he algorithm uses the fact that the set to be permuted consists of distinct numbers. Thus, you cannot use the same algorithm to compute the permutations of the characters in a string. You can, however, use this technique to get all permutations of the character positions and then compute a string whose ith character is s[a[i]]. Use this approach to reimplement the generate_permutations function without recursion. c++
he algorithm uses the fact that the set to be permuted consists of distinct numbers. Thus, you cannot use the same algorithm to compute the permutations of the characters in a string. You can, however, use this technique to get all permutations of the character positions and then compute a string whose ith character is s[a[i]]. Use this approach to reimplement the generate_permutations function without recursion. c++
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
The following program generates all permutations of the numbers 0, 1, 2, ... , n – 1, without using recursion.
The
![```cpp
#include <iostream>
#include <vector>
using namespace std;
void swap(int& x, int& y)
{
int temp = x;
x = y;
y = temp;
}
void reverse(vector<int>& a, int i, int j)
{
while (i < j)
{
swap(a[i], a[j]); i++; j--;
}
}
bool next_permutation(vector<int>& a)
{
for (int i = a.size() - 1; i > 0; i--)
{
if (a[i - 1] < a[i])
{
int j = a.size() - 1;
while (a[i - 1] > a[j]) { j--; }
swap(a[i - 1], a[j]);
reverse(a, i, a.size() - 1);
return true;
}
}
return false;
}
void print(const vector<int>& a)
{
for (int i = 0; i < a.size(); i++)
{
cout << a[i] << " ";
}
cout << endl;
}
int main()
{
const int n = 4;
vector<int> a(n);
for (int i = 0; i < a.size(); i++) { a[i] = i; }
print(a);
while (next_permutation(a)) { print(a); }
return 0;
}
```
### Explanation:
This C++ program is designed to generate and print all permutations of a vector containing integers from 0 up to `n-1`. Here's a detailed breakdown of the program:
#### Functions:
- **swap(int& x, int& y):**
- Swaps the values of two integers, `x` and `y`.
- **reverse(vector<int>& a, int i, int j):**
- Reverses the portion of the vector `a` between indices `i` and `j`.
- **bool next_permutation(vector<int>& a):**
- Finds the next lexicographical permutation of the vector `a`.
- It returns `true` if the next permutation is found, otherwise returns `false`.
- **void print(const vector<int>& a):**
- Prints the elements of the vector `a` separated by spaces.
#### Main Function:
- Defines a constant `n` which is the size](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc0bffaab-5f14-4b72-8fd9-de0a2ce0a568%2F56f6929c-f587-49c7-b18d-beca31332648%2Fl0az93e_processed.png&w=3840&q=75)
Transcribed Image Text:```cpp
#include <iostream>
#include <vector>
using namespace std;
void swap(int& x, int& y)
{
int temp = x;
x = y;
y = temp;
}
void reverse(vector<int>& a, int i, int j)
{
while (i < j)
{
swap(a[i], a[j]); i++; j--;
}
}
bool next_permutation(vector<int>& a)
{
for (int i = a.size() - 1; i > 0; i--)
{
if (a[i - 1] < a[i])
{
int j = a.size() - 1;
while (a[i - 1] > a[j]) { j--; }
swap(a[i - 1], a[j]);
reverse(a, i, a.size() - 1);
return true;
}
}
return false;
}
void print(const vector<int>& a)
{
for (int i = 0; i < a.size(); i++)
{
cout << a[i] << " ";
}
cout << endl;
}
int main()
{
const int n = 4;
vector<int> a(n);
for (int i = 0; i < a.size(); i++) { a[i] = i; }
print(a);
while (next_permutation(a)) { print(a); }
return 0;
}
```
### Explanation:
This C++ program is designed to generate and print all permutations of a vector containing integers from 0 up to `n-1`. Here's a detailed breakdown of the program:
#### Functions:
- **swap(int& x, int& y):**
- Swaps the values of two integers, `x` and `y`.
- **reverse(vector<int>& a, int i, int j):**
- Reverses the portion of the vector `a` between indices `i` and `j`.
- **bool next_permutation(vector<int>& a):**
- Finds the next lexicographical permutation of the vector `a`.
- It returns `true` if the next permutation is found, otherwise returns `false`.
- **void print(const vector<int>& a):**
- Prints the elements of the vector `a` separated by spaces.
#### Main Function:
- Defines a constant `n` which is the size
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
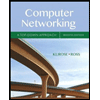
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
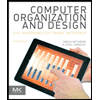
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
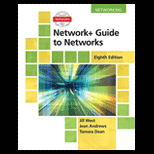
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
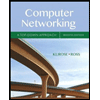
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
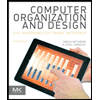
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
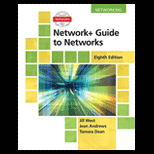
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
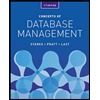
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
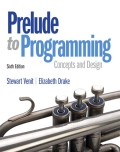
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
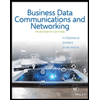
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY