Hello. I can't get this Java code to work. It takes a series of sentences as a single string. The function capitalizes the first letter of every sentence What am I doing wrong? /* Programming Challenges Chapter 9 #3 Pg. 608 Title: Sentencee Capitallizer Write a method that accepts a String object as an argument and returns a copy of the string with the first character of each sentence capitalized. For instance, if the argument is “hello. my name is Joe. what is your name?” the method should return the string “Hello. My name is Joe. What is your name?” Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The modified string should be displayed on the screen. */ import javax.swing.JOptionPane; public class SentenceCapitalizer { public static void main(String[] args) { String input; input = JOptionPane.showInputDialog("Enter a string."); JOptionPane.showMessageDialog(null, sentenceCap(input)); System.exit(0); } public static String sentenceCap(String str) { int i; StringBuilder temp = new StringBuilder(str); if(temp.length() > 0) temp.setCharAt(0, Character.toUpperCase(temp.charAt(0))); i = temp.indexOf("."); while(i != -1) { i++; while(i < temp.length() && temp.charAt(i) == ' ') i++; temp.setCharAt(i, Character.toUpperCase(temp.charAt(i))); i = temp.indexOf(".", i); } return temp.toString(); } }
Hello. I can't get this Java code to work. It takes a series of sentences as a single string. The function capitalizes the first letter of every sentence What am I doing wrong?
/*
Programming Challenges
Chapter 9 #3 Pg. 608
Title: Sentencee Capitallizer
Write a method that accepts a String object as an argument and returns a copy of the string
with the first character of each sentence capitalized. For instance, if the argument is “hello.
my name is Joe. what is your name?” the method should return the string “Hello. My name
is Joe. What is your name?” Demonstrate the method in a program that asks the user to
input a string and then passes it to the method. The modified string should be displayed on
the screen.
*/
import javax.swing.JOptionPane;
public class SentenceCapitalizer
{
public static void main(String[] args)
{
String input;
input = JOptionPane.showInputDialog("Enter a string.");
JOptionPane.showMessageDialog(null, sentenceCap(input));
System.exit(0);
}
public static String sentenceCap(String str)
{
int i;
StringBuilder temp = new StringBuilder(str);
if(temp.length() > 0)
temp.setCharAt(0, Character.toUpperCase(temp.charAt(0)));
i = temp.indexOf(".");
while(i != -1)
{
i++;
while(i < temp.length() && temp.charAt(i) == ' ')
i++;
temp.setCharAt(i, Character.toUpperCase(temp.charAt(i)));
i = temp.indexOf(".", i);
}
return temp.toString();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

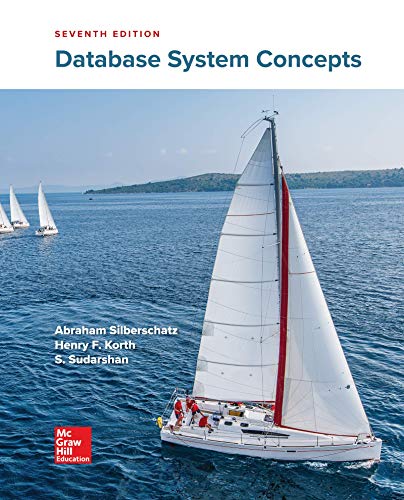
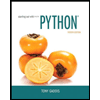
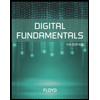
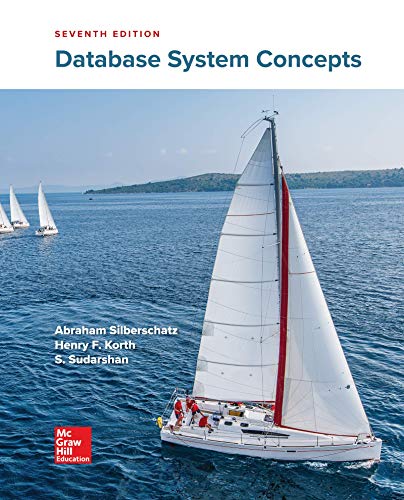
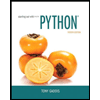
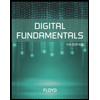
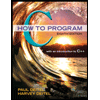
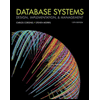
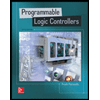