How would I implement the sort()? For implementing a max heap, in Java?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How would I implement the sort()?
For implementing a max heap, in Java?
![```java
public Heapsort(int arr[])
{
int n = arr.length;
this.arr=new int[n];
for (int i = 0; i<n; i++)
this.arr[i]=arr[i];
}
public void sort()
{
int n = arr.length;
// Build heap (rearrange array)
int startIndex = (n / 2) - 1;
// fill statements
// One by one extract an element from heap
for (int i=n-1; i>=0; i--)
{
// Move current root to end
//fill
heapify(arr, n, i);
//fill
// call max heapify on the reduced heap
//fill
}
}
// To heapify a subtree rooted with node i which is
// an index in arr[]. n is size of heap
void heapify(int arr[], int n, int i)
{
int largest = i; // Initialize largest as root
int l = 2*i + 1; // left = 2*i + 1
int r = 2*i + 2; // right = 2*i + 2
// If left child is larger than root
if (l < n && arr[l] > arr[largest])
largest = l;
// If right child is larger than largest so far
if (r < n && arr[r] > arr[largest])
largest = r;
// If largest is not root
if (largest != i)
{
int swap = arr[i];
arr[i] = arr[largest];
arr[largest] = swap;
// Recursively heapify the affected sub-tree
heapify(arr, n, largest);
}
}
/* A utility function to save array of size n as a String */
public String toString()
{
String s=new String();
for (int i=0; i<arr.length; i++)
s=s + " " + arr[i];
return s;
}
```
**Explanation:**
This code defines a simple implementation of the Heap Sort algorithm in Java. Here’s a breakdown of the key components:
1. **Constructor `Heapsort`:**
- Initializes a new array `arr` from the input array.
2. **Method `sort`:**
-](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F346b36e0-a4d8-47d7-8bd1-1ad776cb1cf3%2Fd2025966-4e8f-4356-bc69-35bc58981208%2Fh5exqr_processed.png&w=3840&q=75)
Transcribed Image Text:```java
public Heapsort(int arr[])
{
int n = arr.length;
this.arr=new int[n];
for (int i = 0; i<n; i++)
this.arr[i]=arr[i];
}
public void sort()
{
int n = arr.length;
// Build heap (rearrange array)
int startIndex = (n / 2) - 1;
// fill statements
// One by one extract an element from heap
for (int i=n-1; i>=0; i--)
{
// Move current root to end
//fill
heapify(arr, n, i);
//fill
// call max heapify on the reduced heap
//fill
}
}
// To heapify a subtree rooted with node i which is
// an index in arr[]. n is size of heap
void heapify(int arr[], int n, int i)
{
int largest = i; // Initialize largest as root
int l = 2*i + 1; // left = 2*i + 1
int r = 2*i + 2; // right = 2*i + 2
// If left child is larger than root
if (l < n && arr[l] > arr[largest])
largest = l;
// If right child is larger than largest so far
if (r < n && arr[r] > arr[largest])
largest = r;
// If largest is not root
if (largest != i)
{
int swap = arr[i];
arr[i] = arr[largest];
arr[largest] = swap;
// Recursively heapify the affected sub-tree
heapify(arr, n, largest);
}
}
/* A utility function to save array of size n as a String */
public String toString()
{
String s=new String();
for (int i=0; i<arr.length; i++)
s=s + " " + arr[i];
return s;
}
```
**Explanation:**
This code defines a simple implementation of the Heap Sort algorithm in Java. Here’s a breakdown of the key components:
1. **Constructor `Heapsort`:**
- Initializes a new array `arr` from the input array.
2. **Method `sort`:**
-
Expert Solution

Step 1
Solution:
Given,
- Implementation of Head Sort in java and also implement the -
- heapSort, buildmax heap, and heapify functionality.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
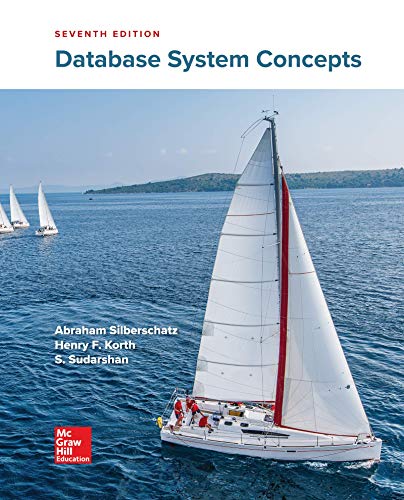
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
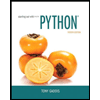
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
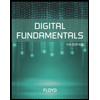
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
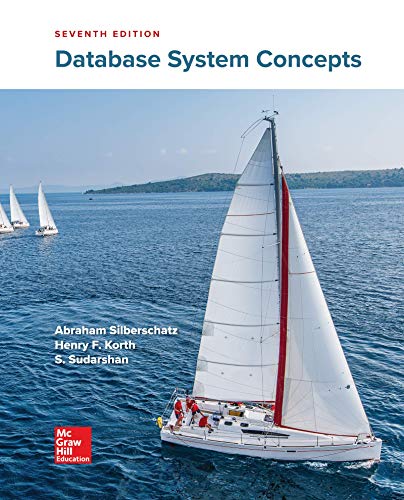
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
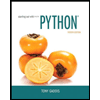
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
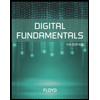
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
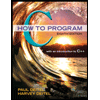
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
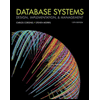
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
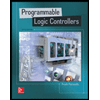
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education