Hi, I need some help with the "Your solution should allow the user to exit the program as well as add one hour, minute, or second to both clocks from a user menu" requirement of my C++ program. So far, my code will print the local time in a 12hr and 24hr format, I just need some help with implimenting the user input to add an hour, minute, or second. Thanks Below is my current code, 3 C++ files one of which is a header file: ClockTime.cpp #include "ClockTime.h" //default Constructor ClockTime::ClockTime() { time_t now = time(0); //gets current Time tm *ltm = localtime(&now); //converts current Time to struct tm type this->hour = ltm->tm_hour; this->minute = ltm->tm_min; this->second = ltm->tm_sec; } // parameter constructor ClockTime::ClockTime(int hour, int min, int sec) { this->hour = hour; this->minute = min; this->second = sec; } void ClockTime::addHour(int hour = 1) { this->hour = (this->hour + hour) % 24; } void ClockTime::addMinute(int min = 1) { if (min == 59){ this->addHour(); } this->minute = (this->minute + min) % 60; } void ClockTime::addSecond(int sec = 1) { if (this->second == 59){ this->addMinute(); } this->second = (this->second + sec) % 60; } // function return AM/PM respect to hour of time string ClockTime::getAM_PM() { return this->hour >= 12 ? "PM" : "AM"; } // function returns integer used in 12 hour format int ClockTime::gethour_12() { return (this->hour == 0 || this->hour == 12) ? 12 : this->hour % 12; } // function displays 12 hour formatClock void ClockTime::display_12_HourTime() { cout.fill('0'); cout << setw(2) << this->gethour_12()<< ":" << setw(2) << this->minute << ":" << setw(2) << this->second << " " << this->getAM_PM(); } // function displays 24 hour formatClock void ClockTime::display_24_HourTime() { cout.fill('0'); cout << setw(2) << this->hour << ":" << setw(2) << this->minute << ":" << setw(2) << this->second; } // Function Displays Both Clocks void ClockTime::displayClock() { cout << "**************************" << " " << "**************************\n"; cout << "* 12-Hour Clock *" << " " << "* 24-Hour Clock *\n"; cout << "* "; this->display_12_HourTime(); cout << " * * "; this->display_24_HourTime(); cout << " *\n"; cout << "**************************" << " " << "**************************\n"; } _____________________________________________________________ mainDrive.cpp #include"ClockTime.h" int main() { ClockTime t; t.displayClock(); return 0; } _____________________________________________________________ ClockTime.h #pragma once #ifndef CLOCK_TIME_H #define CLOCK_TIME_H #define _CRT_SECURE_NO_WARNINGS #include #include #include #include using namespace std; class ClockTime { private: int hour; int minute; int second; void display_12_HourTime(); void display_24_HourTime(); public: ClockTime(); ClockTime(int , int, int ); void addSecond(int ); void addMinute(int ); void addHour(int ); string getAM_PM(); int gethour_12(); void displayClock(); }; #endif
Hi, I need some help with the "Your solution should allow the user to exit the program as well as add one hour, minute, or second to both clocks from a user menu" requirement of my C++ program.
So far, my code will print the local time in a 12hr and 24hr format, I just need some help with implimenting the user input to add an hour, minute, or second. Thanks
Below is my current code, 3 C++ files one of which is a header file:
ClockTime.cpp
#include "ClockTime.h"
//default Constructor
ClockTime::ClockTime()
{
time_t now = time(0); //gets current Time
tm *ltm = localtime(&now); //converts current Time to struct tm type
this->hour = ltm->tm_hour;
this->minute = ltm->tm_min;
this->second = ltm->tm_sec;
}
// parameter constructor
ClockTime::ClockTime(int hour, int min, int sec)
{
this->hour = hour;
this->minute = min;
this->second = sec;
}
void ClockTime::addHour(int hour = 1)
{
this->hour = (this->hour + hour) % 24;
}
void ClockTime::addMinute(int min = 1)
{
if (min == 59){
this->addHour();
}
this->minute = (this->minute + min) % 60;
}
void ClockTime::addSecond(int sec = 1)
{
if (this->second == 59){
this->addMinute();
}
this->second = (this->second + sec) % 60;
}
// function return AM/PM respect to hour of time
string ClockTime::getAM_PM()
{
return this->hour >= 12 ? "PM" : "AM";
}
// function returns integer used in 12 hour format
int ClockTime::gethour_12()
{
return (this->hour == 0 || this->hour == 12) ? 12 : this->hour % 12;
}
// function displays 12 hour formatClock
void ClockTime::display_12_HourTime()
{
cout.fill('0');
cout << setw(2) << this->gethour_12()<< ":" << setw(2) << this->minute << ":" << setw(2) << this->second
<< " " << this->getAM_PM();
}
// function displays 24 hour formatClock
void ClockTime::display_24_HourTime()
{
cout.fill('0');
cout << setw(2) << this->hour << ":" << setw(2) << this->minute << ":" << setw(2) << this->second;
}
// Function Displays Both Clocks
void ClockTime::displayClock()
{
cout << "**************************" << " " << "**************************\n";
cout << "* 12-Hour Clock *" << " " << "* 24-Hour Clock *\n";
cout << "* "; this->display_12_HourTime();
cout << " * * ";
this->display_24_HourTime(); cout << " *\n";
cout << "**************************" << " " << "**************************\n";
}
_____________________________________________________________
mainDrive.cpp
#include"ClockTime.h"
int main()
{
ClockTime t;
t.displayClock();
return 0;
}
_____________________________________________________________
ClockTime.h
#pragma once
#ifndef CLOCK_TIME_H
#define CLOCK_TIME_H
#define _CRT_SECURE_NO_WARNINGS
#include<string>
#include<iostream>
#include <ctime>
#include <iomanip>
using namespace std;
class ClockTime
{
private:
int hour;
int minute;
int second;
void display_12_HourTime();
void display_24_HourTime();
public:
ClockTime();
ClockTime(int , int, int );
void addSecond(int );
void addMinute(int );
void addHour(int );
string getAM_PM();
int gethour_12();
void displayClock();
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

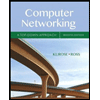
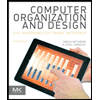
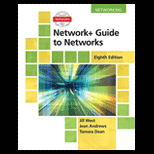
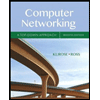
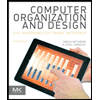
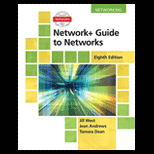
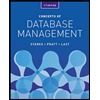
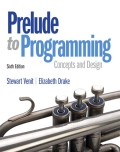
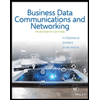