Help with C++. Assign pointer engine1 with a new Engine object. Call engine1's Read() to read the object's data members from input. Then, call engine1's Print() to output the values of the data members. Finally, delete engine1. Ex: If the input is 1 20, then the output is: Engine's power: 1Engine's temperature: 20 ```#include "memtest.h"#include <iostream>using namespace std; class Engine { public: Engine(); void Read(); void Print(); private: int power; int temperature;}; Engine::Engine() { power = 0; temperature = 0;} void Engine::Read() { cin >> power; cin >> temperature;} void Engine::Print() { cout << "Engine's power: " << power << endl; cout << "Engine's temperature: " << temperature << endl;} int main() { Engine* engine1 = nullptr; /* Your code goes here */ memtest(); // Check memory for testing purposes only return 0;}```
Help with C++.
Assign pointer engine1 with a new Engine object. Call engine1's Read() to read the object's data members from input. Then, call engine1's Print() to output the values of the data members. Finally, delete engine1.
Ex: If the input is 1 20, then the output is:
Engine's power: 1
Engine's temperature: 20
```
#include "memtest.h"
#include <iostream>
using namespace std;
class Engine {
public:
Engine();
void Read();
void Print();
private:
int power;
int temperature;
};
Engine::Engine() {
power = 0;
temperature = 0;
}
void Engine::Read() {
cin >> power;
cin >> temperature;
}
void Engine::Print() {
cout << "Engine's power: " << power << endl;
cout << "Engine's temperature: " << temperature << endl;
}
int main() {
Engine* engine1 = nullptr;
/* Your code goes here */
memtest(); // Check memory for testing purposes only
return 0;
}
```
Unlock instant AI solutions
Tap the button
to generate a solution
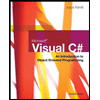
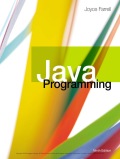
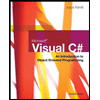
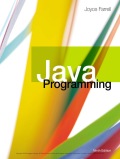
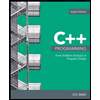
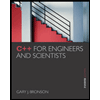
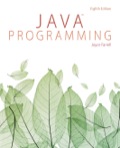