here is myLinReg needed to solve this problem function [a,E] = myLinReg(x,y) % [a,E] = myLinReg(x,y) % calculate the linear least squares regression to data given in x,y % Input % x: column vector of measured x data to fit % y: column vector of measured y data to fit % Output % a: vector of coefficients for the linear fit y = a(1)+a(2)*x % E: error of the fit = sum of the residual square % define a as a 2 entry vector a = zeros(2,1); n = length(x); % determine number of data points if n ~= length(y) fprintf ('Error: the length of data vectors x and y must be the same\n') a(:) = realmax(); E = realmax(); % set a and E to real max return end % calculate and store sum terms Sx = sum(x); Sy = sum(y); Sxx = sum(x.*x); Sxy = sum(x.*y); % Calculate linear equation coefficients a(1) = (Sxx*Sy-Sxy*Sx)/(n*Sxx-Sx*Sx); % a0 coefficient a(2) = (n*Sxy-Sx*Sy)/(n*Sxx-Sx*Sx); % a1 coefficient % Calculate the error of the fit E = sum((y-(a(2)*x+a(1))).^2); end
here is myLinReg needed to solve this problem function [a,E] = myLinReg(x,y) % [a,E] = myLinReg(x,y) % calculate the linear least squares regression to data given in x,y % Input % x: column vector of measured x data to fit % y: column vector of measured y data to fit % Output % a: vector of coefficients for the linear fit y = a(1)+a(2)*x % E: error of the fit = sum of the residual square % define a as a 2 entry vector a = zeros(2,1); n = length(x); % determine number of data points if n ~= length(y) fprintf ('Error: the length of data vectors x and y must be the same\n') a(:) = realmax(); E = realmax(); % set a and E to real max return end % calculate and store sum terms Sx = sum(x); Sy = sum(y); Sxx = sum(x.*x); Sxy = sum(x.*y); % Calculate linear equation coefficients a(1) = (Sxx*Sy-Sxy*Sx)/(n*Sxx-Sx*Sx); % a0 coefficient a(2) = (n*Sxy-Sx*Sy)/(n*Sxx-Sx*Sx); % a1 coefficient % Calculate the error of the fit E = sum((y-(a(2)*x+a(1))).^2); end
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
here is myLinReg needed to solve this problem
function [a,E] = myLinReg(x,y)
% [a,E] = myLinReg(x,y)
% calculate the linear least squares regression to data given in x,y
% Input
% x: column vector of measured x data to fit
% y: column vector of measured y data to fit
% Output
% a: vector of coefficients for the linear fit y = a(1)+a(2)*x
% E: error of the fit = sum of the residual square
% define a as a 2 entry vector
a = zeros(2,1);
n = length(x); % determine number of data points
if n ~= length(y)
fprintf ('Error: the length of data vectors x and y must be the same\n')
a(:) = realmax(); E = realmax(); % set a and E to real max
return
end
% calculate and store sum terms
Sx = sum(x); Sy = sum(y);
Sxx = sum(x.*x); Sxy = sum(x.*y);
% Calculate linear equation coefficients
a(1) = (Sxx*Sy-Sxy*Sx)/(n*Sxx-Sx*Sx); % a0 coefficient
a(2) = (n*Sxy-Sx*Sy)/(n*Sxx-Sx*Sx); % a1 coefficient
% Calculate the error of the fit
E = sum((y-(a(2)*x+a(1))).^2);
end
![**Developing a MATLAB Function for Least Squares Regression**
In this tutorial, you will learn how to develop a MATLAB function, `myFit`, designed to find the best fit for the function \( y(x) = \frac{1}{(mx^3 + b)} \) using a set of given data points \((x_i, y_i)\). This will involve applying least squares regression techniques.
**Function Requirements:**
- The function `myFit` should take two column vectors, `x` and `y`, as input arguments. These vectors represent the data points used in the fitting process.
- **Output:** The function should return two scalar values, `m` and `b`, which are the parameters for the function \( y(x) = \frac{1}{(mx^3 + b)} \).
