onstructors as NumberGuesser. But the getCurrentGuess method will have different behavior. In the NumberGuesser class getCurrentGuess always returns the midpoint of the range of values that might still be correct. In the RandomNumberGuesser class the getCurrentGuess method should return a random number from within the range of possible values. How to create the RandomNumberGuesser class The RandomNumberGuesser class must be a subclass of NumberGuesser. public class RandomNumberGuesser extends NumberGuesser { // your code here } Feel free to modify your NumberGuesser if that helps. You might want to make your upper and lower bounds protected instead of private. You will definitely need to override the getCurrentGuess method to modify its behavior. You can also override higher() and lower() if that helps. A caveat for the new getCurrentGuess method In the NumberGuesser class getCurrentGuess will always return the same value if it is invoked multiple times between invocations of higher() and lower(). That is because it returns the midpoint of the range. Your new getCurrentGuess method should have that same behavior. It should return a random value in the range. But it should continue to return the same random value until the range is modified by an invocation of higher() and lower(). This can be a little bit tricky. If you have any questions, post in the discussion and we can talk about it. How to put your new RandomNumberGuesser into your guessing game You should be able to use your number guessing game code that i
Java Programming
Write a subclass of your NumberGuesser class named RandomNumberGuesser. It should have the same methods and constructors as NumberGuesser. But the getCurrentGuess method will have different behavior.
In the NumberGuesser class getCurrentGuess always returns the midpoint of the range of values that might still be correct.
In the RandomNumberGuesser class the getCurrentGuess method should return a random number from within the range of possible values.
How to create the RandomNumberGuesser class
The RandomNumberGuesser class must be a subclass of NumberGuesser.
public class RandomNumberGuesser extends NumberGuesser {
// your code here
}
Feel free to modify your NumberGuesser if that helps. You might want to make your upper and lower bounds protected instead of private.
You will definitely need to override the getCurrentGuess method to modify its behavior. You can also override higher() and lower() if that helps.
A caveat for the new getCurrentGuess method
In the NumberGuesser class getCurrentGuess will always return the same value if it is invoked multiple times between invocations of higher() and lower(). That is because it returns the midpoint of the range.
Your new getCurrentGuess method should have that same behavior. It should return a random value in the range. But it should continue to return the same random value until the range is modified by an invocation of higher() and lower().
This can be a little bit tricky. If you have any questions, post in the discussion and we can talk about it.
How to put your new RandomNumberGuesser into your guessing game
You should be able to use your number guessing game code that is in Main.java with only a tiny modification.
Somewhere in your code is the line where you create NumberGuessers. It should look something like this:
NumberGuesser guesser = new NumberGuesser(1, 100);
You can change it by creating a RandomNumberGuesser instead:
NumberGuesser guesser = new RandomNumberGuesser(1, 100);
That should be all that is necessary in Main.java

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Her's my current NumberGuesser
public class NumberGuesser {
private int lowerBound;
private int upperBound;
private int originalLowerBound;
private int originalUpperBound;
public NumberGuesser(int lowerBound, int upperBound){
this.lowerBound = lowerBound;
this.upperBound = upperBound;
this.originalLowerBound = lowerBound;
this.originalUpperBound = upperBound;
}
public void higher() {
lowerBound = getCurrentGuess()+1;
}
public void lower() {
upperBound = getCurrentGuess() -1;
}
public int getCurrentGuess(){
return(lowerBound + upperBound)/2;
}
public void reset() {
lowerBound = originalLowerBound;
upperBound = originalUpperBound;
}
}
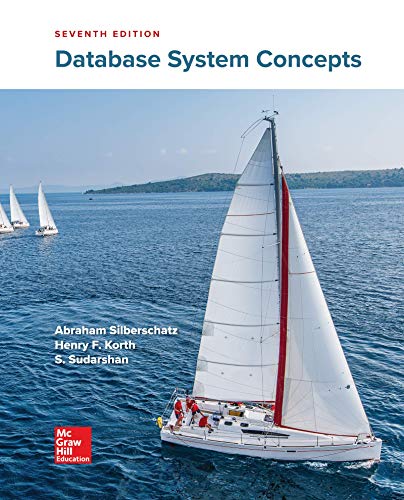
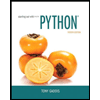
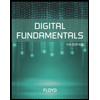
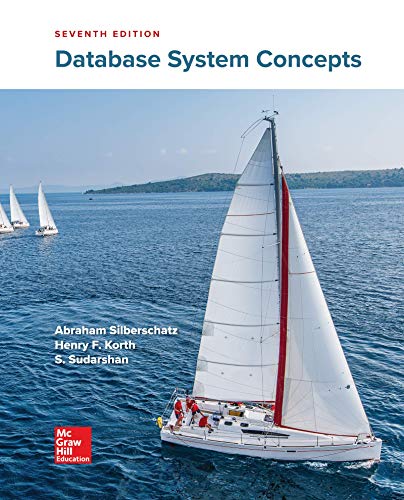
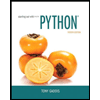
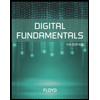
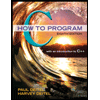
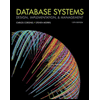
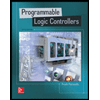