how to write a main function that combine all of these different functions so after switchButton is pressed toggled led run and after that RGBled will run and after pressing start_button the printWaterLevel and readWaterTemperature will run and lastly without pressing anything run_washing_machine_cycle and check_force_sensor will run and after pressing switchButton again everything turn off, the code does work and this pins are identified but not in the required sequence // Function to toggle the LEDs void toggleLEDs() { staticbool ledsOn =false; // Initialize LED state to off ledsOn = !ledsOn; // Toggle LED state if (ledsOn) { // Turn on all LEDs ledRed = 1; ledGreen1 = 1; ledGreen2 = 1; ledGreen3 = 1; } else { // Turn off all LEDs ledRed = 0; ledGreen1 = 0; ledGreen2 = 0; ledGreen3 = 0; LedRed.write(0); // Turn off RGB LED LedGreen.write(0); LedBlue.write(0); } if (ledsOn && switchCount ==2) { // If LEDs are turned on and switch has been pressed twice ledRed = 0; // Turn off red LED } } void RGBled() { // Read potentiometer value and map it to the range [0,1] float value =pot.read(); // Map potentiometer value to different ranges for each color LedRed.write(value *0.5f); // Red - maps from [0, 0.5] range LedGreen.write((value -0.5f) *2.0f); // Green - maps from [0.5, 1] range LedBlue.write(1.0f- value); // Blue - maps from [1, 0] range } void run_washing_machine_cycle() { bool wash_done =false; bool rinse_done =false; bool dry_done =false; bool cycle_complete =false; while (!cycle_complete) { // Wash cycle ledGreen1 = 1; display = 0b00010000; // Display "wash" on 7 segment display printf("Wash cycle started.\n"); int cycle_time_left =10; while (cycle_time_left >0) { display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10); ThisThread::sleep_for(WAIT_TIME_MS); cycle_time_left--; } cycle_time_left = 5; wash_done = true; ledGreen1 = 0; // Rinse cycle ledGreen2 = 1; display = 0b00100000; // Display "rinse" on 7 segment display printf("Rinse cycle started.\n"); while (cycle_time_left >0) { display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10); ThisThread::sleep_for(WAIT_TIME_MS); cycle_time_left--; } cycle_time_left = 5; rinse_done = true; ledGreen2 = 0; // Dry cycle ledGreen3 = 1; display = 0b01000000; // Display "dry" on 7 segment display printf("Dry cycle started.\n"); while (cycle_time_left >0) { display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10); ThisThread::sleep_for(WAIT_TIME_MS); cycle_time_left--; } cycle_time_left = 0; dry_done = true; ledGreen3 = 0; // Cycle complete cycle_complete = true; ledGreen1 = 0; ledGreen2 = 0; ledGreen2 = 0; ledGreen3 = 0; ledRed = 1; play_buzzer(); } } void printWaterLevel() { // Read water level sensor value and print it to the serial monitor int waterLevel =analog_value.read_u16(); if (waterLevel <=0) { printf("%d | Empty\n", waterLevel); } else if (waterLevel <= 150) { printf("%d | Water level is low\n", waterLevel); } else if (waterLevel <= 400) { printf("%d | Water level is medium\n", waterLevel); } else { printf("%d | Water level is high\n", waterLevel); } } void readWaterTemperature() { // Read the analog input for water temperature sensor and convert it to a temperature value float temp =water_temp_sensor.read() *100; if(temp >30.0) { ledRed = 1; // Turn on LED1 and turn off all other LEDs ledGreen1 = 0; ledGreen2 = 0; ledGreen3 = 0; } else { ledRed = 0; // Turn off LED1 and turn on all other LEDs ledGreen1 = 1; ledGreen2 = 1; ledGreen3 = 1; } } void check_force_sensor() { // Read the analog input for force sensor and convert it to a force value float force =force_sensor.read() *100; if(force >80.0) { printf("Open door\r\n"); // Send a message over serial to indicate that the door should be opened } }
