Write a counting program in MIPS assembly. The program should print the first sixteen powers of 2 beginning with 2^0 with a space between each value. The output of the program should be exactly as follows: 1 2 4 8 16 32 64 128 256 512 1024 2048 4096 8192 16384 32768 Additional requirements for this problem: You must use a logical shift operation in your implementation. You must use a loop (i.e., a conditional branch that causes program instructions to repeat).
Write a counting program in MIPS assembly. The program should print the first sixteen powers of 2 beginning with 2^0 with a space between each value. The output of the program should be exactly as follows:
1 2 4 8 16 32 64 128 256 512 1024 2048 4096 8192 16384 32768
Additional requirements for this problem: You must use a logical shift operation in your implementation. You must use a loop (i.e., a conditional branch that causes program instructions to repeat).

li $t0, 16
li $t1, 0
li $t3, 1
loop:
beq $t1, $t0, end
li $v0, 1
move $a0, $t3
syscall
sll $t3, $t3, 1
addi $t1, $t1, 1
j loop
end:
li $v0, 10
syscall
jr $ra
Step by step
Solved in 2 steps

How to add space in between each power of 2 printed by this mips assembly
The output currently is:
124816326412825651210242048409681921638432768
When it needs to be:
1 2 4 8 16 32 64 128 256 512 1024 2048 4096 8192 16384 32768
-------
main:
li $t0, 16
li $t1, 0
li $t3, 1
loop:
beq $t1, $t0, end
li $v0, 1
move $a0, $t3
syscall
sll $t3, $t3, 1
addi $t1, $t1, 1
j loop
end:
li $v0, 10
syscall
jr $ra
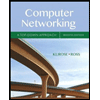
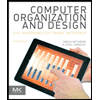
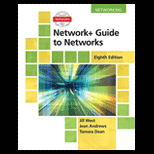
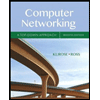
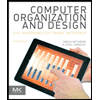
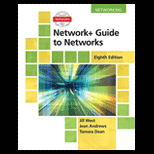
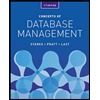
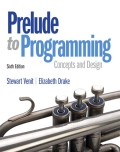
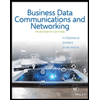