How to show the i) sequence of constructor calls; ii) use of protected access using different packages using the following code. Attaching the picture of the question of the code. //creating class Employee class Employee { //data fields String name; int empID; //parameterized constructor to initialize data fields public Employee(String name, int empID) { this.name = name; this.empID = empID; } //Override public String toString() { return String.format( "Employee: %s (ID: %d)", name, empID); } } import java.util.Scanner; // class HourlyEmployee inherited from Employee class class HourlyEmployee extends Employee { //data fields double hourlyIncome; int totalHours; Scanner scan = new Scanner(System.in); // parameterized constructor to initialize data fields public HourlyEmployee(String name, int empID, double hourlyIncome) { //call base class constructor with arguments super(name, empID); this.hourlyIncome = hourlyIncome; System.out.print("Enter total hours for an Hourly Employee: "); //input number of hours totalHours = scan.nextInt(); } //method to calculate income for totalHours public double calculate_the_hourly_income() { return (totalHours*hourlyIncome); } //Override public String toString() { return String.format("Employee: %s (ID: %d)\nThis month salary = %d * %.2f = %.2f", name, empID, totalHours,hourlyIncome,calculate_the_hourly_income()); } } // class PermanentEmployee inherited from Employee class class PermanentEmployee extends Employee { double hourlyIncome; // parameterized constructor to initialize data fields public PermanentEmployee(String name, int empID, double hourlyIncome) { //call base class constructor with arguments super(name, empID); this.hourlyIncome = hourlyIncome; } //method to calculate income for exact 180 hrs public double calculate_the_income() { return (180*hourlyIncome); } //Override public String toString() { return String.format("Employee: %s (ID: %d)\nThis month salary @%.2f PKR/Hour = %.2f", name, empID,hourlyIncome,calculate_the_income()); } } //test class public class Main { //main method public static void main(String[] args) { //create and initialize HourlyEmployee object HourlyEmployee he = new HourlyEmployee("Ali",786,500); //create and initialize PermanentEmployee object PermanentEmployee pe = new PermanentEmployee("Ali",786,500); System.out.println("-------Hourly Employee--------"); //call toString() method of HourlyEmployee System.out.println(he); System.out.println("-------Permanent Employee---------"); //call toString() method of PermanentEmployee System.out.println(pe); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
How to show the i) sequence of constructor calls; ii) use of protected access using different packages using the following code.
Attaching the picture of the question of the code.
//creating class Employee
class Employee {
//data fields
String name;
int empID;
//parameterized constructor to initialize data fields
public Employee(String name, int empID) {
this.name = name;
this.empID = empID;
}
//Override
public String toString() {
return String.format( "Employee: %s (ID: %d)", name, empID);
}
}
import java.util.Scanner;
// class HourlyEmployee inherited from Employee class
class HourlyEmployee extends Employee {
//data fields
double hourlyIncome;
int totalHours;
Scanner scan = new Scanner(System.in);
// parameterized constructor to initialize data fields
public HourlyEmployee(String name, int empID, double hourlyIncome) {
//call base class constructor with arguments
super(name, empID);
this.hourlyIncome = hourlyIncome;
System.out.print("Enter total hours for an Hourly Employee: ");
//input number of hours
totalHours = scan.nextInt();
}
//method to calculate income for totalHours
public double calculate_the_hourly_income() {
return (totalHours*hourlyIncome);
}
//Override
public String toString() {
return String.format("Employee: %s (ID: %d)\nThis month salary = %d * %.2f = %.2f", name, empID, totalHours,hourlyIncome,calculate_the_hourly_income());
}
}
// class PermanentEmployee inherited from Employee class
class PermanentEmployee extends Employee {
double hourlyIncome;
// parameterized constructor to initialize data fields
public PermanentEmployee(String name, int empID, double hourlyIncome) {
//call base class constructor with arguments
super(name, empID);
this.hourlyIncome = hourlyIncome;
}
//method to calculate income for exact 180 hrs
public double calculate_the_income() {
return (180*hourlyIncome);
}
//Override
public String toString() {
return String.format("Employee: %s (ID: %d)\nThis month salary @%.2f PKR/Hour = %.2f", name, empID,hourlyIncome,calculate_the_income());
}
}
//test class
public class Main
{
//main method
public static void main(String[] args) {
//create and initialize HourlyEmployee object
HourlyEmployee he = new HourlyEmployee("Ali",786,500);
//create and initialize PermanentEmployee object
PermanentEmployee pe = new PermanentEmployee("Ali",786,500);
System.out.println("-------Hourly Employee--------");
//call toString() method of HourlyEmployee
System.out.println(he);
System.out.println("-------Permanent Employee---------");
//call toString() method of PermanentEmployee
System.out.println(pe);
}
}


Step by step
Solved in 2 steps

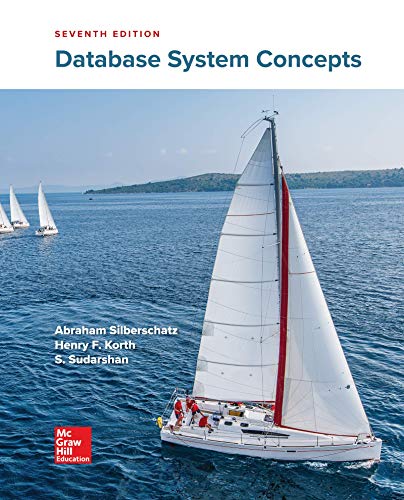
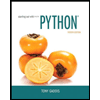
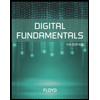
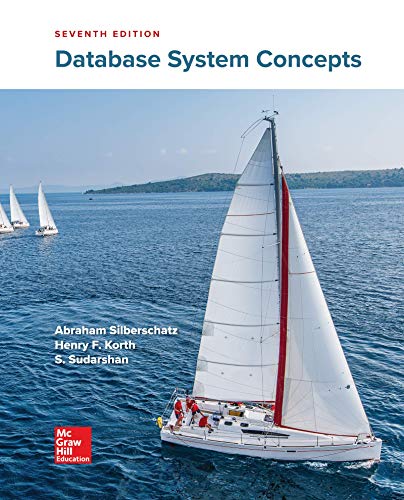
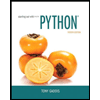
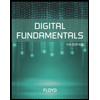
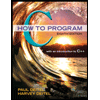
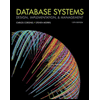
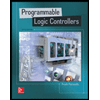