how to write a main function that combine all of these different functions so after switchButton is pressed toggled led run and after that RGBled will run and after pressing start_button the printWaterLevel and readWaterTemperature will run and lastly without pressing anything run_washing_machine_cycle and check_force_sensor will run and after pressing switchButton again everything turn off, the code does work and this pins are identified but not in the required sequence // Function to toggle the LEDs void toggleLEDs() { staticbool ledsOn =false; // Initialize LED state to off ledsOn = !ledsOn; // Toggle LED state if (ledsOn) { // Turn on all LEDs ledRed = 1; ledGreen1 = 1; ledGreen2 = 1; ledGreen3 = 1; } else { // Turn off all LEDs ledRed = 0; ledGreen1 = 0; ledGreen2 = 0; ledGreen3 = 0; LedRed.write(0); // Turn off RGB LED LedGreen.write(0); LedBlue.write(0); } if (ledsOn && switchCount ==2) { // If LEDs are turned on and switch has been pressed twice ledRed = 0; // Turn off red LED } } void RGBled() { // Read potentiometer value and map it to the range [0,1] float value =pot.read(); // Map potentiometer value to different ranges for each color LedRed.write(value *0.5f); // Red - maps from [0, 0.5] range LedGreen.write((value -0.5f) *2.0f); // Green - maps from [0.5, 1] range LedBlue.write(1.0f- value); // Blue - maps from [1, 0] range } void run_washing_machine_cycle() { bool wash_done =false; bool rinse_done =false; bool dry_done =false; bool cycle_complete =false; while (!cycle_complete) { // Wash cycle ledGreen1 = 1; display = 0b00010000; // Display "wash" on 7 segment display printf("Wash cycle started.\n"); int cycle_time_left =10; while (cycle_time_left >0) { display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10); ThisThread::sleep_for(WAIT_TIME_MS); cycle_time_left--; } cycle_time_left = 5; wash_done = true; ledGreen1 = 0; // Rinse cycle ledGreen2 = 1; display = 0b00100000; // Display "rinse" on 7 segment display printf("Rinse cycle started.\n"); while (cycle_time_left >0) { display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10); ThisThread::sleep_for(WAIT_TIME_MS); cycle_time_left--; } cycle_time_left = 5; rinse_done = true; ledGreen2 = 0; // Dry cycle ledGreen3 = 1; display = 0b01000000; // Display "dry" on 7 segment display printf("Dry cycle started.\n"); while (cycle_time_left >0) { display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10); ThisThread::sleep_for(WAIT_TIME_MS); cycle_time_left--; } cycle_time_left = 0; dry_done = true; ledGreen3 = 0; // Cycle complete cycle_complete = true; ledGreen1 = 0; ledGreen2 = 0; ledGreen2 = 0; ledGreen3 = 0; ledRed = 1; play_buzzer(); } } void printWaterLevel() { // Read water level sensor value and print it to the serial monitor int waterLevel =analog_value.read_u16(); if (waterLevel <=0) { printf("%d | Empty\n", waterLevel); } else if (waterLevel <= 150) { printf("%d | Water level is low\n", waterLevel); } else if (waterLevel <= 400) { printf("%d | Water level is medium\n", waterLevel); } else { printf("%d | Water level is high\n", waterLevel); } } void readWaterTemperature() { // Read the analog input for water temperature sensor and convert it to a temperature value float temp =water_temp_sensor.read() *100; if(temp >30.0) { ledRed = 1; // Turn on LED1 and turn off all other LEDs ledGreen1 = 0; ledGreen2 = 0; ledGreen3 = 0; } else { ledRed = 0; // Turn off LED1 and turn on all other LEDs ledGreen1 = 1; ledGreen2 = 1; ledGreen3 = 1; } } void check_force_sensor() { // Read the analog input for force sensor and convert it to a force value float force =force_sensor.read() *100; if(force >80.0) { printf("Open door\r\n"); // Send a message over serial to indicate that the door should be opened } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
how to write a main function that combine all of these different functions so after switchButton is pressed toggled led run and after that RGBled will run and after pressing start_button the printWaterLevel and readWaterTemperature will run and lastly without pressing anything run_washing_machine_cycle and check_force_sensor will run and after pressing switchButton again everything turn off, the code does work and this pins are identified but not in the required sequence
// Function to toggle the LEDs
void toggleLEDs() {
staticbool ledsOn =false; // Initialize LED state to off
ledsOn = !ledsOn; // Toggle LED state
if (ledsOn) { // Turn on all LEDs
ledRed = 1;
ledGreen1 = 1;
ledGreen2 = 1;
ledGreen3 = 1;
} else { // Turn off all LEDs
ledRed = 0;
ledGreen1 = 0;
ledGreen2 = 0;
ledGreen3 = 0;
