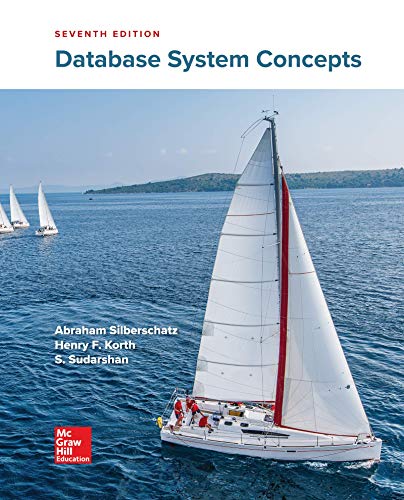
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
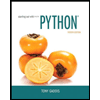
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
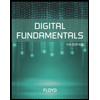
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
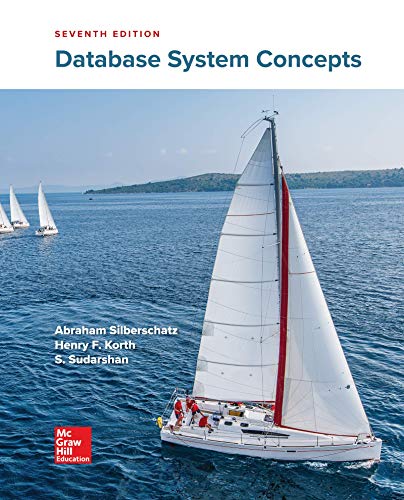
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
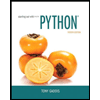
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
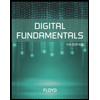
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
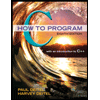
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
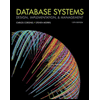
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
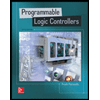
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education