Here is a problem that requires you to use the remainder operator to break a number into pieces and then add the pieces. Complete the standard Java console program named SumDigits.java.
Here is a problem that requires you to use the remainder operator to break a number into pieces and then add the pieces. Complete the standard Java console program named SumDigits.java.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Here is a problem that requires you to use the remainder operator to break a number into pieces and then add the pieces. Complete the standard Java console
The program reads a single integer from the user. (Do not read the input as a String.) You can assume that the user will cooperate and only input values between 0 and 1,000. Your program should use the arithmetic operators to sum all of the individual digits in the number.
![SumDigits.java
1 import java.util.Scanner;
2 /**
3
* Use calculations to process and integer digit.
* @author
4
* @version
6
*/
7 public class SumDigits
8 {
9
/**
10
* Read a number as an integer, then sum each individual digit.
* Do not read the number as a string.
11
12
*/
13
public static void main(String[] args)
{
System.out.println("Sum Digits");
System.out.println(":
// INPUT
// Dummy starter code. Replace with your IPO code.
System.out.print("Enter a number from 0 to 1000: 999");
System.out.println();
System.out.println("\tSum of the digits is: 27");
}
14
15
16
");
17
18
19
20
21
22
23 }](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F07c38dc6-4a9f-400d-9784-ec1cee64c4c5%2F9b682cc7-e0ab-464b-8d26-772c87e6a310%2Feyqfgjw_processed.png&w=3840&q=75)
Transcribed Image Text:SumDigits.java
1 import java.util.Scanner;
2 /**
3
* Use calculations to process and integer digit.
* @author
4
* @version
6
*/
7 public class SumDigits
8 {
9
/**
10
* Read a number as an integer, then sum each individual digit.
* Do not read the number as a string.
11
12
*/
13
public static void main(String[] args)
{
System.out.println("Sum Digits");
System.out.println(":
// INPUT
// Dummy starter code. Replace with your IPO code.
System.out.print("Enter a number from 0 to 1000: 999");
System.out.println();
System.out.println("\tSum of the digits is: 27");
}
14
15
16
");
17
18
19
20
21
22
23 }

Transcribed Image Text:Interactions Console Compiler Output
Gilbert, Stephen: Sum Digits
==
Enter a number from 0 to 1000: 999
Sum of the digits is: 27
To calculate the digits you'll have to do several divisions and integer remainder (%)
operations. Notice that for any integer, n % 10 returns the value of the right-most digit,
and n / 10 returns a new number with the right-most digit discarded.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
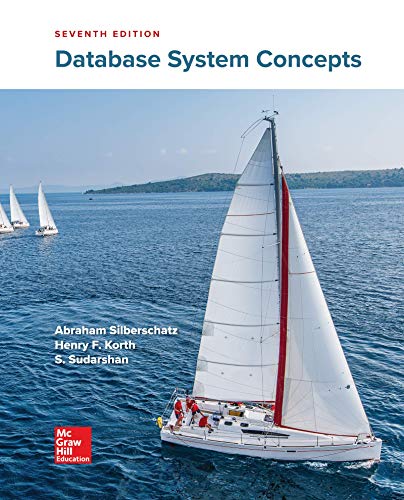
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
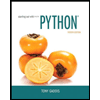
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
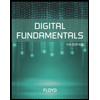
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
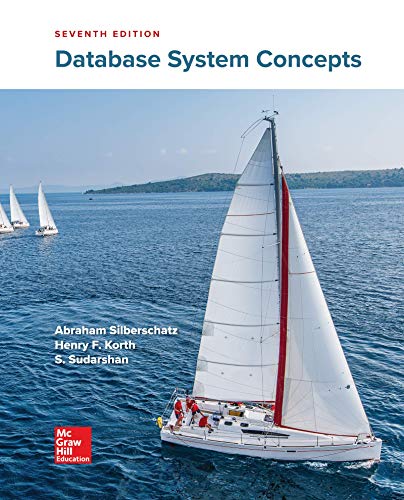
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
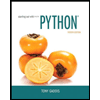
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
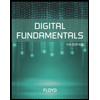
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
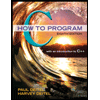
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
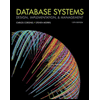
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
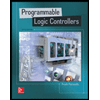
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education