How to answer the following questions? Here is a, possibly flawed, approach for using a traversal to print the contents of the linked list of strings accessed through letters. Indicate the description that best matches the code. LLNode currNode = letters; while (currNode != null) { System.out.println(currNode.getInfo()); if (currNode.getLink() != null) currNode = currNode.getLink(); else currNode = null; } A. very good B. correct but inefficient C. correct but too complex D. will bomb if list is empty E. will bomb if list is not empty What is the result of evaluating the following postfix expression? 6 4 + * 3 10 + A. 0 B. 3 C. too many operands D. too few operands stack implementations throw an exception if a pop is attempted on an empty stack. A valid alternate approach to handling this situation is to: A. ignore it B. state as a precondition that pop will not be attempted on an empty stack. C. have the pop operation return a boolean indicating success. D state as a precondition that pop will not be attempted on an empty stack, and have the pop operation return a boolean indicating success. E. state as a precondition that pop will not be attempted on an empty stack, or have the pop operation return a boolean indicating success.
How to answer the following questions?
Here is a, possibly flawed, approach for using a traversal to print the contents of the linked list of strings accessed through letters. Indicate the description that best matches the code.
LLNode<String> currNode = letters;
while (currNode != null) {
System.out.println(currNode.getInfo());
if (currNode.getLink() != null)
currNode = currNode.getLink();
else
currNode = null;
}
What is the result of evaluating the following postfix expression?
6 4 + * 3 10 +
A. 0 B. 3 C. too many operands D. too few operands
stack implementations throw an exception if a pop is attempted on an empty stack. A valid alternate approach to handling this situation is to:
which of these statements is the best representation of the current status of a Stack ADT in the Java Standard Library?
A. There is no support for stacks in the library. B. The library provides a Stack class.
C. The library does not provide a Stack class, but does provide other classes that can be used for the same functionality.


Trending now
This is a popular solution!
Step by step
Solved in 4 steps

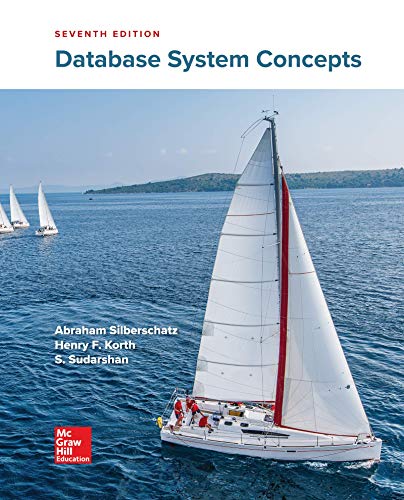
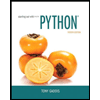
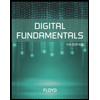
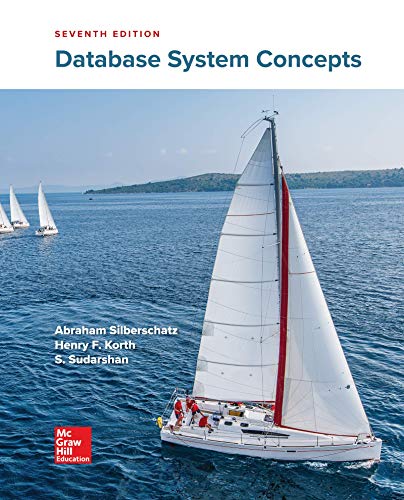
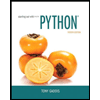
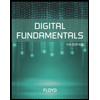
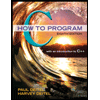
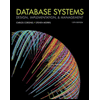
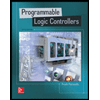