**Procedure:**
1. **Set Up Regression:**
- To perform the linear least squares regression, utilize the function call:
```
[a, ~] = myLinReg(...)
```
- Inside your function, declare `a` as a global variable:
```
global a;
```
- This should be the first line in your `myFit` function to ensure proper usage of the global variable.
2. **Implementing myFit:**
- The function should correctly interact with the regression function `myLinReg`, performing operations to adjust the parameters `m` and `b` for the best fit.
By following these instructions, you will be able to create a MATLAB function that efficiently fits a curve to the given dataset using linear regression techniques. This skill is commonly applied in data analysis and computational modeling across various scientific and engineering fields.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffa3769d6-5bdb-4366-8adb-dd8310df7605%2F40f710ce-211b-49f4-a708-efa559f50b80%2Fcvlm9_processed.png&w=3840&q=75)
Transcribed Image Text:**Developing a MATLAB Function for Least Squares Regression**
In this tutorial, you will learn how to develop a MATLAB function, `myFit`, designed to find the best fit for the function \( y(x) = \frac{1}{(mx^3 + b)} \) using a set of given data points \((x_i, y_i)\). This will involve applying least squares regression techniques.
**Function Requirements:**
- The function `myFit` should take two column vectors, `x` and `y`, as input arguments. These vectors represent the data points used in the fitting process.
- **Output:** The function should return two scalar values, `m` and `b`, which are the parameters for the function \( y(x) = \frac{1}{(mx^3 + b)} \).
**Procedure:**
1. **Set Up Regression:**
- To perform the linear least squares regression, utilize the function call:
```
[a, ~] = myLinReg(...)
```
- Inside your function, declare `a` as a global variable:
```
global a;
```
- This should be the first line in your `myFit` function to ensure proper usage of the global variable.
2. **Implementing myFit:**
- The function should correctly interact with the regression function `myLinReg`, performing operations to adjust the parameters `m` and `b` for the best fit.
By following these instructions, you will be able to create a MATLAB function that efficiently fits a curve to the given dataset using linear regression techniques. This skill is commonly applied in data analysis and computational modeling across various scientific and engineering fields.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
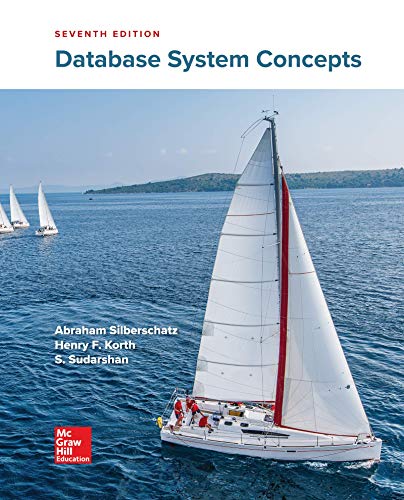
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
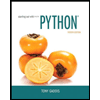
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
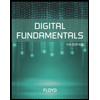
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
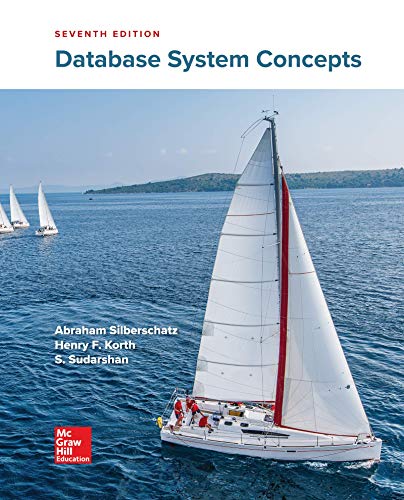
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
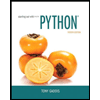
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
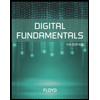
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
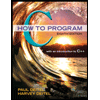
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
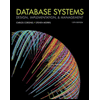
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
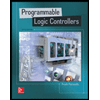
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education