How to display the python code in tabular format. import csv def main(): n = int(input("Enter the number of students: ")) rows = [["First Name", "Last Name", "Ex_am 1 Grade", "Ex_am 2 Grade", "Ex_am 3 Grade", "Average"]] for i in range(n): first = input("\nEnter First Name of Student "+str(i+1)+": ") last = input("Enter Last Name of Student "+str(i+1)+": ") ex1 = int(input("Enter Ex_am 1 Grade of Student "+str(i+1)+": ")) ex2 = int(input("Enter Ex_am 2 Grade of Student "+str(i+1)+": ")) ex3 = int(input("Enter Ex_am 3 Grade of Student "+str(i+1)+": ")) avg = (ex1+ex2+ex3)/3.0 rows.append([first,last,ex1,ex2,ex3,avg]) with open("grades.csv",'w',newline = '') as _file: file = csv.writer(_file) for i in rows: file.writerow(i) print("\nWriting Done.\n") with open("grades.csv",'r') as _file: file = csv.reader(_file) header = next(file) for i in file: i[2] = int(i[2]) i[3] = int(i[3]) i[4] = int(i[4]) i[5] = float(i[5]) print(i) main()
How to display the python code in tabular format.
import csv
def main():
n = int(input("Enter the number of students: "))
rows = [["First Name", "Last Name", "Ex_am 1 Grade", "Ex_am 2 Grade", "Ex_am 3 Grade", "Average"]]
for i in range(n):
first = input("\nEnter First Name of Student "+str(i+1)+": ")
last = input("Enter Last Name of Student "+str(i+1)+": ")
ex1 = int(input("Enter Ex_am 1 Grade of Student "+str(i+1)+": "))
ex2 = int(input("Enter Ex_am 2 Grade of Student "+str(i+1)+": "))
ex3 = int(input("Enter Ex_am 3 Grade of Student "+str(i+1)+": "))
avg = (ex1+ex2+ex3)/3.0
rows.append([first,last,ex1,ex2,ex3,avg])
with open("grades.csv",'w',newline = '') as _file:
file = csv.writer(_file)
for i in rows:
file.writerow(i)
print("\nWriting Done.\n")
with open("grades.csv",'r') as _file:
file = csv.reader(_file)
header = next(file)
for i in file:
i[2] = int(i[2])
i[3] = int(i[3])
i[4] = int(i[4])
i[5] = float(i[5])
print(i)
main()

To print the information in tabular format.
Step by step
Solved in 3 steps with 1 images

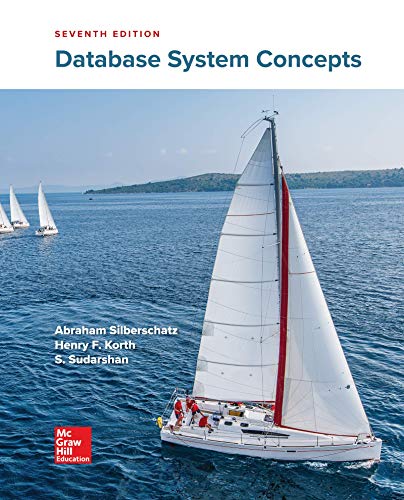
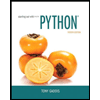
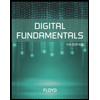
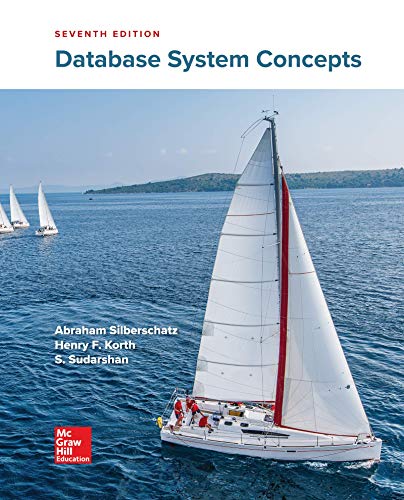
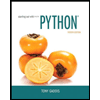
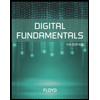
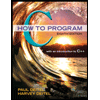
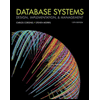
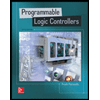