Hi, I need help finishing this code in Java. Instructions: Write a program that reads a sequence of up to 10 names and grade point averages, terminated by a line that contains simply the text end. Store each student's data in an object of class Student designed to store a first name (String), last name (String), and grade point average (float). Assume that each line of input contains two strings followed by a float value, each separated by a comma character. Print the data in the reverse order in which it was read. Then, print the average GPA for the students. Hints: Use a Scanner to read the lines. Use the String split() method to separate the line into parts. Use DecimalFormat to print the average GPA to 2 decimal points. Example input: Joseph,Smith,4.0 Bill,Williams,3.2 end Example output: 3.2: Williams, Bill 4.0: Smith, Joseph Average GPA: 3.60 *screenshot attached is what i have so far.
Hi, I need help finishing this code in Java. Instructions: Write a program that reads a sequence of up to 10 names and grade point averages, terminated by a line that contains simply the text end. Store each student's data in an object of class Student designed to store a first name (String), last name (String), and grade point average (float). Assume that each line of input contains two strings followed by a float value, each separated by a comma character. Print the data in the reverse order in which it was read. Then, print the average GPA for the students. Hints: Use a Scanner to read the lines. Use the String split() method to separate the line into parts. Use DecimalFormat to print the average GPA to 2 decimal points. Example input: Joseph,Smith,4.0 Bill,Williams,3.2 end Example output: 3.2: Williams, Bill 4.0: Smith, Joseph Average GPA: 3.60 *screenshot attached is what i have so far.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hi, I need help finishing this code in Java.
Instructions:
Write a
- Store each student's data in an object of class Student designed to store a first name (String), last name (String), and grade point average (float).
- Assume that each line of input contains two strings followed by a float value, each separated by a comma character.
- Print the data in the reverse order in which it was read.
- Then, print the average GPA for the students.
Hints:
- Use a Scanner to read the lines.
- Use the String split() method to separate the line into parts.
- Use DecimalFormat to print the average GPA to 2 decimal points.
Example input:
Joseph,Smith,4.0
Bill,Williams,3.2
end
Example output:
3.2: Williams, Bill
4.0: Smith, Joseph
Average GPA: 3.60
*screenshot attached is what i have so far.
![Files
Q Find a file
Main.java
Student.java
⠀
Student.java x +
1 ▼ class Student {
234
2
3
4
5
6 ▼
7
8
9
10
123
11
12 ▼
13
45
14
private String firstName;
private String lastName;
private float gpa;
public Student (String firstName, String lastName, float gpa) {
this.firstName = firstName;
this.lastName = lastName;
this.gpa = gpa;
}
public String getFirstName() {
return firstName;
}
15
16 ▼ public String getLastName() {
17
return lastName;
18
19
20 ▼
21
22
23
24 ▼
25
26
27 }
}
public float getGPA() {
return gpa;
}
public String toString() {
return "first: " + firstName +
}
last: + lastName +
GPA:
14]
+ gpa;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd6ded41a-9f92-4a9d-b437-015644cf6784%2F5c1acda0-f0ef-4e7c-8395-7dd22da94c2f%2Fgsjn7a_processed.png&w=3840&q=75)
Transcribed Image Text:Files
Q Find a file
Main.java
Student.java
⠀
Student.java x +
1 ▼ class Student {
234
2
3
4
5
6 ▼
7
8
9
10
123
11
12 ▼
13
45
14
private String firstName;
private String lastName;
private float gpa;
public Student (String firstName, String lastName, float gpa) {
this.firstName = firstName;
this.lastName = lastName;
this.gpa = gpa;
}
public String getFirstName() {
return firstName;
}
15
16 ▼ public String getLastName() {
17
return lastName;
18
19
20 ▼
21
22
23
24 ▼
25
26
27 }
}
public float getGPA() {
return gpa;
}
public String toString() {
return "first: " + firstName +
}
last: + lastName +
GPA:
14]
+ gpa;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
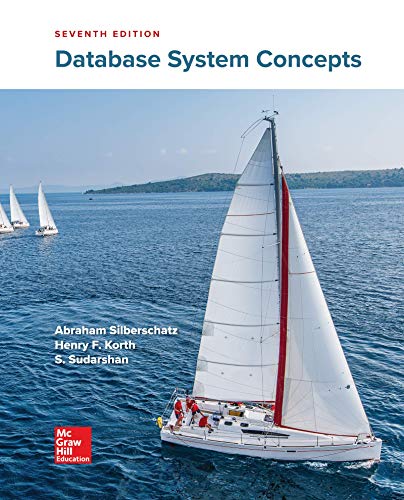
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
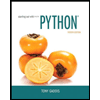
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
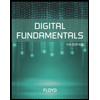
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
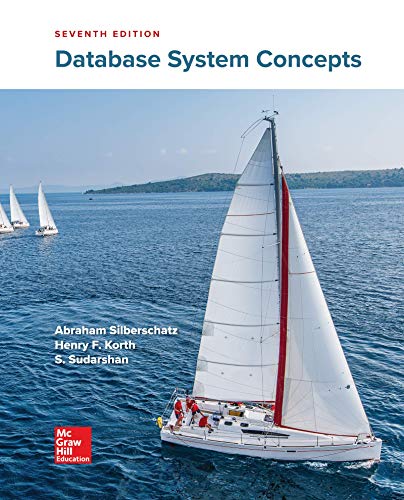
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
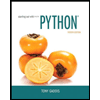
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
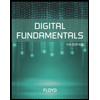
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
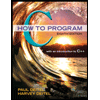
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
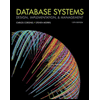
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
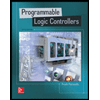
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education