how can i fix my code to get the expected output ? my code : main.py: import csv from ItemToPurchase import ItemToPurchase, Item def main(): items = {} with open('available_items.csv', newline='') as csvfile: reader = csv.DictReader(csvfile) for row in reader: item_id = row['item_id'] description = row['description'] price = float(row['price']) items[item_id] = Item(description, price) cart = [] while True: print('Enter the id of an item you wish to purchase:') item_id = input().lower() if item_id in items: item = items[item_id] print('Enter the quantity of {} to purchase:'.format(item.d
how can i fix my code to get the expected output ?
my code :
main.py:
import csv
from ItemToPurchase import ItemToPurchase, Item
def main():
items = {}
with open('available_items.csv', newline='') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
item_id = row['item_id']
description = row['description']
price = float(row['price'])
items[item_id] = Item(description, price)
cart = []
while True:
print('Enter the id of an item you wish to purchase:')
item_id = input().lower()
if item_id in items:
item = items[item_id]
print('Enter the quantity of {} to purchase:'.format(item.description))
quantity = int(input())
if quantity > 0:
i = ItemToPurchase(item, quantity)
cart.append(i)
i.print()
else:
print('Invalid quantity')
elif item_id == 'q':
break
else:
print('Invalid item id')
total = 0
for item in cart:
item.print()
total += item.cost()
print('\n Total $ {:>6.2f}'.format(total))
if __name__ == '__main__':
main()
ItemToPurchase.py:
from collections import namedtuple
Item = namedtuple('Item', ('description', 'price',))
class ItemToPurchase:
def __init__(self, item:Item, quantity:int):
self.item = item
self.quantity = quantity
def cost(self):
return self.quantity * self.item.price
def print(self):
desc = self.item.description
price = self.item.price
qty = self.quantity
total = self.cost()
print(' {} {:<25} @ $ {:0.2f} ea. = ${:>6.2f}'.format(qty, desc, price, total))


Step by step
Solved in 2 steps

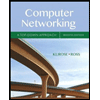
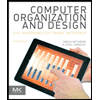
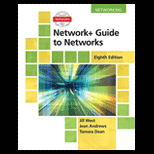
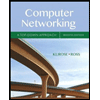
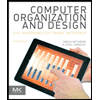
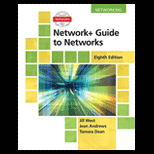
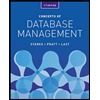
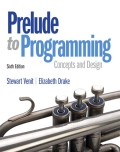
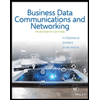