help with java heap public class MinHeap implements Heap { privateint size =0; // number of elements currently in the heap privateint[] elts; // heap array privateint max; // array declared size // ================================================ // constructors // ================================================ publicMinHeap(intumax) { // user defined heap size this.max = umax; this.elts =newint[umax]; } publicMinHeap( ) { // default heap size is 100 this.max =100; this.elts =newint[100]; } //================================================== // methods we need to grade //================================================== publicint[] getArray() { // do not change this method return this.elts; } //========================================================= // public methods -- Implement these for the assignment. // Note that we want a Min Heap... so the operations // getFront and delFront and insert have to compare // for min being at the root //========================================================= publicvoidinsert(intp){ //Hint: remember to update size. Also, remember that we skip index 0 in the array. /*Your code here */
help with java heap public class MinHeap implements Heap { privateint size =0; // number of elements currently in the heap privateint[] elts; // heap array privateint max; // array declared size // ================================================ // constructors // ================================================ publicMinHeap(intumax) { // user defined heap size this.max = umax; this.elts =newint[umax]; } publicMinHeap( ) { // default heap size is 100 this.max =100; this.elts =newint[100]; } //================================================== // methods we need to grade //================================================== publicint[] getArray() { // do not change this method return this.elts; } //========================================================= // public methods -- Implement these for the assignment. // Note that we want a Min Heap... so the operations // getFront and delFront and insert have to compare // for min being at the root //========================================================= publicvoidinsert(intp){ //Hint: remember to update size. Also, remember that we skip index 0 in the array. /*Your code here */
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
help with java heap
public class MinHeap implements Heap { | |
privateint size =0; // number of elements currently in the heap | |
privateint[] elts; // heap array | |
privateint max; // array declared size | |
// ================================================ | |
// constructors | |
// ================================================ | |
publicMinHeap(intumax) { // user defined heap size | |
this.max = umax; | |
this.elts =newint[umax]; | |
} | |
publicMinHeap( ) { // default heap size is 100 | |
this.max =100; | |
this.elts =newint[100]; | |
} | |
//================================================== | |
// methods we need to grade | |
//================================================== | |
publicint[] getArray() { // do not change this method | |
return this.elts; | |
} | |
//========================================================= | |
// public methods -- Implement these for the assignment. | |
// Note that we want a Min Heap... so the operations | |
// getFront and delFront and insert have to compare | |
// for min being at the root | |
//========================================================= | |
publicvoidinsert(intp){ | |
//Hint: remember to update size. Also, remember that we skip index 0 in the array. | |
/*Your code here */ | |
} |

Transcribed Image Text:3
public interface Heap {
4
5
void insert(int p);
6.
// Insert adds the element p to the heap.
7
//
8
// In this heap the element is an int and IS the priority.
//
10
// We do not know at this abstract level if this will be
// a min-heap or a max-heap (or even something else like
// a fibonacci heap) and those details will be added in
// the class that implements this heap interface.
11
12
13
14
// Duplicate values are allowed.
15
//
// Error: The insert should fail if the heap is "full"
// meaning the array is maxed out and there is no room
16
17
// in the array for another element.
In this case
18
// simply return, and do nothing.
19
20
21
//Min-heap example:
// Suppose we have the following min-heap:
// 3, 5, 4, 12, 6, 9
22
23
24
25
// After inserting 2, the min-heap now looks as follows:
26
// 2, 5, 3, 12, 6, 9, 4
27
28
void delFront();
29
// Remove the "next" item from the heap, however "next"
30
// is defined... in this assignment our implementing class
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
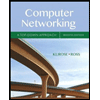
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
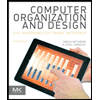
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
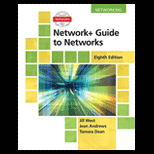
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
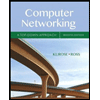
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
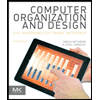
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
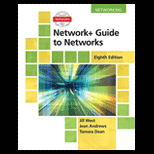
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
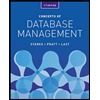
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
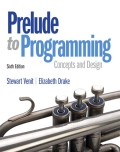
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
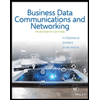
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY