Hello im trying to make a list for my code but i cant use the library, how would i make a list for my linked list array based hashtable code
C++
Hello im trying to make a list for my code but i cant use the <list> library, how would i make a list for my linked list array based hashtable code
wordlist: incase you want to test the entire code
a
aaa
hello
goodbye
#pragma once
//hashtable.h
#include <iostream>
#include <string>
#include <list>
#include <cstdlib>
#include "word.h"
using namespace std;
class HashTable {
int BUCKET;
list<Word>* table;
public:
HashTable(int W);
//to check if the table is empty
bool isEmpty() const;
//to insert a key into the hashtable
void insertItem(string& x);
//to delete a key from the hashtable
void deleteItem(int key);
int hashFunction(Word x);
//to look for the key in the table
bool searchTable(string& key);
void displayHash();
};
//hashtable.cpp
#include "hashtable.h"
#include <iostream>
HashTable::HashTable(int W)
{
table = new list < Word >[W];
BUCKET = W;
return;
}
//to check if the table is empty
bool HashTable::isEmpty() const
{
for (int i = 0; i < BUCKET; i++)
{
if (!table[i].empty())
return false;
}
return true;
}
//to insert a key into the hashtable
void HashTable::insertItem(string& x)
{
int bucketIdx;
bucketIdx = hashFunction(x);
if (searchTable(x))
{
for (list < Word >::iterator i = table[bucketIdx].begin();
i != table[bucketIdx].end(); i++)
if (i->getWord().compare(x) == 0)
{
i->increment();
}
}
else
{
table[bucketIdx].push_back(x);
table[bucketIdx].sort();
}
}
//to delete a key from the hashtable
void HashTable::deleteItem(int key)
{
}
int HashTable::hashFunction(Word x)
{
int hashValue = 0;
for (char& c : x.getWord())
{
hashValue += c;
}
return (hashValue % BUCKET);
}
//to look for the key in the table
bool HashTable::searchTable(string& x)
{
int bucketIdx;
bucketIdx = hashFunction(x);
for (list < Word >::iterator i = table[bucketIdx].begin();
i != table[bucketIdx].end(); i++)
{
if (i->getWord().compare(x) == 0)
{
return true;
}
}
return false;
}
void HashTable::displayHash()
{
}
//word.h
#pragma once
#ifndef WORD_H_
#define WORD_H_
#include <string>
using namespace std;
class Word
{
private:
std::string word;
int occurrences;
public:
Word();
Word(std::string w); //word = w and occurrences = 1
std::string getWord() const; //return the stored word
int getOccurrences() const;
void increment(); //increment the occurrences value
bool operator <(const Word& w2) const //for sorting
{
return (word.compare(w2.word) < 0);
}
};
#endif
//word.cpp
#include <iostream>
#include <string>
#include "Word.h"
using namespace std;
//the default constructor
Word::Word() : word(""), occurrences(0)
{}
// the parameterized constructor
Word::Word(string w) : word(w), occurrences(1)
{}
// this return word
string Word::getWord() const
{
return word;
}
// this return occurrences
int Word::getOccurrences() const
{
return occurrences;
}
// increment occurrences
void Word::increment()
{
occurrences++;
}
//end of Word.cpp
//main.cpp
#include <iostream>
#include <iomanip>
#include <list>
#include <fstream>
//#include <bits/stdc++.h>
#include <chrono>
#include "word.h"
#include "hashtable.h"
using namespace std::chrono;
using namespace std;
int main() {
//inserting keys into the hashtable
HashTable ht(1000000);
//for the word lists
fstream wrdFile("wordlist.txt");
string word;
char chc ;
if (wrdFile.is_open()) {
while (wrdFile >> word) {
ht.insertItem(word);
}
}
else
{
cout << "file cannot be read" << endl;
}
wrdFile.close();
cout << "would you like to search for a word: " << endl
<< "please select your choice" << endl
<< "1.yes" << endl
<< "2.no" << endl;
cin >> chc;
if (chc == 1)
*/
//to search for the word in the hashtable
cout << "Give Word to Search : ";
while (cin >> word) {
//chrono to get back how long it took to search for the word
auto start = chrono::system_clock::now();
cout << " The Word " << word;
//searching for the word in the hashtable
if (!ht.searchTable(word))
cout << " was not";
cout << " was found in table" << endl << endl;
auto end = std::chrono::system_clock::now();
auto elapsed = std::chrono::duration_cast<std::chrono::microseconds>(end - start);
auto elapsed2 = std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
//printing out how long it took to find the word
cout << "How many microseconds it took to search for the word: " << elapsed.count() << " microseconds" << endl << endl;
cout << "How many milliseconds it took to search for the word: " << elapsed2.count() << " milliseconds" << endl << endl;
//asking user if they would like to search for a new word
cout << "would you like to look for a new word [y/n] : ";
cin >> chc;
cout << endl;
if (chc == 'y')
cout << "Give new word to Search: ";
else
return 0;
}
system("pause");
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

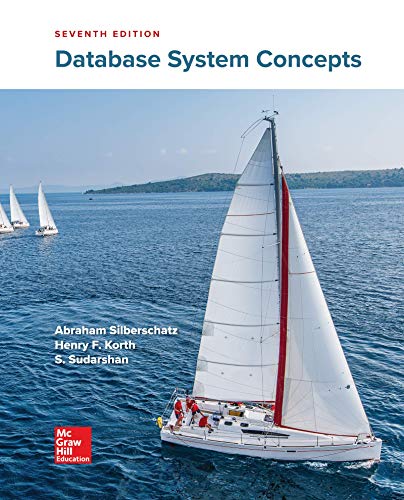
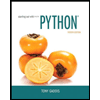
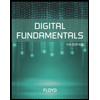
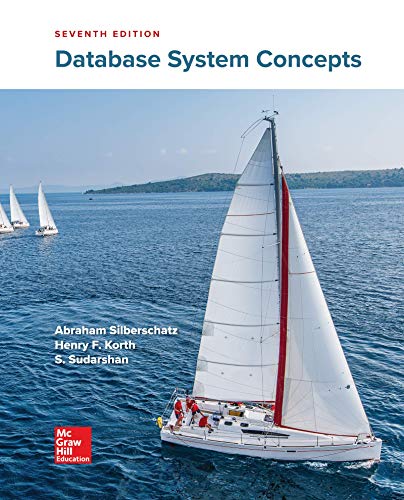
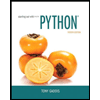
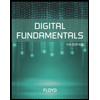
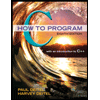
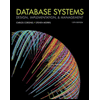
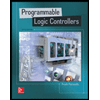