help creating a ULM Class Diagram, please. I have included my 3 classes of code below (UserInput, Participant, and Exchange Results). // Participant Class class Participant { // Participants private String name; private int age; public Participant(String name, int age) { this.name = name; this.age = age;
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I need help creating a ULM Class Diagram, please. I have included my 3 classes of code below (UserInput, Participant, and Exchange Results).
// Participant Class
class Participant
{
// Participants
private String name;
private int age;
public Participant(String name, int age)
{
this.name = name;
this.age = age;
}
// Name and Age Getters
public String getName()
{
return name;
}
public int getAge()
{
return age;
}
// Name and Age Setters
public void setAge(int age)
{
this.age = age;
}
public void setName(String name)
{
this.name = name;
}
}
import java.util.Random;
// ExchangeResults Class
public class ExchangeResults
{
public static void main(String[] args)
{
// Input Participants From The User
UserInput userinput = new UserInput();
Participant[] participants = userinput.inputParticipants();
// Shuffle The Array Of Participants
shufflepParticipants(participants);
// Print Gifts Exchanged
System.out.println(" Christmas Gift Exchange Results");
System.out.println("~ * ~ * ~ * ~ * ~ * ~ * ~ * ~ * ~");
for (int i = 0; i < participants.length / 2; i++)
{
Participant p1 = participants[i];
Participant p2 = participants[participants.length - i - 1];
System.out.printf("%s (age: %d) will echange a gift with %s (age: %d)\n",
p1.getName(), p1.getAge(), p2.getName(), p2.getAge());
}
}
// Shuffle Array Of Participants
public static void shufflepParticipants(Participant[] participants)
{
Random rand = new Random();
Participant temp;
// Shuffle Array
for (int i = participants.length - 1; i > 0; i--)
{
int index = rand.nextInt(i + 1);
temp = participants[index];
participants[index] = participants[i];
participants[i] = temp;
}
}
}
// UserInput Class
import java.util.Scanner;
public class UserInput {
Scanner input = new Scanner(System.in);
Participant[] inputParticipants()
{
System.out.println("* * * * CHRISTMAS GIFT EXCHANGE * * * *");
// Input Number Of Participants
System.out.print("How many people are participating in the exchange? ");
int numParticipants = Integer.parseInt(input.nextLine());
System.out.println();
// While Number Of Participants Is Odd,
// Keep Inputting Number Of Participants
while (numParticipants % 2 == 1)
{
System.out.println();
System.out.println("There must be an even number of people participating.");
System.out.println("(Add an 'ELF' if needed.)");
System.out.println();
System.out.print("How many people are participating in the exchange? ");
numParticipants = Integer.parseInt(input.nextLine());
System.out.println();
}
// Initialize Array Of Participants
Participant[] participants = new Participant[numParticipants];
// Input All Particicpants
for (int i = 0; i < participants.length; i++)
{
System.out.printf("Participant #%d", i + 1);
System.out.print("\n-------------------------\n");
// Input Name And Age
System.out.print("Name: ");
String name = input.nextLine();
System.out.print("Age: ");
int age = Integer.parseInt(input.nextLine());
participants[i] = new Participant(name, age);
System.out.println();
}
// Return Array Of Participants
return participants;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

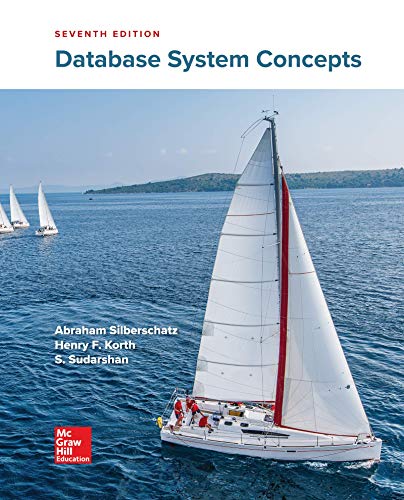
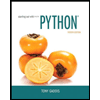
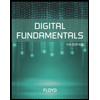
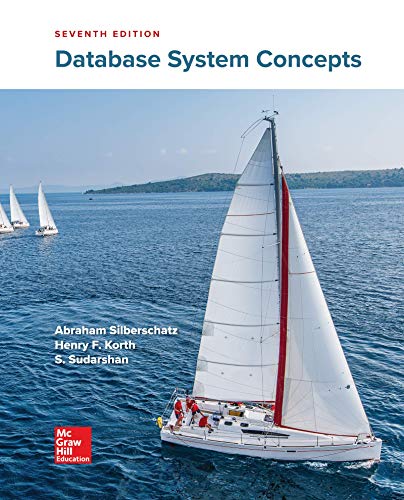
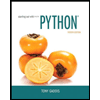
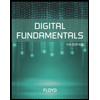
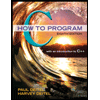
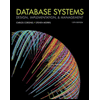
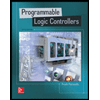