LedRed.write(0); // Turn off RGB LED
LedGreen.write(0);
LedBlue.write(0);
}
if (ledsOn && switchCount ==2) { // If LEDs are turned on and switch has been pressed twice
ledRed = 0; // Turn off red LED
}
}
void RGBled() {
// Read potentiometer value and map it to the range [0,1]
float value =pot.read();
// Map potentiometer value to different ranges for each color
LedRed.write(value *0.5f); // Red - maps from [0, 0.5] range
LedGreen.write((value -0.5f) *2.0f); // Green - maps from [0.5, 1] range
LedBlue.write(1.0f- value); // Blue - maps from [1, 0] range
}
void run_washing_machine_cycle() {
bool wash_done =false;
bool rinse_done =false;
bool dry_done =false;
bool cycle_complete =false;
while (!cycle_complete) {
// Wash cycle
ledGreen1 = 1;
display = 0b00010000; // Display "wash" on 7 segment display
printf("Wash cycle started.\n");
int cycle_time_left =10;
while (cycle_time_left >0) {
display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10);
ThisThread::sleep_for(WAIT_TIME_MS);
cycle_time_left--;
}
cycle_time_left = 5;
wash_done = true;
ledGreen1 = 0;
// Rinse cycle
ledGreen2 = 1;
display = 0b00100000; // Display "rinse" on 7 segment display
printf("Rinse cycle started.\n");
while (cycle_time_left >0) {
display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10);
ThisThread::sleep_for(WAIT_TIME_MS);
cycle_time_left--;
}
cycle_time_left = 5;
rinse_done = true;
ledGreen2 = 0;
// Dry cycle
ledGreen3 = 1;
display = 0b01000000; // Display "dry" on 7 segment display
printf("Dry cycle started.\n");
while (cycle_time_left >0) {
display = (int_to_display(cycle_time_left / 10) << 8) | int_to_display(cycle_time_left % 10);
ThisThread::sleep_for(WAIT_TIME_MS);
cycle_time_left--;
}
cycle_time_left = 0;
dry_done = true;
ledGreen3 = 0;
// Cycle complete
cycle_complete = true;
ledGreen1 = 0;
ledGreen2 = 0;
ledGreen2 = 0;
ledGreen3 = 0;
ledRed = 1;
play_buzzer();
}
}
void printWaterLevel() {
// Read water level sensor value and print it to the serial monitor
int waterLevel =analog_value.read_u16();
if (waterLevel <=0) {
printf("%d | Empty\n", waterLevel);
} else if (waterLevel <= 150) {
printf("%d | Water level is low\n", waterLevel);
} else if (waterLevel <= 400) {
printf("%d | Water level is medium\n", waterLevel);
} else {
printf("%d | Water level is high\n", waterLevel);
}
}
void readWaterTemperature() {
// Read the analog input for water temperature sensor and convert it to a temperature value
float temp =water_temp_sensor.read() *100;
if(temp >30.0) {
ledRed = 1; // Turn on LED1 and turn off all other LEDs
ledGreen1 = 0;
ledGreen2 = 0;
ledGreen3 = 0;
} else {
ledRed = 0; // Turn off LED1 and turn on all other LEDs
ledGreen1 = 1;
ledGreen2 = 1;
ledGreen3 = 1;
}
}
void check_force_sensor() {
// Read the analog input for force sensor and convert it to a force value
float force =force_sensor.read() *100;
if(force >80.0) {
printf("Open door\r\n"); // Send a message over serial to indicate that the door should be opened
}
}
int main() {
printf("System started\n");
while (1) {
// Check if switch is pressed
if (switchButton ==0) {
switchCount++; // Increment switch count
toggleLEDs(); // Toggle the LEDs
RGBled();
while (switchButton ==0); // Wait until switch is released
}
}
RGBled();
readWaterTemperature();
printWaterLevel();
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
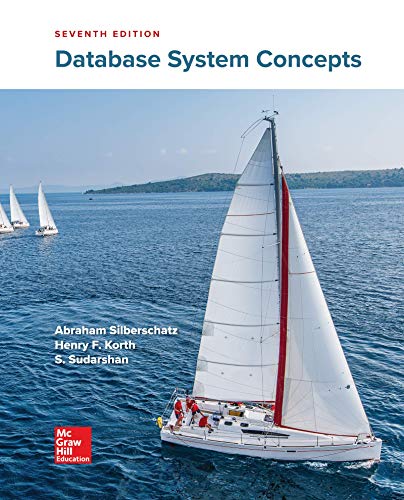
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
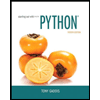
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
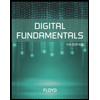
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
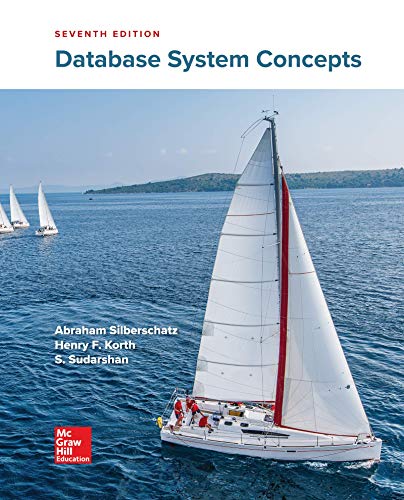
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
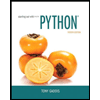
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
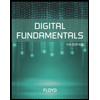
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
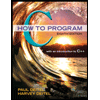
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
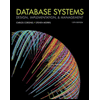
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
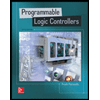
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education