How do I create a class Battle, with the following instance attributes: iron_chef: a Chef object challenger: a Chef object secret_ingredient: a string (the ingredient to be used by the chefs in this battle) dishes: a list of Dish objects outcome: an empty string The Battle class should also have the following instance method: conclude: Make the critics rate the dishes in the battle, and then set the outcome attribute to either 'iron_chef', 'challenger', or 'tie' based on the results. (Whichever chef gets a higher total rating across all dishes is declared the winner.) BELOW IS THE CODE I HAVE -------------------------------------- class Battle: def __init__(self, iron_chef, challenger, secret_ingredient, dishes, outcome=''): '''(Chef, Chef, string, list, string) -> Battle''' self.iron_chef = iron_chef self.challenger = challenger self.secret_ingredient = secret_ingredient self.dishes = dishes self.outcome = outcome def __str__(self): s = "Iron Chef: \t\t" + self.iron_chef.name s += "\nChallenger: \t\t" + self.challenger.name s += "\nSecret ingredient: \t" + self.secret_ingredient dishnames = '' for d in self.dishes: dishnames += str(d.name) + ", " s += "\nDishes: \t\t" + str(dishnames[:-2]) # Probably a bad way but it works return s
How do I create a class Battle, with the following instance attributes:
iron_chef: a Chef object
challenger: a Chef object
secret_ingredient: a string (the ingredient to be used by the chefs in this battle)
dishes: a list of Dish objects
outcome: an empty string
The Battle class should also have the following instance method:
conclude: Make the critics rate the dishes in the battle, and then set the outcome attribute to either 'iron_chef', 'challenger', or 'tie' based on the results. (Whichever chef gets a higher total rating across all dishes is declared the winner.)
BELOW IS THE CODE I HAVE
--------------------------------------
class Battle:
def __init__(self, iron_chef, challenger, secret_ingredient, dishes, outcome=''):
'''(Chef, Chef, string, list, string) -> Battle'''
self.iron_chef = iron_chef
self.challenger = challenger
self.secret_ingredient = secret_ingredient
self.dishes = dishes
self.outcome = outcome
def __str__(self):
s = "Iron Chef: \t\t" + self.iron_chef.name
s += "\nChallenger: \t\t" + self.challenger.name
s += "\nSecret ingredient: \t" + self.secret_ingredient
dishnames = ''
for d in self.dishes:
dishnames += str(d.name) + ", "
s += "\nDishes: \t\t" + str(dishnames[:-2]) # Probably a bad way but it works
return s

Step by step
Solved in 3 steps with 1 images

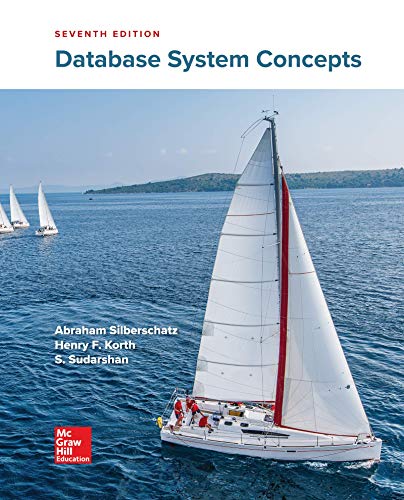
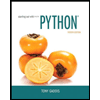
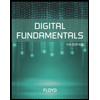
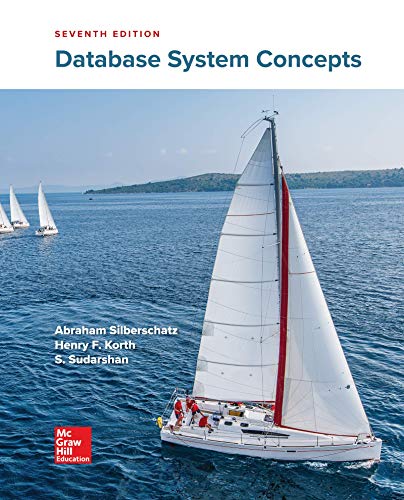
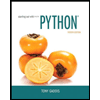
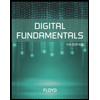
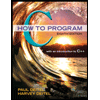
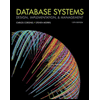
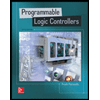