/* how many time are the if conditions executed? */ public static void fw (int[] [] G) { int n G.length; // G is a square array for(int i=0; i G[j] [i] + G[i] [k]) { G[j] [k] = G[j] [i] + G[i] [k]; } /* how would you describe the runtime of the above code? */
/* how many time are the if conditions executed? */ public static void fw (int[] [] G) { int n G.length; // G is a square array for(int i=0; i G[j] [i] + G[i] [k]) { G[j] [k] = G[j] [i] + G[i] [k]; } /* how would you describe the runtime of the above code? */
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help finding out the run time of the following
![```java
/* how many time are the if conditions executed? */
public static void fw(int[][] G) {
int n = G.length; // G is a square array
for (int i=0; i<G.length; i++ ) {
for (int j=0; j<n; j++ ) {
if( i==j ) {
continue;
}
else {
G[i][j] = (int) (Math.random()*n);
}
}
}
for (int i=0; i<n; i++ ) {
for (int j=0; j<n; j++ ) {
for (int k=0; k<n; k++ ) {
if( G[j][k] > G[j][i] + G[i][k] ) {
G[j][k] = G[j][i] + G[i][k];
}
}
}
}
}
/* how would you describe the runtime of the above code? */
```
### Explanation:
This code defines a method `fw` that operates on a square 2D integer array `G`.
1. **First Nested Loops:**
- The first set of nested loops iterates through all elements of the array `G` except for diagonal elements (where `i == j`).
- If `i != j`, it assigns a random integer to `G[i][j]`.
2. **Second Nested Loops:**
- The second set of nested loops performs an operation similar to the Floyd-Warshall algorithm for finding shortest paths between all pairs of nodes in a weighted graph.
- It checks if the direct path from `j` to `k` is more than the path going through `i` (`G[j][i] + G[i][k]`). If so, it updates `G[j][k]` to be the smaller path sum.
### Runtime:
The runtime complexity for this code is O(n³), where `n` is the length of one side of the square array `G`. This is because of the three nested loops iterating over `n` elements.
The questions raised are:
- How many times are the if conditions executed?
- How would you describe the runtime of the provided code?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84b9759d-3bd7-481b-9da5-01ee09be0953%2F6081e4c4-b130-4ad8-809f-515305ae40ff%2Fihbkqpx_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```java
/* how many time are the if conditions executed? */
public static void fw(int[][] G) {
int n = G.length; // G is a square array
for (int i=0; i<G.length; i++ ) {
for (int j=0; j<n; j++ ) {
if( i==j ) {
continue;
}
else {
G[i][j] = (int) (Math.random()*n);
}
}
}
for (int i=0; i<n; i++ ) {
for (int j=0; j<n; j++ ) {
for (int k=0; k<n; k++ ) {
if( G[j][k] > G[j][i] + G[i][k] ) {
G[j][k] = G[j][i] + G[i][k];
}
}
}
}
}
/* how would you describe the runtime of the above code? */
```
### Explanation:
This code defines a method `fw` that operates on a square 2D integer array `G`.
1. **First Nested Loops:**
- The first set of nested loops iterates through all elements of the array `G` except for diagonal elements (where `i == j`).
- If `i != j`, it assigns a random integer to `G[i][j]`.
2. **Second Nested Loops:**
- The second set of nested loops performs an operation similar to the Floyd-Warshall algorithm for finding shortest paths between all pairs of nodes in a weighted graph.
- It checks if the direct path from `j` to `k` is more than the path going through `i` (`G[j][i] + G[i][k]`). If so, it updates `G[j][k]` to be the smaller path sum.
### Runtime:
The runtime complexity for this code is O(n³), where `n` is the length of one side of the square array `G`. This is because of the three nested loops iterating over `n` elements.
The questions raised are:
- How many times are the if conditions executed?
- How would you describe the runtime of the provided code?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
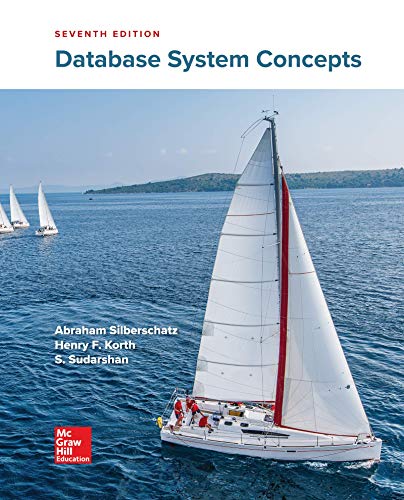
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
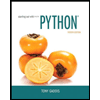
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
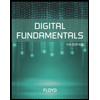
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
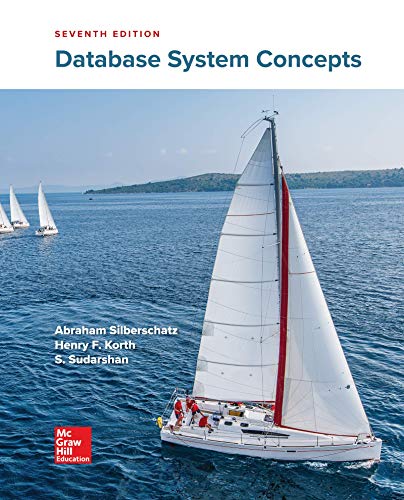
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
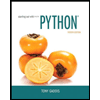
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
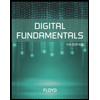
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
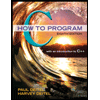
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
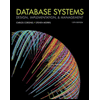
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
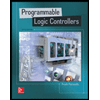
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education